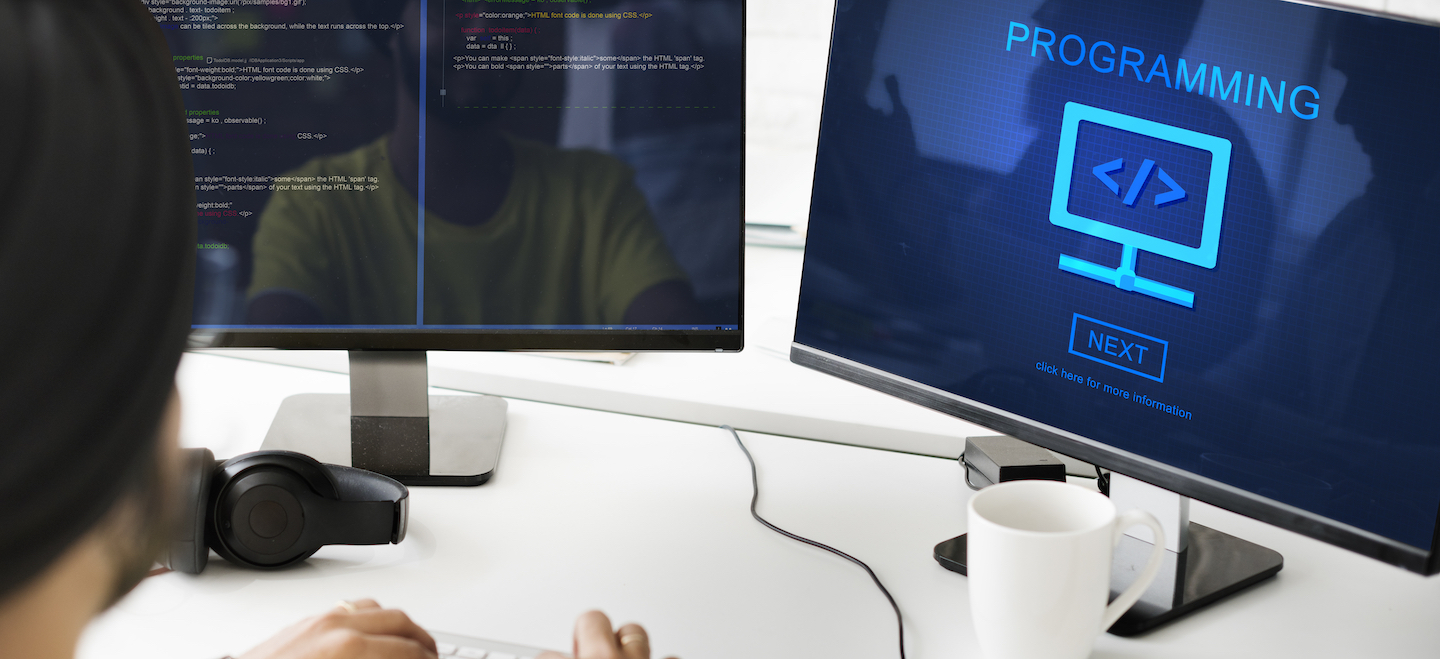
- 18th Dec 2023
- 15:44 pm
Java Regular Expressions, also known as Java Regex, are a powerful and versatile tool for pattern matching and text manipulation. Regular expressions are character sequences that define a search pattern. The 'java.util.regex' package in Java contains a set of classes and methods designed expressly for the smooth usage of regular expressions, allowing developers to harness their power in diverse text-processing contexts.
Primary Elements:
- Pattern Class:
The 'Pattern' class is the foundation of Java Regex. It represents a regex pattern that has been compiled. The 'Pattern.compile()' technique is used to construct patterns.
```
Pattern pattern = Pattern.compile("[a-zA-Z]+");
```
- Matcher Class:
The'Matcher' class is used to compare a pattern to a string. Calling the'matcher()' function on a 'Pattern' instance yields it.
```
Matcher matcher = pattern.matcher("HelloWorld");
```
- Syntax of Regex:
Java Regex employs a syntax akin to that of other programming languages. It introduces special characters such as '.' (representing any character), '*' (indicating zero or more occurrences), '+' (signifying one or more occurrences), '[]' (utilized for character classes), '()' (employed for grouping), and additional elements.
- Operations for Matching:
Key methods in the 'Matcher' class include'matches()', which checks if the entire string matches the pattern, 'find()', which finds the next match, and 'group()', which retrieves the substring that matches the pattern.
```
boolean matches = matcher.matches();
```
- Regex Flags:
Flags can be used with regex patterns to modify their behavior. For example, `Pattern.CASE_INSENSITIVE` makes the pattern case-insensitive.
```
Pattern pattern = Pattern.compile("java", Pattern.CASE_INSENSITIVE);
```
Java Regex is extensively employed for tasks such as data validation, text search and manipulation, and parsing within Java programs. Its indispensability arises from its expressive and concise syntax, making it an essential tool for dealing with string patterns effectively.
List of classes inside java.util.regex package
Here is a list of important classes inside the `java.util.regex` package along with their descriptions:
Class | Description |
‘Pattern’ | Represents a compiled regular expression pattern. |
‘Matcher’ | Performs match operations on a character sequence by interpreting a Pattern. |
‘MatchResult’ | A result object that contains information about the most recent match operation. |
‘PatternSyntaxException’ | Thrown when a syntax error is detected in the regular expression pattern. |
These classes interact to give a complete set of tools for working with regular expressions in Java.
- Pattern Type: The 'Pattern' class is where you start when dealing with regular expressions. It turns a regular expression pattern into a 'Pattern' object that may be used by the 'Matcher' class.
- Matcher Class: The 'Matcher' class is used to perform match operations on a character sequence using a compiled 'Pattern'. It has methods for conducting various matching operations and extracting matched substrings.
- MatchResult Interface: The 'MatchResult' interface represents the outcome of a match operation. It has methods for retrieving information about the most recent match, such as matched group start and end indices.
- PatternSyntaxException Class: The 'PatternSyntaxException' class is an unchecked exception that indicates a syntax mistake in a regular expression pattern. It contains important information regarding the error, such as the pattern, the error-index, and a thorough explanation of the detected error.
These classes enable developers to use regular expressions to perform complex string manipulations and pattern-matching operations in Java.
List of Java regex metacharacters along with their descriptions
. | Matches any character except a newline character. |
^ | Anchors the regex at the start of the string. |
$ | Anchors the regex at the end of the string. |
* | Matches 0 or more occurrences of the preceding character or group. |
+ | Matches 1 or more occurrences of the preceding character or group. |
? | Matches 0 or 1 occurrence of the preceding character or group. |
[] | Represents a character class, matches any single character within the brackets. |
[^] | Represents a negated character class, matches any single character not within the brackets. |
- | Specifies a range within a character class. |
() | Groups expressions together. |
\ | Escapes a metacharacter, allowing it to be treated as a literal character. |
` | ` |
{} | Specifies a specific number of occurrences. |
\d | Matches any digit (equivalent to [0-9]). |
\D | Matches any non-digit. |
\w | Matches any word character (alphanumeric plus underscore). |
\W | Matches any non-word character. |
\s | Matches any whitespace character. |
\S | Matches any non-whitespace character. |
(?i) | Enables case-insensitive matching. |
(?m) | Enables multiline mode, allowing ^ and $ to match the start/end of lines. |
(?s) | Enables dotall mode, allowing . to match newline characters. |
Used of Regex In Java
Regular expressions (regex) in Java are frequently utilised in a range of applications due to their comprehensive pattern-matching and modification capabilities. Here are some examples of common regex usage in Java:
- Validation of Data: Regex is frequently used to check user input and guarantee that it follows particular patterns. Validating email addresses, phone numbers, or zip codes, for example.
- Text Searching and Manipulation: A popular application is searching for certain patterns within text or changing text based on patterns. This entails detecting keywords dynamically, extracting information, and replacing text.
- Tokenization and Parsing: Long strings can be broken down into meaningful pieces using regex. When parsing and processing structured data such as log files, CSV files, or configuration files, this is critical.
- Extraction of Data: Regex can be used to extract specific data from text. This can be used to scrape websites, extract data from documents, and interpret log entries.
- Pattern Matching in Switch Statements (Java 12+): Java 12 introduced regex pattern support in switch statements. This simplifies complex pattern-based branching.
```
String input = "apple";
switch (input) {
case "apple" -> System.out.println("It's an apple!");
case "orange" -> System.out.println("It's an orange!");
// ...
}
```
- Validation of Password Strength: Regex is frequently used to ensure that passwords meet specific requirements (e.g., minimum length, use of uppercase, lowercase, digits, and special characters).
- Log File Analysis: Using regex in log file analysis is vital. Developers and system administrators use it to filter, search, and analyse log entries using predetermined patterns or criteria.
- URL Parsing: The use of regex simplifies processing and extracting components from URLs. When working with web-based apps or processing data from online sites, this comes in handy.
- NLP Tokenization: Regex is used in NLP activities to tokenize text, breaking it down into individual words or phrases for analysis.
Regex in Java is a vital tool for a wide range of applications in software development, data processing, and system administration because it provides a versatile and expressive solution to handle complex string-related tasks.
Java program that uses regex to validate email addresses
```
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.Scanner;
public class EmailValidator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Get email input from the user
System.out.print("Enter an email address: ");
String email = scanner.nextLine();
// Define the regex pattern for a basic email validation
String regexPattern = "^[a-zA-Z0-9_+&*-]+(?:\\.[a-zA-Z0-9_+&*-]+)*@(?:[a-zA-Z0-9-]+\\.)+[a-zA-Z]{2,7}$";
// Compile the regex pattern
Pattern pattern = Pattern.compile(regexPattern);
// Create a Matcher object
Matcher matcher = pattern.matcher(email);
// Check if the email matches the pattern
if (matcher.matches()) {
System.out.println("Valid email address!");
} else {
System.out.println("Invalid email address. Please enter a valid email.");
}
// Close the scanner
scanner.close();
}
}
```
Explanation:
- User Input: The programme use the 'Scanner' class to obtain an email address from the user.
- Regex Pattern: The regex pattern ('regexPattern') is used to validate simple email addresses. It examines an email address's general structure.
- Pattern Compilation: The pattern is compiled using the 'Pattern.compile()' function.
- Matcher Object: A 'Matcher' object is created by running the built pattern's matcher() function.
- Matching Check: The 'Matcher' class'fits()' method determines whether the supplied email matches the regex pattern.
- Results: The application indicates whether the entered email address is valid or not.
This application demonstrates how to use regex for email validation in Java. Based on the validation criteria, it may be customized for more complicated regex patterns.