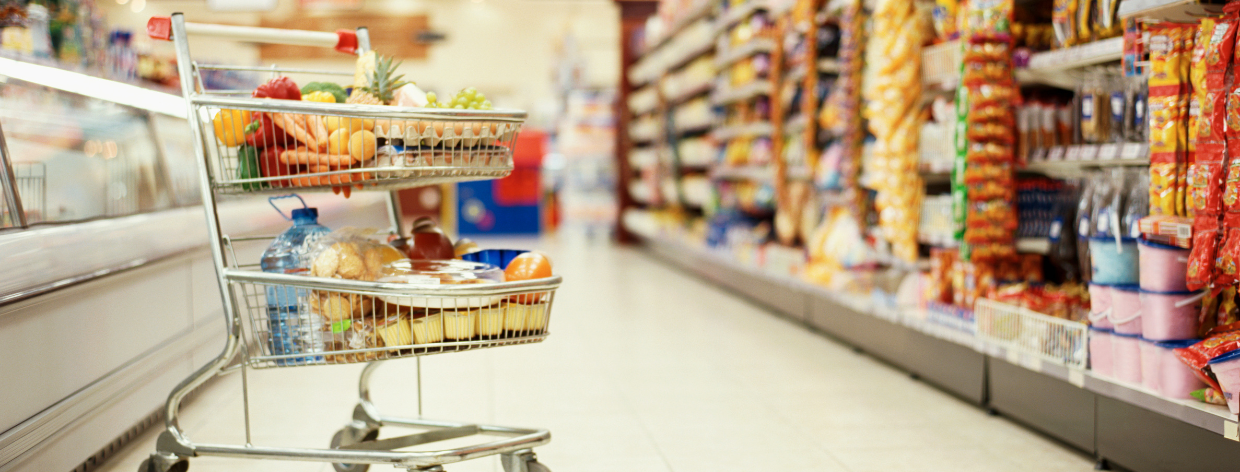
- 13th Feb 2024
- 22:00 pm
- Ali Akavis
Java Assignment Question
The management of a certain chain of supermarkets would like to know on a comparative basis the performance of its supermarkets in each city. The data in the following table shows the amount of profit for all the supermarkets in each city.
City Profit
Miami $10,200,000.00
Sunrise $14,600,000.00
Hollywood $17,000,000.00
Tallahassee $$6,000,000.00
Jacksonville $21,600,000.00
Orlando $9,100,000.00
Gainesville $8,000,000.00
Pensacola $12,500,000.00
Ocala $2,000,000.00
Sebring $4,500,000.00
The information required must be presented in the following order:
- Display the original data set, as shown in the table.
- The average profit for the supermarket chain.
- The city with the highest profit.
- A list of all the cities with profit at, or above the average.
- The standard deviation of the profits.
- The cities and their profit listed in descending order of the profits.
- Find the profit for any given city.
To carry out this exercise:
- Design a class called Supermarkets that accepts two arrays - one containing the names of the cities, and the other containing the profits. Write separate methods for each of the seven (7) activities stated in the list of requirements.
- Design a test class called TestSupermarket that:
i. Reads the data using the class GetData
ii. Creates a Supermarket object using the arrays as arguments to its constructor.
iii. Call the respective methods to carry out the requirements.
Using the example discussed in class, note the following:
The standard deviation is the square root of the sum of the squared difference of
an observation and the mean, divided by the number of observations.
That is,
-
s = Ö(S(xi – ? )2/N), i ? [0, MAX]
-
The expression S(xi - ?)2 can be expressed as follows:
-
sumDifference = sumDifference + Math.pow((rainfall [i] - mean), 2);
-
where:
-
(xi - ?)2 is Math.pow((rainfall [i] - mean), 2);
-
xi - is rainfall [i]
-
? - is the mean (average) that was already calculated, and
-
MAX - is rainfall.length – 1
// The following may help you.
double standardDeviation()
{
double sumDifference = 0;
double mean = average();
for (int i = 0; i < rainfall.length; i++)
sumDifference = sumDifference + Math.pow((rainfall [i] - mean), 2);
return Math.sqrt(sumDifference/(rainfall.length – 1));
}
Java Assignment Solution
Get Data
public class GetData {
//function to get the city name
public String[] getCity() {
String cityName[] = {"Miami", "Sunrise", "Hollywood", "Tallahassee", "Jacksonville", "Orlando", "Gainesville", "Pensacola", "Ocala", "Sebring"};
return cityName;
}
//function to get the profit
public double[] getProfit() {
double profit[] = {10200000.00, 14600000.00, 17000000.00, 6000000.00, 21600000.00, 9100000.00, 8000000.00, 12500000.00, 2000000.00, 4500000.00};
return profit;
}
}
Supermarkets
import java.text.DecimalFormat;
public class Supermarkets {
//define all the private variable
private String cityName[];
private double profit[];
//Parameterised constructor
public Supermarkets(String[] cityName, double[] profit) {
this.cityName = cityName;
this.profit = profit;
}
//method for the first task
public void display() {
System.out.printf("%-20s%-15s\n", "City", "Profit");
//use decimal format to print comma in thousand
DecimalFormat decimalFormat = new DecimalFormat("#.00");
decimalFormat.setGroupingUsed(true);
decimalFormat.setGroupingSize(3);
//iterate over the array and print it details
for (int i = 0; i < this.cityName.length; i++) {
System.out.printf("%-20s$%-15s\n", this.cityName[i], decimalFormat.format(this.profit[i]));
}
}
//method for the second task
public double average() {
double avg = 0;
double sum = 0;
//iterate over the array sum the profit
for (int i = 0; i < this.profit.length; i++) {
sum += this.profit[i];
}
//calculate average
avg = sum / this.profit.length;
return avg;
}
//method for the third task
public String cityWithHighestProfit() {
int index = 0;//assume first city has highest proft
//iterate over the array
for (int i = 0; i < this.profit.length; i++) {
if (this.profit[i] > this.profit[index]) {
index = i;
}
}
return this.cityName[index];
}
//method for the fourth task
public void cityList() {
boolean flag = true;
//iterate over the array
for (int i = 0; i < this.profit.length; i++) {
if (average() <= this.profit[i]) {
System.out.println(this.cityName[i]);
flag = false;
}
}
if (flag == true) {
System.out.println("No City Found");
}
}
//method for the fifth task
public double standardDeviation() {
double sumDifference = 0;
double mean = average();
for (int i = 0; i < this.profit.length;
i++) {
sumDifference = sumDifference + Math.pow((this.profit[i] - mean), 2);
}
return Math.sqrt(sumDifference / (this.profit.length - 1));
}
//method for the sixth task
public void displayDescending() {
//perform the bubble sort for sorting
for (int i = 0; i < this.profit.length; i++) {
for (int j = 1; j < (this.profit.length - i); j++) {
if (this.profit[j - 1] < this.profit[j]) {
//swap elements
double tempDouble = this.profit[j - 1];
this.profit[j - 1] = this.profit[j];
this.profit[j] = tempDouble;
String tempString = this.cityName[j - 1];
this.cityName[j - 1] = this.cityName[j];
this.cityName[j] = tempString;
}
}
}
display();
}
//method for the seventh task
public void find(String city) {
DecimalFormat decimalFormat = new DecimalFormat("#.00");
decimalFormat.setGroupingUsed(true);
decimalFormat.setGroupingSize(3);
int index = -1;
for (int i = 0; i < this.profit.length; i++) {
if(this.cityName[i].compareTo(city)==0)
{
index =i;
break;
}
}
if(index ==-1)
System.out.println("City not found in list");
else
System.out.printf("Profit for city %s is %s\n",this.cityName[index],decimalFormat.format(this.profit[index]));
}
}
Test Supermarket
import java.text.DecimalFormat;
public class TestSupermarket {
public static void main(String args[]) {
//create getdata object to get the data
GetData data = new GetData();
//create supermarket object
Supermarkets supermarket = new Supermarkets(data.getCity(), data.getProfit());
//create decimal format object to print comma in thousand place
DecimalFormat decimalFormat = new DecimalFormat("#.00");
decimalFormat.setGroupingUsed(true);
decimalFormat.setGroupingSize(3);
//call the first method
System.out.println("1. Display the original data set, as shown in the table.");
supermarket.display();
System.out.println();
//call the second method
System.out.println("2. The average profit for the supermarket chain.");
System.out.printf("Average Profit is : %s\n", decimalFormat.format(supermarket.average()));
System.out.println();
//call the third method
System.out.println("3. The city with the highest profit.");
System.out.println("City with highest Profit is : " + supermarket.cityWithHighestProfit());
System.out.println();
//call the fourth method
System.out.println("4. A list of all the cities with profit at, or above the average.");
supermarket.cityList();
System.out.println();
//call the fifth method
System.out.println("5. The standard deviation of the profits.");
System.out.printf("Standard deviation is : %s\n", decimalFormat.format(supermarket.standardDeviation()));
System.out.println();
//call the sixth method
System.out.println("6. The cities and their profit listed in descending order of the profits.");
supermarket.displayDescending();
System.out.println();
//call the seventh method
System.out.println("7. Find the profit for any given city.");
supermarket.find("Gainesville");
System.out.println();
}
}