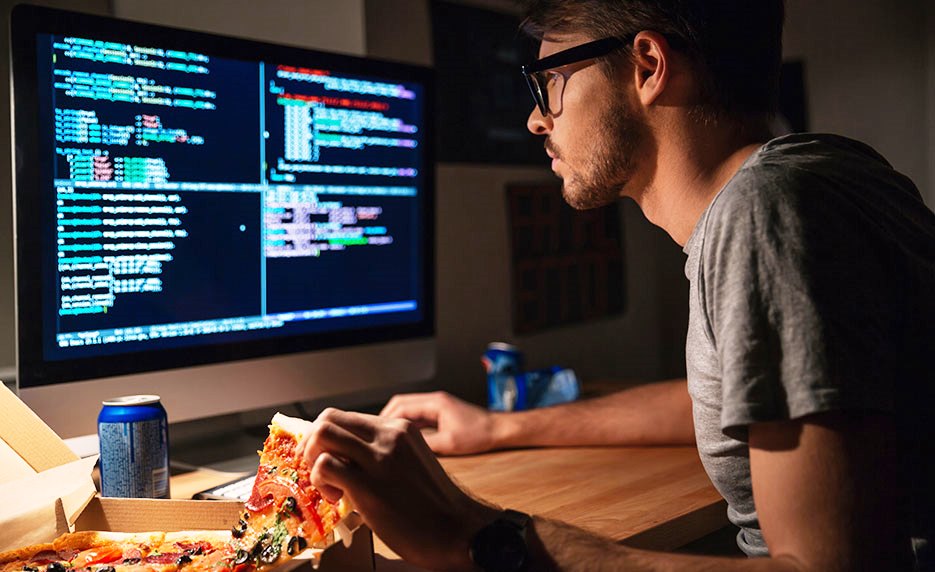
- 8th Sep 2019
- 03:33 am
- Ali Akavis
Java Assignment Question
Java Assignment – Implement An Operation Using Online IDE (coding) Platform
- Go to the online IDE to implement the following.
- Read a message from a file or stdin (the online IDE should support this).
- Use the key "S3cR3TP@55w0rD", and a random IV in CBC mode.
- Encrypt the text using any encryption function supported in the library. Decrypt the output from step 6
- Your program should output both the encrypted message (ciphertext) and the decrypted ciphertext.
Java Assignment Help - Solution
from Crypto.Cipher import AES import random print ("enter a message to encrypt") message = raw_input().encode('utf-8') #take input as a message from user for encryption key = '"S3cR3TP@55w0rD"' #random value as a key and must be used same key for encryption and decryption both iv = ''.join([chr(random.randint(0, 0xFF)) for i in range(16)]) #random iv value message += ' ' * (16 - len(message) % 16) #padded message in 16 bytes format cipher_encrypt = AES.new(key,AES.MODE_CBC,IV = iv) # encryption of IV using AES cipher_text = cipher_encrypt.encrypt(message) #encrypt user entered message print ("ciphertext value : " + cipher_text) #print "IV value : " + iv cipher_decrypt = AES.new(key,AES.MODE_CBC,IV = iv) #decryot user entered message print ("Decrypted Message : " + cipher_decrypt.decrypt(cipher_text))
Java Code Output
Enter a message to encrypt hi how are you hero ciphertext value : /??F0?%?????l#?f????@q?-V???r Decrypted Message : hi how are you hero Process finished with exit code 0