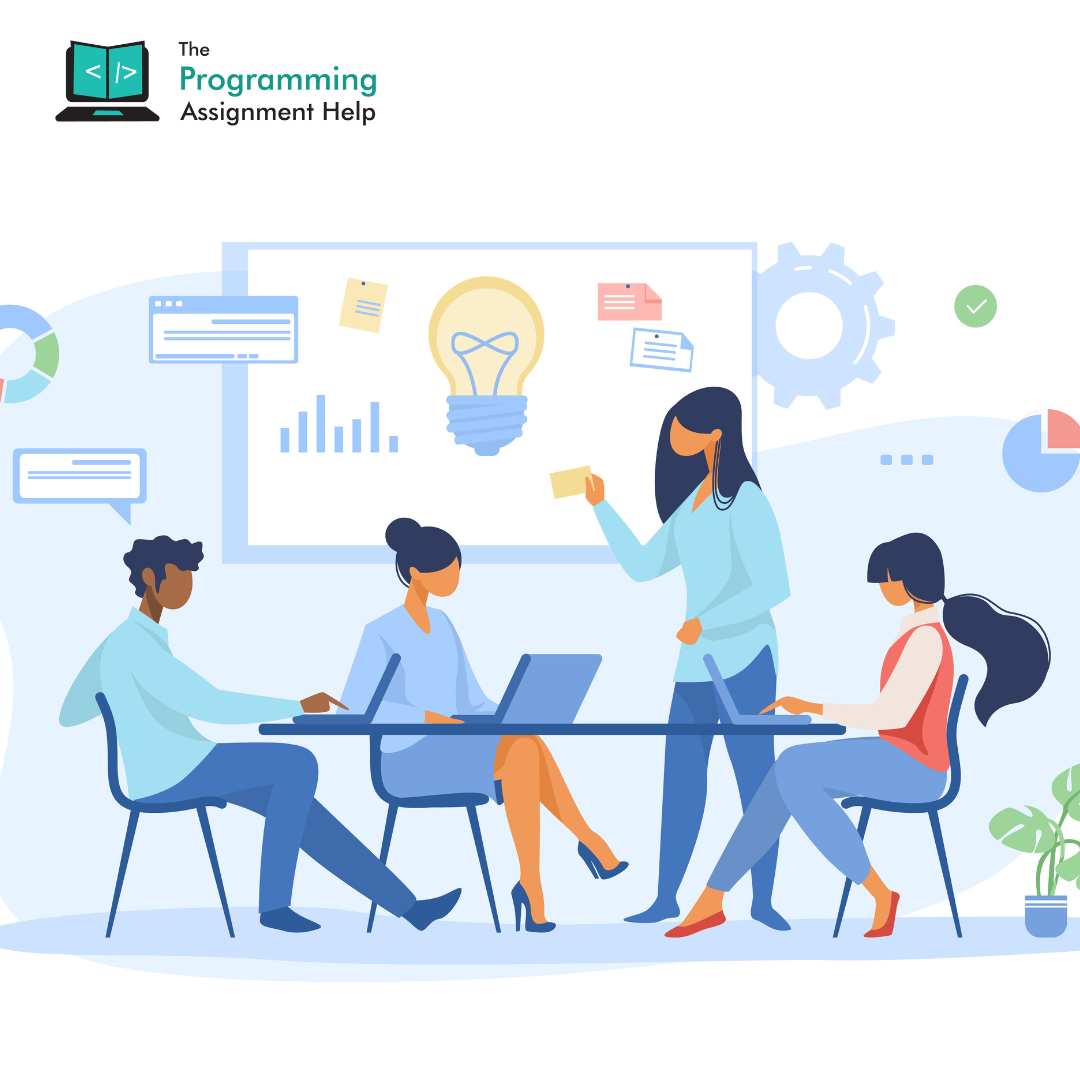
- 24th Nov 2022
- 03:40 am
- Admin
Below is a simple Java program for a School Management Application. This program allows users to perform basic school management operations like adding students, displaying student information, and calculating average grades.
import java.util.Scanner;
class Student {
String name;
int age;
double[] grades;
Student(String name, int age, double[] grades) {
this.name = name;
this.age = age;
this.grades = grades;
}
double getAverageGrade() {
double total = 0;
for (double grade : grades) {
total += grade;
}
return total / grades.length;
}
void displayInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
System.out.print("Grades: ");
for (double grade : grades) {
System.out.print(grade + " ");
}
System.out.println("\nAverage Grade: " + getAverageGrade());
System.out.println();
}
}
public class SchoolManagementApplication {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int capacity = 5; // Maximum number of students
Student[] students = new Student[capacity];
int count = 0; // Current number of students in the system
while (true) {
System.out.println("School Management Application");
System.out.println("1. Add Student");
System.out.println("2. Display All Students");
System.out.println("3. Exit");
System.out.print("Enter your choice: ");
int choice = sc.nextInt();
switch (choice) {
case 1:
if (count == capacity) {
System.out.println("Student database is full. Cannot add more students.");
} else {
sc.nextLine(); // Consume newline left by previous nextInt() call
System.out.print("Enter student name: ");
String name = sc.nextLine();
System.out.print("Enter student age: ");
int age = sc.nextInt();
System.out.print("Enter number of grades: ");
int numGrades = sc.nextInt();
double[] grades = new double[numGrades];
System.out.println("Enter grades (separated by spaces): ");
for (int i = 0; i < numGrades; i++) {
grades[i] = sc.nextDouble();
}
students[count] = new Student(name, age, grades);
count++;
}
break;
case 2:
if (count == 0) {
System.out.println("No students in the system.");
} else {
System.out.println("Student Information:");
for (int i = 0; i < count; i++) {
students[i].displayInfo();
}
}
break;
case 3:
System.out.println("Exiting School Management Application. Goodbye!");
sc.close();
System.exit(0);
break;
default:
System.out.println("Invalid choice. Please try again.");
break;
}
}
}
}
Note that this is a basic school management application with limited functionality. In a real-world scenario, a more robust and extensive application would be required to handle various operations and manage student information effectively.