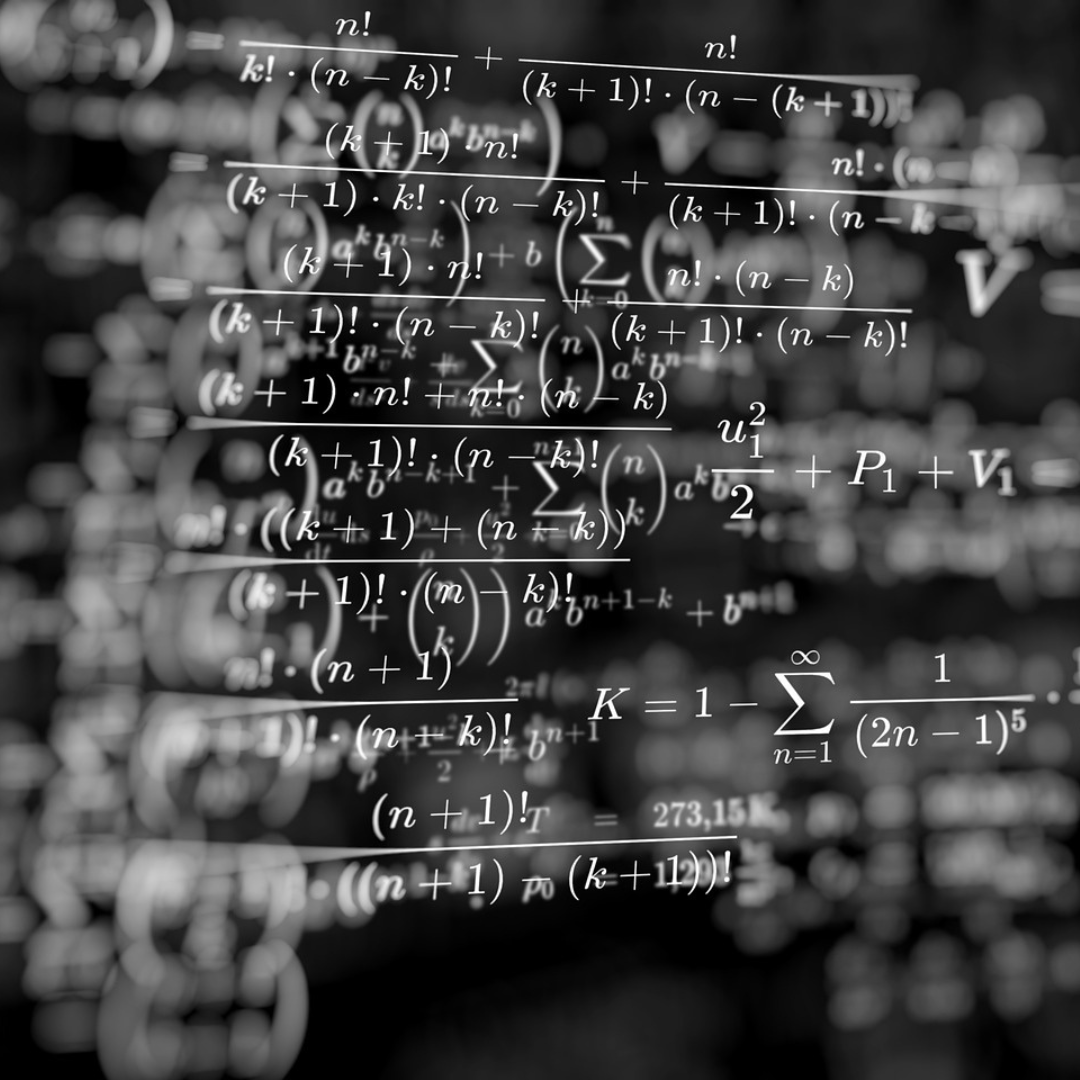
- 3rd Apr 2023
- 08:42 am
Java Homework Help
Java Homework Question
Heron's Formula using Math Class - Write an app that reads the lengths of the sides of a triangle from the user. Compute the area of the triangle using Heron's formula in which 's' represents half of the perimeter of the triangle, and 'a' and 'b' and 'c' are the lengths of the three sides. Print area to three decimal places.
Java Homework Solution
Here's the Java code for the app that reads the lengths of the sides of a triangle from the user, computes the area of the triangle using Heron's formula, and prints the area to three decimal places:
import java.util.Scanner;
public class HeronFormula {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// Read the lengths of the three sides of the triangle
System.out.print("Enter the length of side a: ");
double a = input.nextDouble();
System.out.print("Enter the length of side b: ");
double b = input.nextDouble();
System.out.print("Enter the length of side c: ");
double c = input.nextDouble();
// Calculate half of the perimeter of the triangle
double s = (a + b + c) / 2;
// Calculate the area of the triangle using Heron's formula
double area = Math.sqrt(s * (s - a) * (s - b) * (s - c));
// Print the area of the triangle to three decimal places
System.out.printf("The area of the triangle is %.3f\n", area);
input.close();
}
}
When you run this code and enter the lengths of the sides of the triangle, the program calculates the area using Heron's formula and prints it to three decimal places. For example:
Enter the length of side a: 3
Enter the length of side b: 4
Enter the length of side c: 5
The area of the triangle is 6.000
Note that we used the Math.sqrt() method to calculate the square root of a number, and the printf() method with the %f format specifier to print the area to three decimal places.