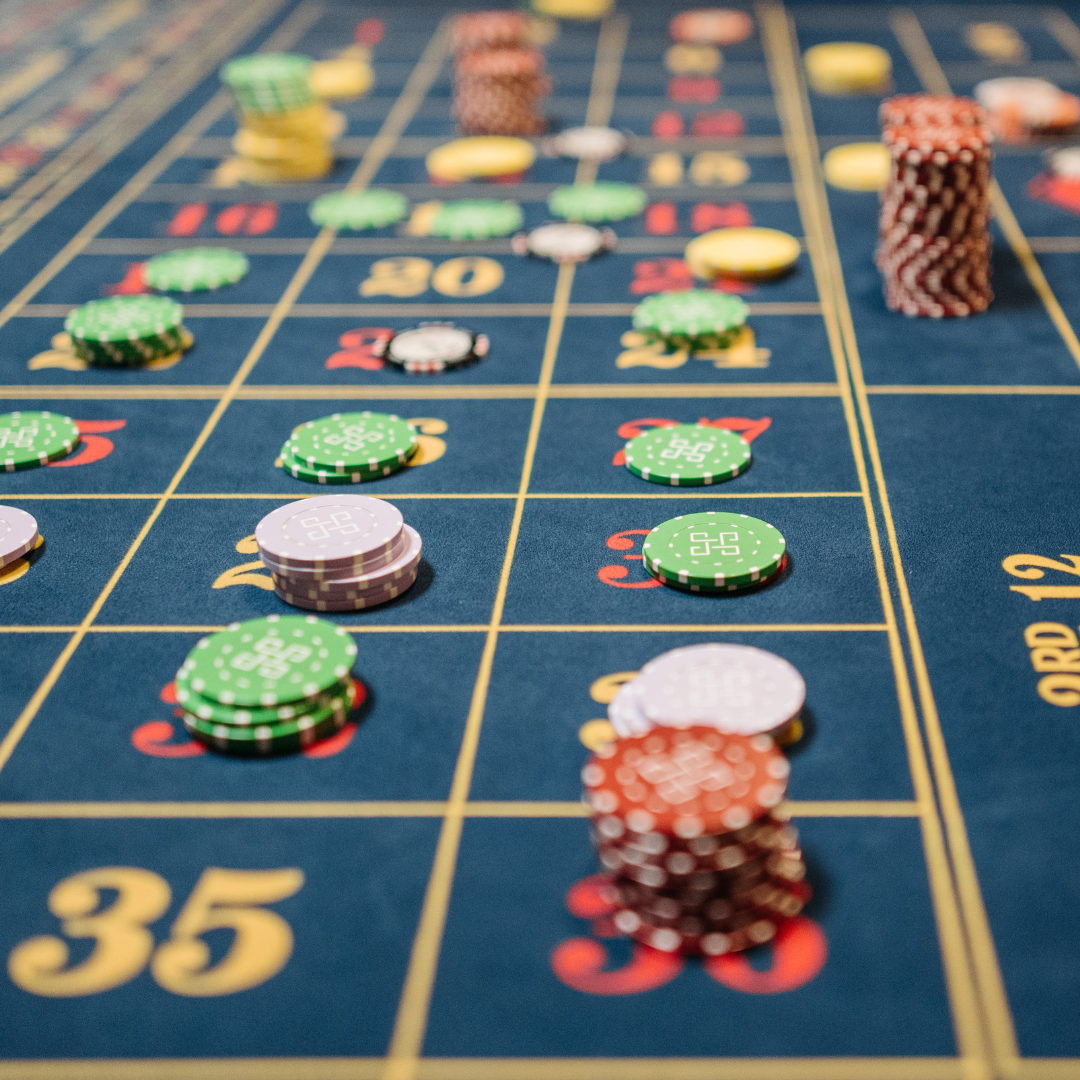
- 5th Apr 2023
- 09:39 am
- Admin
Python Homework Help
Python Homework Question
Python Logical Puzzles, Games, and Algorithms: Game of Craps
Python Homework Solution
Here's a Python program for the game of Craps:
import random
def roll_dice():
"""Roll two dice and return their face values as a tuple."""
die1 = random.randint(1, 6)
die2 = random.randint(1, 6)
return (die1, die2)
def play_game():
"""Play one round of the game of craps and return the result."""
roll = roll_dice()
total = sum(roll)
print("You rolled", roll, "for a total of", total)
if total in (7, 11):
print("You win!")
return True
elif total in (2, 3, 12):
print("You lose!")
return False
else:
print("The game continues...")
point = total
while True:
roll = roll_dice()
total = sum(roll)
print("You rolled", roll, "for a total of", total)
if total == point:
print("You rolled your point! You win!")
return True
elif total == 7:
print("You rolled a 7! You lose!")
return False
def main():
"""Play multiple rounds of the game of craps until the user quits."""
while True:
play_game()
response = input("Do you want to play again? (y/n) ")
if response.lower() != "y":
break
if __name__ == "__main__":
main()
This program first defines a roll_dice function that returns the face values of two dice rolled together. It then defines a play_game function that plays one round of the game of Craps. If the first roll of the dice results in a 7 or 11, the player wins. If it results in a 2, 3, or 12 (known as "craps"), the player loses. Otherwise, the game continues, and the player tries to roll the same total again before rolling a 7.
Finally, the main function repeatedly calls play_game until the user chooses to quit.