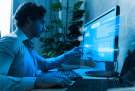
- 14th Nov 2023
- 15:54 pm
Indentation in Python is an essential aspect that defines the code's structure. It's used to denote blocks of code like loops and functions. Python, unlike other languages, doesn't use brackets or braces; instead, it employs consistent indentation, usually involving spaces or tabs. This is a defining characteristic of Python's syntax.
What is Indentation in Python?
Indentation in Python is a structural element in coding that represents the visual organization of code blocks. Unlike many other programming languages, Python doesn't use traditional braces or brackets to encapsulate blocks of code. Instead, it enforces a standardized, consistent indentation of code using spaces or tabs. Indentation is crucial in Python as it defines the scope and structure of code, particularly within control structures like loops, conditional statements, and function definitions.
The visual indentation, typically set at four spaces, determines where blocks of code begin and end. The indentation level must be consistent throughout the code to ensure it is executed correctly. This unique aspect of Python syntax enhances readability and maintains a clean and structured appearance, but it can also introduce challenges when not adhered to, as Python relies on proper indentation for program execution.
What are Rules of Indentation in Python?
Indentation in Python is a fundamental aspect of its syntax and follows specific rules to organize code blocks. The key rules governing indentation in Python include:
- Consistency: Python mandates consistent indentation within the same block of code. Mixing spaces and tabs for indentation can lead to errors. It's recommended to choose either spaces or tabs and use them uniformly throughout the code.
- Standard Size: The standard practice is to use four spaces for each level of indentation. While Python allows different indentation sizes, a consistent standard of four spaces ensures better readability and consistency.
- Nested Blocks: Code within nested blocks, such as loops or conditional statements, should maintain a consistent indentation level to represent their hierarchical structure. This structure should reflect the logical flow of the program.
- Alignment: Proper alignment aids in code readability. Elements within a block should be aligned vertically to improve code comprehension.
Adhering to these indentation rules ensures code clarity and enhances readability, making it easier for programmers to understand the logical structure of the code. Failure to follow these rules can result in syntax errors, affecting the program's functionality and readability. Therefore, observing these rules is crucial for writing clean, maintainable, and error-free Python code.
Indentation in Python For Loop
In Python, indentation plays a critical role in for loops to demarcate the block of code to be executed iteratively. When using a for loop, the code indented following the loop definition determines the block of code that will be repeatedly executed for each iteration.
For example, consider the following code snippet:
for i in range(5):
print(i)
print("Inside the loop")
print("Outside the loop")
In this case, the indented statements following the "for" loop definition, namely print(i) and print("Inside the loop"), are executed for each iteration. However, the statement print("Outside the loop") is outside the loop's indented block and thus gets executed only once, after the loop completes.
Indentation in for loops, as with any other block of code in Python, is crucial for maintaining the correct structure and functionality of the loop, ensuring the accurate execution of code within the loop and distinguishing it from the code outside the loop.
How To Fix Indentation Error in Python?
Fixing indentation errors in Python is crucial to ensure the code runs properly. Here are steps to resolve indentation issues:
- Check Consistency: Verify that the indentation in the code is uniform throughout. Python requires consistent use of spaces or tabs but not a mix of both.
- Use Text Editors with Indentation Guides: Editors like Visual Studio Code, PyCharm, or Sublime Text often offer indentation guides or color distinctions for better visualization of the code's indentation.
- Be Mindful of Mixing Spaces and Tabs: In Python, mixing spaces and tabs can cause indentation errors. Configure your editor to use either spaces or tabs consistently.
- Utilize Linters: Linters such as Pylint or flake8 can assist in identifying and correcting indentation issues.
- Check for Mismatched Indentation: Be watchful for inconsistent indentation within the same block of code, as this can lead to errors.
- Inspect for Unnecessary Indentation: Sometimes, code may be indented more than necessary. Make sure that the indentation level aligns logically with the code structure.
Careful observation, using supportive tools, and maintaining consistency in indentation practices are key strategies to rectify indentation errors in Python.
Example of Handling Indentation Error:
In Python, rectifying indentation errors involves ensuring proper space or tab indentation within the code. Here's an example where an "IndentationError" is handled using a 'try-except' block to catch and address indentation issues in the code.
try:
if True:
print("Indentation error fixed!") # Missing indentation causing an error
except IndentationError:
print("Fix indentation issue to resolve error.")
Advantages of Indentation in Python
Indentation in Python brings several advantages:
- Improved Readability: Python's enforced indentation enhances code readability by making the code structure more apparent.
- Consistency: The required indentation fosters consistent coding practices among developers, contributing to more standardized code.
- Reduced Syntax: Python's structural simplicity, partially achieved through indentation, reduces unnecessary syntax.
- Reduced Errors: The enforced indentation helps catch errors early, preventing common programming mistakes.
- Increased Efficiency: Despite potential initial learning curves, the discipline of using indentation streamlines coding and often makes it more efficient.
- Imposes Structure: Indentation enforces code structure, enabling easy identification of blocks and nesting, leading to clearer program organization.
Disadvantages of Indentation in Python
Indentation in Python, while beneficial, does have certain disadvantages:
- Limited Flexibility: The enforced indentation makes Python less flexible compared to languages that use brackets or other symbols to delineate code blocks. This can be restrictive, especially for developers accustomed to different coding styles.
- Difficulties with Copy and Paste: Indentation can sometimes create issues when code is copied and pasted from external sources or if the indentation levels differ.
- Increased Learning Curve: Developers new to Python might initially struggle with mastering the indentation rules, leading to a steeper learning curve.
- Maintenance Issues: Modifying code with different indentation styles or adjusting existing code to conform to a new indentation style can be challenging, leading to maintenance difficulties.
- Code Collaboration Challenges: When multiple developers with varying indentation styles collaborate on a project, it might cause confusion and inconsistencies in code formatting.