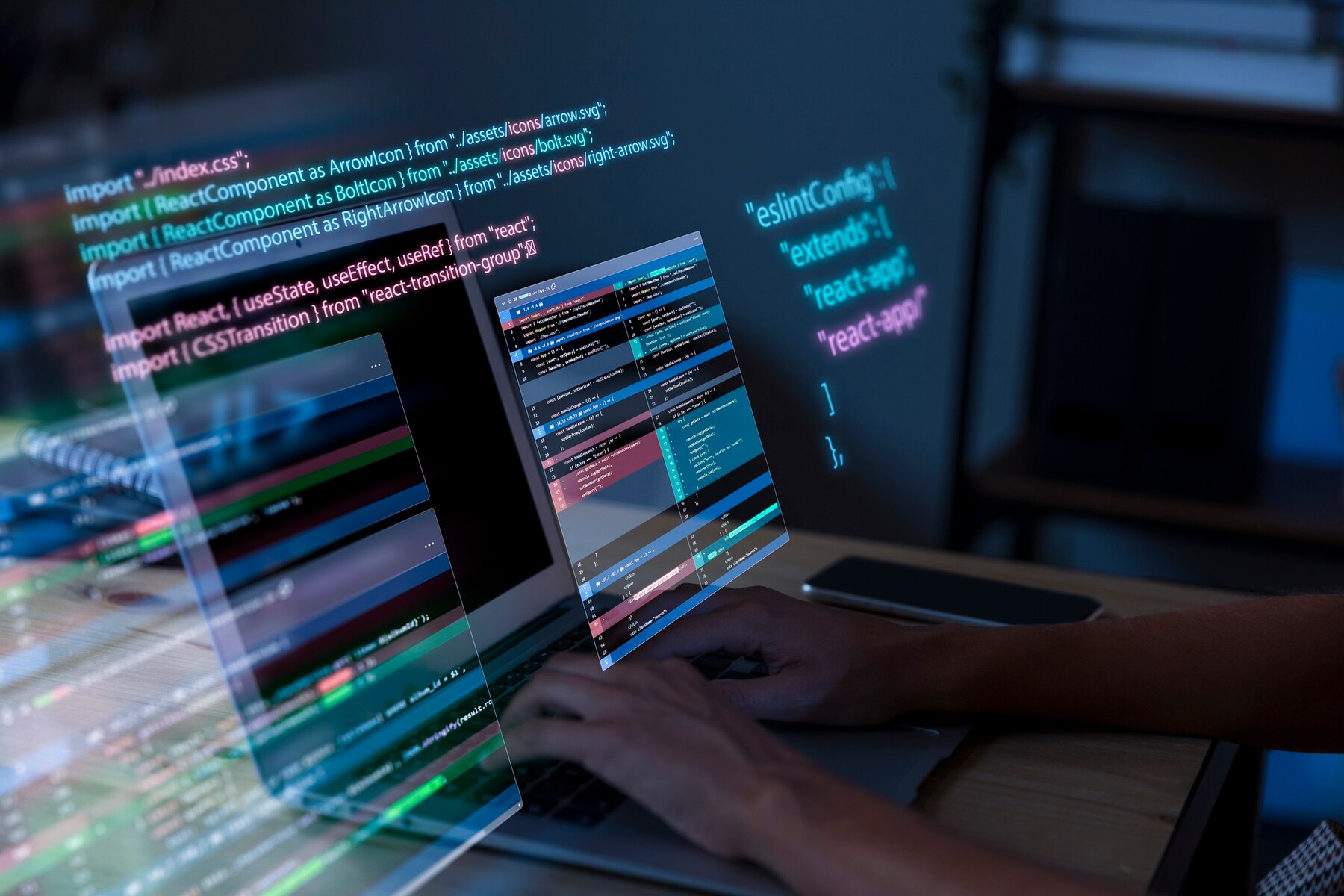
- 15th Jan 2024
- 17:29 pm
- Admin
One of our student's asked us a query on how he can write a code for Java GUI development using AWT. He wanted an example and our Java Tutors helped him understand the concept and solve 10-12 basic Java GUI problem. This problem written here is one of those. This example creates a basic GUI window with a button and a label. When the button is clicked, the label's text is updated.
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class SimpleAWTExample {
// Declare components
private Frame frame;
private Button button;
private Label label;
public SimpleAWTExample() {
// Initialize components
frame = new Frame("Java AWT Example");
button = new Button("Click Me");
label = new Label("Hello, World!");
// Set layout manager
frame.setLayout(new FlowLayout());
// Add components to the frame
frame.add(button);
frame.add(label);
// Add action listener to the button
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// Update label text when button is clicked
label.setText("Button Clicked!");
}
});
// Set frame properties
frame.setSize(300, 150);
frame.setVisible(true);
// Handle window closing event
frame.addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent windowEvent) {
System.exit(0);
}
});
}
public static void main(String[] args) {
new SimpleAWTExample();
}
}
This example creates a simple GUI window with a button and a label. When the button is clicked, the label's text is updated.