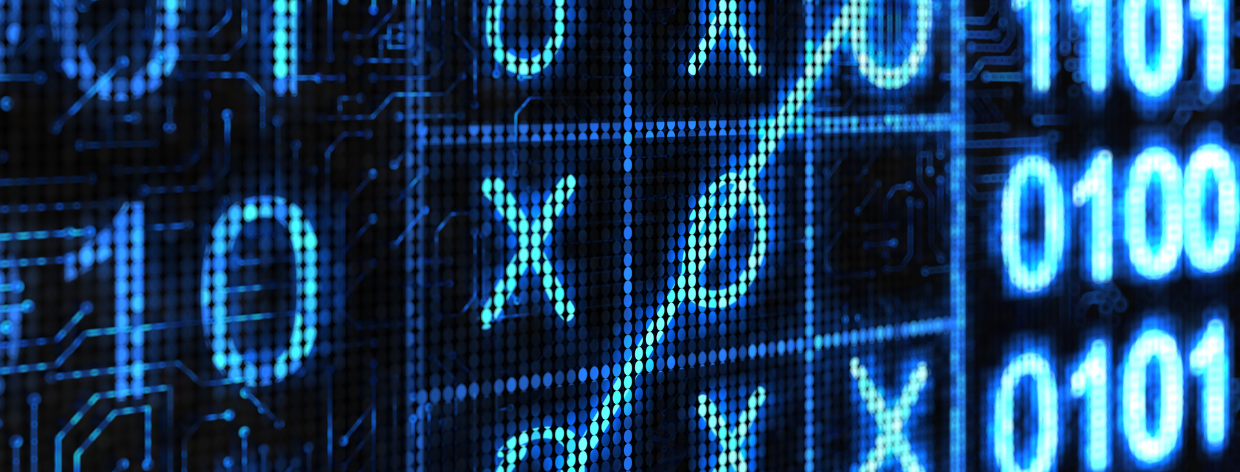
- 13th Feb 2024
- 21:24 pm
Java Gaming Project Question
The term project Tic-Tac-Toe will be Tic-Tac-Toe 2.0. Instead of 2 players, Tic-Tac-Toe 2.0 will allow from 3 to 10 players. Instead of a 3x3 board, the board size will be player count + 1 x player count + 1. For example if 6 players want to play, it’ll be a 7x7 board and if 4 players want to play it will be a 5x5 board. The rules and most of the game logic will be the same as the classical Tic-Tac-Toe. Each player will take turns and put their piece on the board. One twist though in the game logic will be how many pieces the winner needs to have in a row in order to win. It will be asked to the user to decide before the game begins to select the number of "in a row" pieces. Minimum can be 3 and maximum can be player count +1. For example, a game of four players can be chosen anywhere from 3 - 5 pieces in a row to define the winner. If the entire board is filled and there is no winner, it is a tie. Programming language is JAVA Automatic 0 if it is in another language Should be multiple classes/object oriented You cannot put everything in one class Automatic 0 if everything is in one class Make sure to comment your code Points will be taken off if comments are not provided Some Reminders Make sure to print the valid board after each move Print the valid board again after an invalid move so they can see the board Make sure to validate user’s moves Do not continue to next player until current player enters valid move Check to make sure the coordinates they provide is not occupied by another piece Make sure to check the coordinates they provide are valid board coordinates On a 4 x 4 board coordinates 6 2 is not valid Keep on asking to enter a valid a move Make sure to print the board in a tie or a win Announce the winner if there is one Make sure to ask for how many players? Go through each player and ask to enter their character Cannot be the same as another character.
Solution
Board.Java File
public class Board {
// data members
private int rows;
private int cols;
private char board[][];
private int linesToWin;
// parametrized constructor
public Board(int rows, int cols, int linesToWin)
{
this.rows = rows;
this.cols = cols;
this.linesToWin = linesToWin;
board = new char[rows][cols];
fillBoardByDefault();
}
private void fillBoardByDefault()
{
for(int i = 0;i <rows;i++)
{
for(int j =0;j< cols;j++)
{
board[i][j] = '-';
}
}
}
public void displayBoard()
{
for(int i = 0;i <rows;i++)
{
for(int j =0;j< cols;j++)
{
System.out.print(board[i][j]+" ");
}
System.out.println();
}
}
public boolean isDraw()
{
for (int i = 0;i <rows;i++)
{
for (int j =0;j< cols;j++)
{
if(board[i][j] == '-')
{
return false;
}
}
}
return true;
}
public boolean checkPlace(int row, int col, char character)
{
for (int i = 0;i <rows;i++)
{
for (int j =0;j< cols;j++)
{
if(board[i][j] == '-' && i == row && j == col)
{
board[i][j] = character;
return true;
}
}
}
return false;
}
public boolean ifPlayerWon(int row, int col, char character)
{
int count = 0;
int lastIndex;
//checking for down row
if(row + linesToWin - 1 < rows)
{
lastIndex = row + linesToWin -1;
for(int i = row; i <= lastIndex;i++)
{
if(board[i][col] == character)
{
count++;
}
else
{
break;
}
}
if(count == linesToWin)
{
return true;
}
}
count = 0;
//checking for up row
if(row - linesToWin + 1 >= 0)
{
lastIndex = row - linesToWin + 1;
for(int i = row ; i >= lastIndex;i--)
{
if(board[i][col] == character)
{
count++;
}
else
{
break;
}
}
if(count == linesToWin)
{
return true;
}
}
count = 0;
//checking for right column
if(col + linesToWin <= cols)
{
lastIndex = col + linesToWin -1;
for(int i = col; i <= lastIndex;i++)
{
if(board[row][i] == character)
{
count++;
}
}
if(count == linesToWin)
{
return true;
}
}
count = 0;
//checking for left col
if(col + 1 - linesToWin >= 0)
{
lastIndex = col + 1 - linesToWin;
for(int i = col; i >= lastIndex;i--)
{
if(board[row][i] == character)
{
count++;
}
else
{
break;
}
}
if(count == linesToWin)
{
return true;
}
}
count = 0;
// checking for left to right diagonal
for(int i =0;i< board.length;i++)
{
if(board[i][i] != character)
{
return false;
}
}
// checking for right to left diagonal
for(int i =0;i< board.length;i++)
{
if(board[i][board.length -1 - i] != character)
{
return false;
}
}
return true;
}
public boolean checkRow(int row)
{
if(row>=0 && row<rows)
{
return true;
}
return false;
}
public boolean checkCol(int col)
{
if(col >=0 && col<cols)
{
return true;
}
return false;
}
}
Driver
public class Driver {
public static void main(String[] args) {
Game obj = new Game();
obj.startGame();
}
}
Player
public class Player {
// data members
private String playerName;
private char playerChar;
// parametrized constructor
public Player(String playerName, char playerChar)
{
this.playerName = playerName;
this.playerChar = playerChar;
}
// mutator
public void setPlayerName(String playerName) {
this.playerName = playerName;
}
public void setPlayerChar(char playerChar) {
this.playerChar = playerChar;
}
// accessor
public char getPlayerChar() {
return playerChar;
}
public String getPlayerName() {
return playerName;
}
}
Game
import java.util.InputMismatchException;
import java.util.Scanner;
public class Game {
private Board board;
private Player players[];
public Game()
{
}
public void startGame()
{
int numPlayers = getNumberOfPlayer();
int numberOfLinesToWin = getLineToWin(numPlayers);
players = new Player[numPlayers];
defaultPlayers();
board = new Board(numPlayers+1, numPlayers+1, numberOfLinesToWin);
fillPlayerInfo();
playGame();
}
private void defaultPlayers()
{
for(int i = 0;i<players.length;i++)
{
players[i] = null;
}
}
private void fillPlayerInfo()
{
Scanner scanner = new Scanner(System.in);
String name;
char c;
for(int i = 0;i<players.length;i++)
{
System.out.println("Enter "+(i+1)+" player information");
System.out.print("Enter name: ");
name = scanner.nextLine();
c = getPlayerChar();
Player player = new Player(name, c);
players[i] = player;
}
}
private char getPlayerChar()
{
char c;
Scanner scanner = new Scanner(System.in);
while(true)
{
System.out.print("Enter char: ");
c = scanner.nextLine().charAt(0);
if(ifCharUnique(c))
{
return c;
}
System.out.println("Character already taken by another player");
}
}
private boolean ifCharUnique(char c)
{
for(int i =0;i<players.length;i++)
{
if(players[i] != null)
{
if(players[i].getPlayerChar() == c)
{
return false;
}
}
}
return true;
}
private int getNumberOfPlayer()
{
int num;
Scanner scanner = new Scanner(System.in);
while(true)
{
try
{
System.out.print("Enter number of players: ");
num = scanner.nextInt();
if(num >= 3 && num <=10)
{
return num;
}
System.out.println("Invalid numbers of players it can be between 3-10 inclusive");
}
catch (InputMismatchException e)
{
System.out.println("Please enter integer value");
}
}
}
private int getLineToWin(int numberOfPlayer)
{
int num;
Scanner scanner = new Scanner(System.in);
while(true)
{
try
{
System.out.print("Enter number of pieces player needs to win: ");
num = scanner.nextInt();
if(num >= 3 && num <=numberOfPlayer + 1)
{
return num;
}
System.out.println("Invalid numbers of players it can be between 3 and "+(numberOfPlayer+1)+" inclusive");
}
catch (InputMismatchException e)
{
System.out.println("Please enter integer value");
}
}
}
private void playGame()
{
System.out.println("Starting Game");
board.displayBoard();
System.out.println();
boolean checkMove;
boolean loop = true;
int row = 0;
int col = 0;
while(loop)
{
for(int i = 0;i<players.length;i++)
{
System.out.println("Player "+players[i].getPlayerName()+" turn");
while(true)
{
row = getPlayerRow();
col = getPlayerCol();
checkMove = board.checkPlace(row, col, players[i].getPlayerChar());
if(checkMove)
{
break;
}
else
{
System.out.println("Place have already been occupied");
}
}
board.displayBoard();
if(board.ifPlayerWon(row, col, players[i].getPlayerChar()))
{
System.out.println("Game is won by player "+players[i].getPlayerName());
loop = false;
break;
}
if(board.isDraw())
{
System.out.println("Game is Drawn");
loop = false;
break;
}
System.out.println();
}
}
}
private int getPlayerRow(){
int row;
boolean checkValid;
Scanner scanner = new Scanner(System.in);
while(true)
{
System.out.print("Enter row: ");
row = scanner.nextInt();
checkValid = board.checkRow(row);
if(checkValid)
{
return row;
}
else
{
System.out.println("Invalid row");
}
}
}
private int getPlayerCol(){
int col;
boolean checkValid;
Scanner scanner = new Scanner(System.in);
while(true)
{
System.out.print("Enter col: ");
col = scanner.nextInt();
checkValid = board.checkCol(col);
if(checkValid)
{
return col;
}
else
{
System.out.println("Invalid col");
}
}
}
}