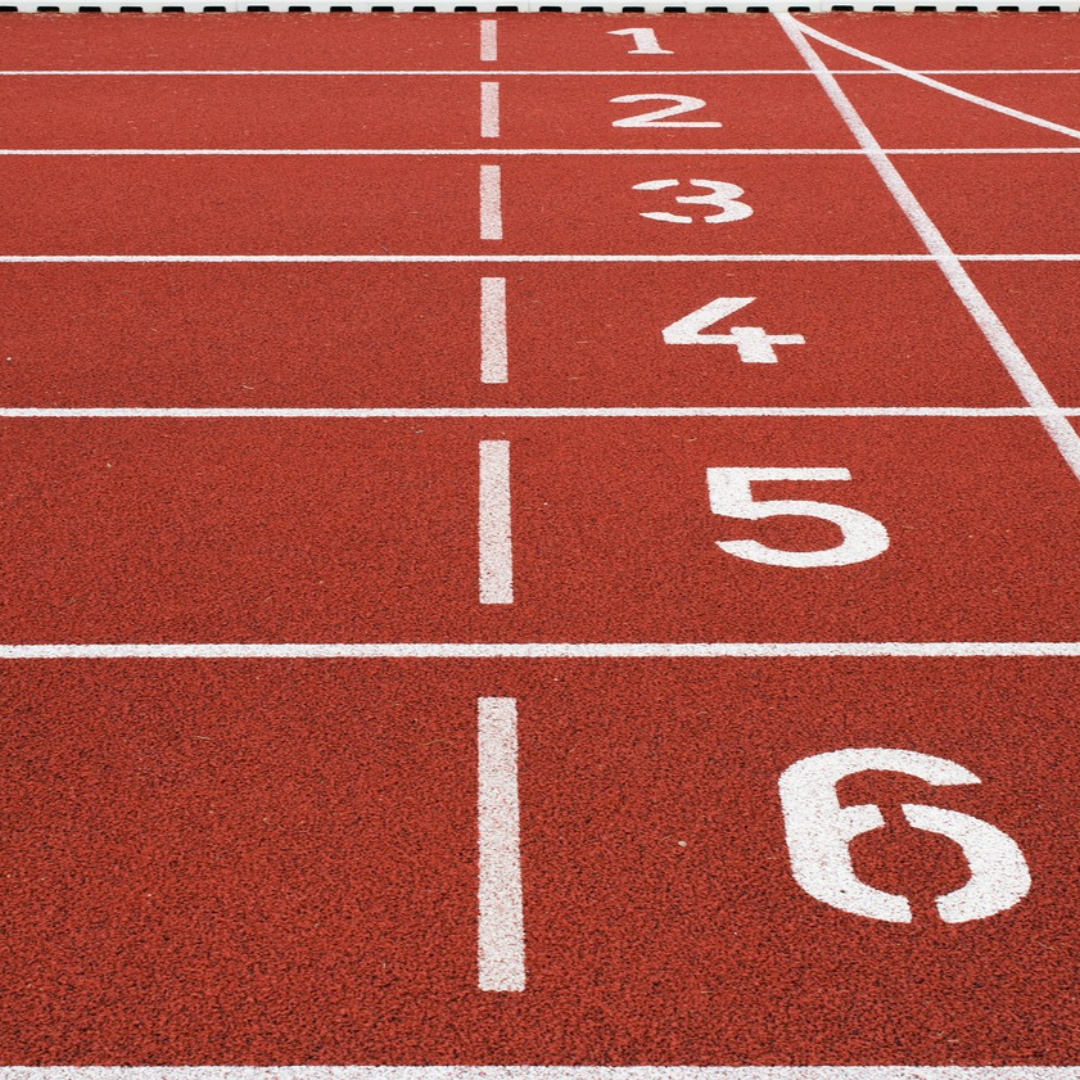
- 2nd Apr 2023
- 05:11 am
C++ Homework Help
C++ Homework Question
C++ Guess The Number Game - Write a program that generates a random number and asks the user to guess what the number is. If the user's guess is higher than the random number, the program should display "Too high, try again". If it is lower, it should display "Too low, try again". The program should use a loop that repeats until the user correctly guesses the random number.
C++ Homework Solution
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int main() {
// Generate a random number between 1 and 100
srand(time(0)); // Seed the random number generator with the current time
int randomNumber = rand() % 100 + 1;
// Ask the user to guess the number and loop until they guess correctly
int guess;
do {
cout << "Guess the number between 1 and 100: ";
cin >> guess;
if (guess > randomNumber) {
cout << "Too high, try again." << endl;
} else if (guess < randomNumber) {
cout << "Too low, try again." << endl;
} else {
cout << "Congratulations, you guessed the number!" << endl;
}
} while (guess != randomNumber);
return 0;
}
This solution uses a do-while loop that continues to ask the user for their guess until they guess correctly. Inside the loop, we use an if-else statement to check if the guess is higher or lower than the random number and give the user feedback accordingly. When the user finally guesses the number, we display a congratulatory message and exit the loop.
Note that we use srand() and rand() from the <cstdlib> library, along with time() from the <ctime> library, to generate a new random number each time the program is run. Without srand(), the program would always generate the same random number, making it less fun to play.