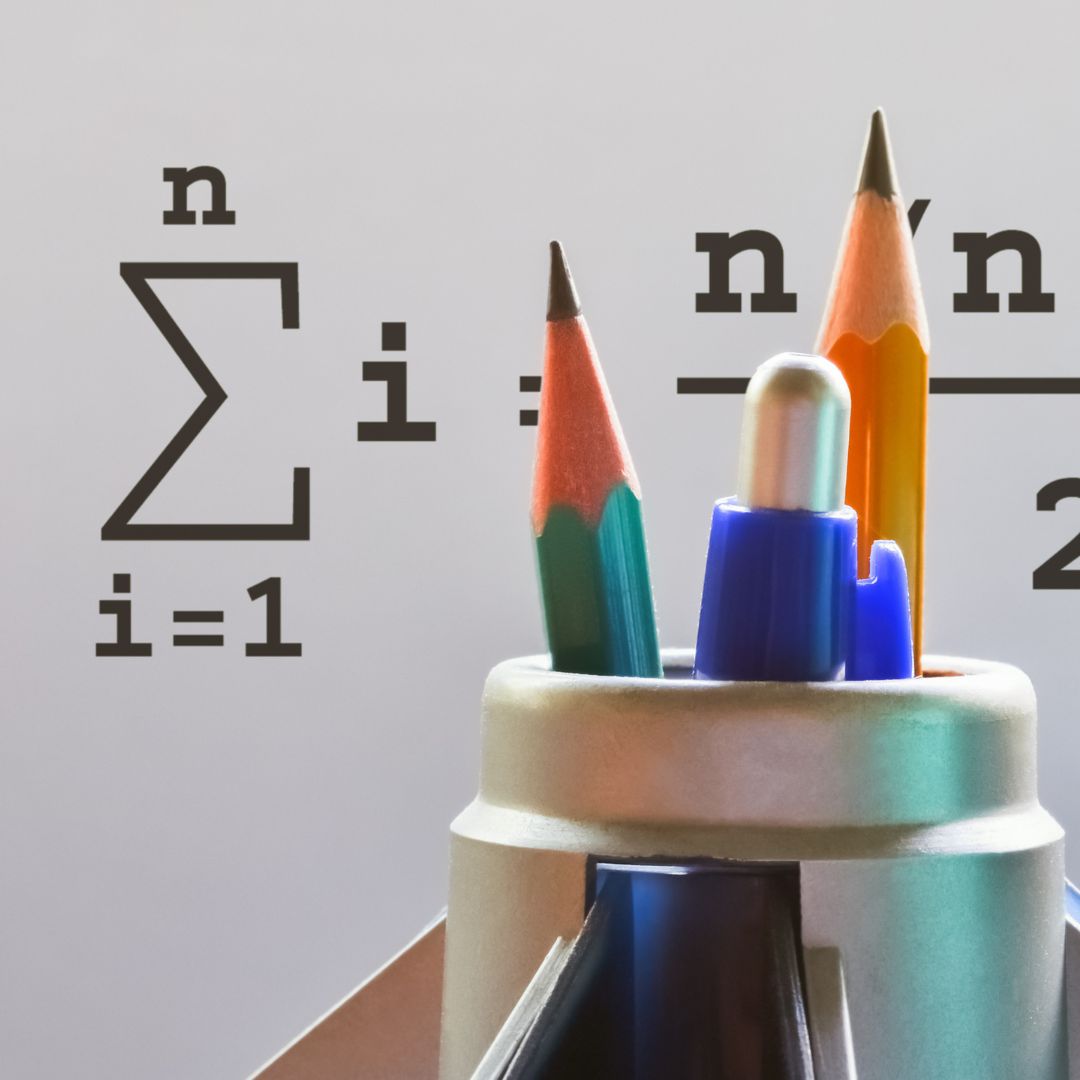
- 19th Mar 2023
- 03:12 am
C++ Programming Assignments for Beginners
Question - Finding Largest and Smallest Integer - Using C++ While Loops and Control Structures Write a program with a loop that lets the user enter a series of integers. The user should enter -99 to signal the end of the series. After all the numbers have been entered, the program should display the largest and the smallest numbers entered
C++ Homework Solution
Here's a C++ program that finds the largest and smallest integer from a series of numbers entered by the user using a while loop and control structures:
#include
using namespace std;
int main() {
int num, largest = INT_MIN, smallest = INT_MAX;
cout << "Enter a series of integers (-99 to stop):" << endl;
while (true) {
cin >> num;
if (num == -99) {
break;
}
if (num > largest) {
largest = num;
}
if (num < smallest) {
smallest = num;
}
}
cout << "The largest number is " << largest << endl;
cout << "The smallest number is " << smallest << endl;
return 0;
}
In this program, we use a while loop to keep asking the user for integers until they enter -99. Inside the loop, we use if statements to check if the current number is larger or smaller than the previous largest or smallest number respectively. If it is, we update the values of largest and smallest accordingly. Once the loop ends, we display the values of largest and smallest.
Here's an example of what the output of the program might look like:
Enter a series of integers (-99 to stop):
10
-5
25
7
0
-99
The largest number is 25
The smallest number is -5