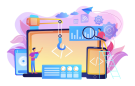
- 15th Nov 2023
- 00:22 am
- Admin
What is HashMap in Java?
A widely used Java implementation of the Map interface, HashMap, acts as a repository for key-value pairs. Its underlying data structure relies on a hash table, facilitating swift insertion, deletion, and retrieval operations. Within the HashMap class, items are organized as an array of linked lists. Each array element serves as a bucket, and linked lists come into play to manage collisions—instances where different keys hash to the same index. This design ensures efficient storage and retrieval of data within the HashMap. The following are HashMap's primary properties and functionality:
- Key-Value Storage: HashMap supports key-value pair storage and allows for a single null key and numerous null values.
- Efficient Operations: Because of its hash-based structure, simple operations like get and put have constant-time average complexity, making it exceptionally efficient for data retrieval and insertion.
- No Order Guarantee: Elements are not kept in any certain order. While iteration sequence may appear to be consistent, this is not guaranteed.
- Unique Keys: HashMap prohibits duplicate keys. When a duplicate key is inserted, the prior mapping is replaced.
- Resizable: When the load factor surpasses a predetermined threshold, HashMap dynamically resizes itself by rehashing, guaranteeing efficient performance.
HashMaps are often employed in applications that demand rapid access to key-value pairs. They provide fast data retrieval and insertion, making them a popular choice for handling key-value associations in Java systems.
Syntax of HashMap in Java with explanation
In Java, the HashMap class provides a way to store key-value pairs using a hash table-based implementation. Here's the syntax of the HashMap class along with an explanation:
```
import java.util.HashMap;
// Declaration and instantiation of a HashMap
HashMap<KeyType, ValueType> mapName = new HashMap<>();
```
`import java.util.HashMap;`: This line imports the `HashMap` class from the `java.util` package, allowing its use in the current Java file.
`HashMap<KeyType, ValueType> mapName = new HashMap<>();`:
- `HashMap`: This is the class name indicating the use of a hash table-based map implementation.
- `<KeyType, ValueType>`: These placeholders represent the types of the keys and values that the map will store. Replace `KeyType` and `ValueType` with the actual types you intend to use.
- `mapName`: It is the variable name representing the HashMap instance. Replace `mapName` with a meaningful identifier.
- Instantiation: The `new HashMap<>()` part creates a new HashMap instance. The empty angle brackets (`<>`) use type inference to match the declared `KeyType` and `ValueType`. It's important to specify the actual types in the angle brackets, e.g., `HashMap<String, Integer> map = new HashMap<>();`, for creating a map that stores String keys and Integer values.
- Note: Replace `KeyType` and `ValueType` with the specific data types of keys and values you want to store in the HashMap.
This syntax generates a HashMap object capable of storing key-value pairs of the provided kinds. It is critical to describe the types precisely in order to ensure correct data usage and retrieval in the HashMap.
Uses of HashMap in Java
HashMap in Java is widely used in various scenarios due to its key-value pair storage and efficient data retrieval capabilities. Its principal applications include:
- Data Storage and Retrieval: This is the process of storing and retrieving key-value pairs. HashMap effectively links keys with values, enabling for rapid key-based data retrieval.
- Cache Implementation: HashMaps are used in caching techniques where rapid access to stored data is required. They provide quick access to stored items.
- Indexes in Databases: HashMaps are used in database management systems to generate in-memory indexes that provide quick access to certain entries.
- Implementing Counters and Frequencies: Used to keep track of item counts or frequencies. For example Tracking the occurrences of items in a collection.
- API Development: HashMaps are handy for specifying parameter choices while creating APIs. By accepting a restricted number of keys, they assure genuine and clearly comprehensible inputs.
- Associative Arrays: Although Java lacks built-in support for associative arrays, HashMaps fulfill this function by mapping keys to values in a manner similar to associative arrays.
- Dictionaries and Lookup Tables: Used as lookup tables and dictionaries, allowing for quick access to values via their related keys.
- Storage Configuration Options: Configuration or setting storage in programs. HashMaps provide an easy way to store and retrieve properties using key-value pairs.
Overall, HashMaps are fundamental data structures used in Java for various purposes, particularly for efficient storage, retrieval, and manipulation of key-value pairs in diverse applications and scenarios.
Advantages of HashMap in Java
The HashMap class in Java provides a key-value pair storing system with the following benefits:
- Efficient Retrieval and Insertion: HashMap allows for quick access to values based on keys as well as efficient key-value pair insertion.
- Data Retrieval with Keys: It allows data to be obtained using unique keys, allowing for rapid search without having to crawl through the full data structure.
- Versatile Storage Mechanism: HashMaps may store numerous data types as keys and values, providing flexibility in managing different data types in the form of key-value pairs.
- Dynamic Size: The size of HashMap can dynamically increase or decrease dependent on the amount of items it contains, thereby changing its capacity.
- No Duplicate Keys: A HashMap's keys must be unique. When a duplicate key is introduced, the prior value is replaced, preventing duplicate entries.
- Data Structure Versatility: HashMaps provide a fundamental data structure that is suited for a wide range of applications, including caching, indexing, and associative arrays.
- Java Collections Framework Implementation: HashMap, which is part of the Java Collections Framework, provides a standard approach to maintain key-value pairs, effortlessly integrating with other collection classes.
- API Design and Implementation: It is commonly used in the design of APIs and data structures, as well as in the implementation of bespoke collections and applications.
Overall, HashMap is favored for its efficiency in data retrieval, versatility in handling key-value pairs, and adaptability in a wide range of scenarios, making it a key component in Java programming for managing associative data.
Constructor in HashMap
In Java, the 'HashMap' class has numerous constructors for creating HashMap objects with varying starting capacity and load factors. The major builders are as follows:
- HashMap(): This constructor returns an empty HashMap with a load factor of 0.75 and a default starting capacity of 16. Based on these default values, it allocates memory space for storing key-value pairs.
- HashMap(int initialCapacity): creates an empty HashMap with the supplied initial capacity. The load factor is set to 0.75 by default, and the capacity must be a positive integer.
- HashMap(int initialCapacity, float loadFactor): Returns an empty HashMap with the initial capacity and load factor supplied. The load factor is the point at which the HashMap resizes itself. A greater load factor allows for more keys and values before resizing but may increase the likelihood of collisions.
These constructors allow developers to create HashMap objects with different starting capacity and load factors based on the needs of their applications. The default constructor generates a HashMap with the default starting capacity and load factor, but the other builders enable you to customize these parameters to maximize HashMap performance in various usage circumstances.
Example Program using HashMap
A Java program that utilizes HashMap to store country names as keys and their corresponding capital cities as values:
```
import java.util.HashMap;
public class CountryCapitalMap {
public static void main(String[] args) {
// Creating a HashMap to store country-capital pairs
HashMap<String, String> countryCapitalMap = new HashMap<>();
// Adding country-capital pairs to the HashMap
countryCapitalMap.put("USA", "Washington, D.C.");
countryCapitalMap.put("UK", "London");
countryCapitalMap.put("France", "Paris");
countryCapitalMap.put("Germany", "Berlin");
countryCapitalMap.put("Japan", "Tokyo");
// Retrieving and displaying capital for a specific country
String capital = countryCapitalMap.get("France");
System.out.println("The capital of France is: " + capital);
// Displaying all country-capital pairs
System.out.println("\nCountry-Capital Pairs:");
for (String country : countryCapitalMap.keySet()) {
String capitalCity = countryCapitalMap.get(country);
System.out.println(country + " - " + capitalCity);
}
}
}
```
Explanation:
- The program creates a HashMap `countryCapitalMap` to store country-capital pairs, with countries as keys and their respective capital cities as values.
- It adds key-value pairs using the `put()` method and retrieves the capital of a specific country using `get()`.
- The program demonstrates accessing and displaying all country-capital pairs using a loop to iterate through the keys and retrieve the corresponding values.
- This simple program illustrates how a HashMap can efficiently store and retrieve key-value pairs, providing a flexible way to manage associations between countries and their capitals.