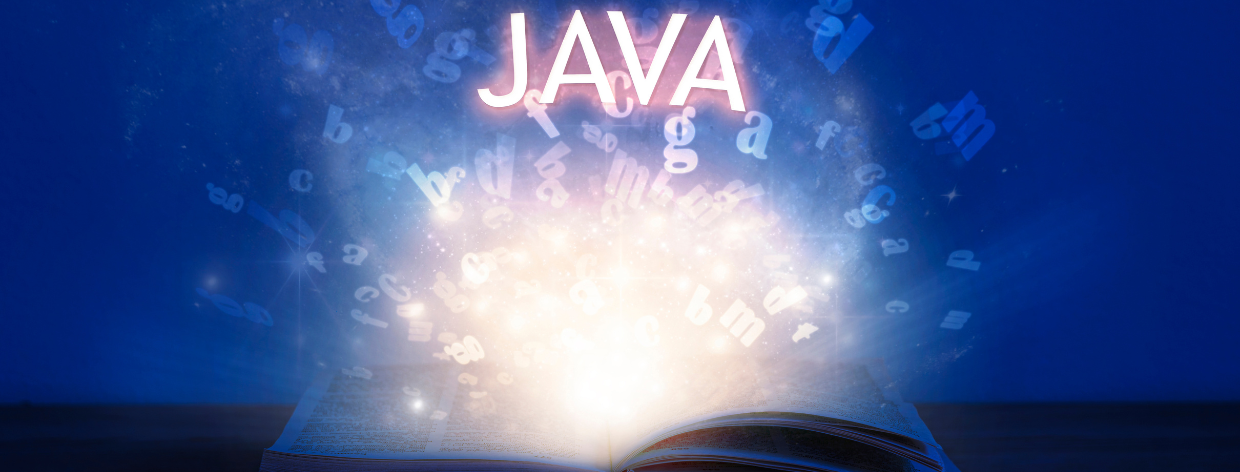
- 7th Feb 2024
- 14:44 pm
- Admin
Question
In less time than expected, you craft a Dice class as instructed! As if by magic, the Wanderer appears in a flash of lightning and thunder! "Well done," the Wanderer says, in that same metallic-sounding voice. "I challenge you to a game! A game of mice and souls! If you win, I will help you leave this place. If you lose, you can try again using your newfound knowledge to do better. You will lose! Mreowhahahahaha!" Reaching both hands up to its face, it starts undoing the clasps to its helmet. With trepidation, you await seeing what the Wanderer really looks like. With agonizing slowness, the helmet is lifted off the Wander's head, revealing...a cat, a giant cat, and still a cat. This explains a lot of things.
ITEC 120 Principles - Dice Souls (This just got dark...) - Assignment Solution
Dice Souls
/*
* ITEC 120 - Assignment 9
*/
/**
* This class simulates playing the Dice Souls dice game. The Soul Reaver
* reaves souls, day-in and day-out, until it dies and becomes a soul to be
* reaved by another reaver. This is really pretty dark stuff.
*
* @author Jeffrey LaMarche
*
* @version 1.0 2022-04-10
* Initial Template Version
*
* @author TODO Add Your Name Here (Remove the TODO text too)
*
* @version TODO Add Your Version Here (Remove the TODO text too)
*/
public class DiceSouls // yeah, this just got dark...
{
/**
* The main method for the Dice Souls game.
*
* @param args a reference to a String array for the command line arguments
*/
public static void main(String[] args)
{
int count = 0;
if(args.length > 1)
{
if(isStringPositiveInteger(args[0]))
{
count = Integer.parseInt(args[0]);
}
}
if(count == 0)
{
count = 8;
}
Dice [] dice = createPlayerDice(count);
int totalRound = 0;
int totalSoul =0;
int soul = 0;
do
{
rollAllDice(dice);
totalRound++;
System.out.println("Round: "+totalRound);
System.out.println("--- Soul Reaver's Roll ---");
displayDice(dice);
System.out.println("--------------------------");
soul = collectedSouls(dice);
totalSoul = totalSoul + soul;
System.out.println("Souls Reaved: "+soul);
System.out.println("Total Souls Reaved: "+totalSoul);
System.out.println();
}while(playerSurvives(dice));
displayGameOver(totalRound, totalSoul);
}
// ---- PROVIDED METHODS BELOW ---- //
/**
* Creates an array of Dice objects, with random sides for each dice
* selected from 4, 6, 8, 10, 12, or 20 sides.
*
* @param numberDice the number of Dice objects that the array can hold
*
* @return an array of Dice objects with length numberDice
*/
public static Dice[] createPlayerDice(int numberDice)
{
Dice[] playerDice;
/*
* If the number of dice is not positive, setup an array with no
* elements that will be returned at the end.
*/
if( numberDice < 0 )
{
playerDice = new Dice[0];
}
else
{
/*
* Represents the valid number of sides for a Dice object. The first
* element is junk and never used. This makes mapping simpler.
*/
final int[] SIDE_VALUES = { -1, 4, 6, 8, 10, 12, 20 };
Dice d6 = new Dice();
playerDice = new Dice[numberDice];
int sides = 0;
boolean addSuccess = false;
/*
* Hopefully the Dice validity test is correct.
*/
if( d6.isValidDice() )
{
/*
* Iterate through the array of Dice, creating a new Dice object
* for each element, having a random number of valid sides.
*/
for( int index = 0; index < playerDice.length; index++ )
{
// Randomly select a value between 1 and 6 inclusive.
d6.rollDice();
// Get the value of the Dice object.
int diceValue = d6.getValue();
// Obtain the number of sides that the 1 to 6 maps to.
sides = SIDE_VALUES[diceValue];
// Add the new Dice object to the array of Dice.
addSuccess = addDie(playerDice, new Dice(sides), index);
/*
* If the Dice object was not added successfully,
* stop execution.
*/
if( !addSuccess )
{
System.err.println("ERROR: ");
System.err.println("addDie did not work correctly!");
System.err.println(" Program terminating...");
System.exit(1);
}
}
}
else
{
System.err.println("ERROR: Dice is not valid!");
System.err.println("Program terminating...");
System.exit(1);
}
}
return playerDice;
}
/**
* Determines if a string is a positive integer value.
*
* @return true if the string is an integer greater or equal to zero,
* false otherwise
*/
public static boolean isStringPositiveInteger(String str)
{
boolean isNumber = false;
/*
* A null or empty string cannot be positive.
*/
if( str != null && str.length() > 0 )
{
isNumber = true;
/*
* Get each character out of a character array.
*/
for( char ch : str.toCharArray() )
{
/*
* If the character is not a digit (i.e., 0 to 9), then
* the string is not a positive integer value.
*/
if( !Character.isDigit(ch) )
{
isNumber = false;
}
}
}
return isNumber;
}
/**
* This method iterates through all of the Dice objects in the array, determining if they are all valid.
* @param dice = Array Of Dice
* @return true if all dice inside the array are valid else false
*/
public static boolean allDiceValid(Dice dice[])
{
boolean valid = true;
for(int i = 0;i<dice.length;i++)
{
if(!dice[i].isValidDice())
{
valid = false;
break;
}
}
return valid;
}
/**
* This method adds a single Dice object into the Dice array at a specified index position.
* @param dice = Array Od Dice
* @param newDice = new dice that needs to be placed in array
* @param index = the index value at which new array is placed
* @return true if newDice is placed in array else false
*/
public static boolean addDie(Dice[] dice, Dice newDice, int index)
{
if(index < 0 && index >= dice.length)
{
return false;
}
dice[index] = newDice;
return true;
}
/**
* This method rolls all of the Dice objects in the array.
* @param dice = Array Of Dice
*/
public static void rollAllDice(Dice[] dice)
{
boolean check = allDiceValid(dice);
if(check)
{
for(int i =0;i<dice.length;i++)
{
dice[i].rollDice();
}
}
}
/**
* This method calculates the sum of all Dice object values in the array.
* @param dice = Array of Dice
* @return if any invalid dice then 0 else the sum of all the values in dice array
*/
public static int sumDice(Dice[] dice)
{
int sum = 0;
boolean check = allDiceValid(dice);
if(check)
{
for(int i =0;i<dice.length;i++)
{
sum = sum + dice[i].getValue();
}
}
return sum;
}
/**
* This method counts the number of times a target value occurs in the Dice array.
* @param dice = Array of Dice
* @param target = target value which need to be checked
* @return the number of times target value appeared
*/
public static int countDiceValue(Dice[] dice, int target)
{
int counter = 0;
boolean check = allDiceValid(dice);
if(check)
{
for(int i =0;i<dice.length;i++)
{
if(dice[i].getValue() == target)
{
counter++;
}
}
}
return counter;
}
/**
* This method determines whether the player has survived to gather mice souls on another day.
* @param dice = Array Of Dice
* @return true if player survives else false
*/
public static boolean playerSurvives(Dice[] dice)
{
boolean check = allDiceValid(dice);
if(check)
{
int fourCount = 0;
int eightCount = 0;
int threeCount = 0;
for(int i =0;i<dice.length;i++)
{
if(dice[i].getValue() == 4)
{
fourCount++;
}
if(dice[i].getValue() == 8)
{
eightCount++;
}
if(dice[i].getValue() == 3)
{
threeCount++;
}
}
if(fourCount >= 1 || eightCount >= 2 || threeCount >= 3)
{
return true;
}
}
return false;
}
/**
* This method determines the number of souls collected by the player.
* @param dice = Array of Dice
* @return = the number of souls collected
*/
public static int collectedSouls(Dice[] dice)
{
int soul = 0;
boolean check = allDiceValid(dice);
if(check)
{
for(int i =0;i<dice.length;i++)
{
if(dice[i].getValue() == 13)
{
soul = soul - 26;
}
else if(dice[i].getValue() == 6)
{
}
else
{
soul = soul + dice[i].getValue();
}
}
}
return soul;
}
/**
* This method will display to standard output all of the Dice objects in the array with one object per line.
* @param dice = Array of Dice
*/
public static void displayDice(Dice[] dice)
{
boolean check = allDiceValid(dice);
if(check)
{
for(int i = 0;i<dice.length;i++)
{
if(dice[i].getValue() == 6)
{
System.out.println(dice[i].toString()+" : rat soul");
}
else if(dice[i].getValue() == 13)
{
System.out.println(dice[i].toString()+" : cat soul");
}
else
{
System.out.println(dice[i].toString());
}
}
}
}
/**
* This method will display the game over screen when the player does not obtain the correct types of souls in a round.
* @param totalRound = total number of rounds played
* @param totalSouls = total number of souls catched
*/
public static void displayGameOver(int totalRound, int totalSouls)
{
System.out.println("--- GAME OVER ---");
System.out.println("\nYour soul joins the pile to be reaved!\n");
System.out.println("Final Game Stats");
System.out.println("--------------------");
System.out.println(String.format("%-15s", "Total Rounds: ")+totalRound);
System.out.println(String.format("%-15s", "Total Souls: ")+totalSouls);
System.out.println(String.format("%15s", "Average Souls: ")+String.format("%.2f", (float)(totalSouls/totalRound)));
}
}
Dice
import java.util.Formatter;
import java.util.Random;
// @author:
// @version: 04/042/2022 Assignment 8
// this class represent a dice with sides and value
public class Dice {
// data members
private int sides;
private int value;
//default constructor
public Dice()
{
sides = 6;
value = 1;
}
//parameterized constructor
public Dice(int sides)
{
this.sides = sides;
value = 1;
}
// Getters
// return number of sides of dice
public int getSides()
{
return sides;
}
//return the value of the dice
public int getValue()
{
return value;
}
//Setter
// set the numbers of sides for dice
public void setSides(int sides)
{
this.sides = sides;
}
// set the value of the dice
public void setValue(int value)
{
this.value = value;
}
// this method assign a random number to value
public void rollDice()
{
Random random = new Random();
value = random.nextInt(sides) + 1;
}
// this method validates if number of sides are right
public boolean isValidSides()
{
if(sides == 4 || sides == 6 || sides == 8 || sides == 10 || sides == 12 || sides == 20)
{
return true;
}
return false;
}
// this method check if the value of the dice is correct
public boolean isValidValue()
{
if(value >= 1 && value <= sides)
{
return true;
}
return false;
}
// this method check of the dice is valid or not
public boolean isValidDice()
{
return isValidSides() && isValidValue();
}
// check if the target value equal to dice value
public boolean equalsTargetValue(int targetValue)
{
return value == targetValue;
}
// check if the argument dice value equal to dice value
public boolean equalsValue(Dice thatDice)
{
return equalsTargetValue(thatDice.value);
}
// check if both the dice are equal or not
public boolean equals(Dice thatDice)
{
return sides == thatDice.sides && value == thatDice.value;
}
// this method display dice data members as a string
@Override
public String toString() {
return String.format("%3s" ,"d"+sides) +" : "+ String.format("%2s",value);
}
}