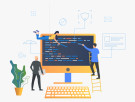
- 14th Nov 2023
- 22:30 pm
- Admin
In Java, the EnumMap class is a customized Map implementation designed for usage with enum types as keys. When the keys are enum constants, it is intended to give great performance and memory efficiency. EnumMap is an extension of AbstractMap that is only dedicated to dealing with enum keys, providing a specific storage mechanism for enum-based key-value mappings.
Key Qualities and Features of the EnumMap
The following are the key qualities and features of the EnumMap:
- EnumMap is specifically designed to work with enum constants as keys, ensuring type safety and performance.
- Implementation Optimization: It internally stores the mappings in an array, using the ordinal values of enum constants as indices for easier access and retrieval.
- Performance Advantages: Because of the underlying array structure, EnumMap is very efficient and designed for enum-based mappings, resulting in speedier operations.
- No Null Keys: To ensure type safety and to use the ordinal values of enum constants for map storage, EnumMap does not allow null keys.
- Enum Constant Iteration Order: EnumMap respects the natural order of enum constants, enabling iteration in the same order as the enum type defines.
This customized map type is especially useful when working with enum-based keys since it allows efficient and type-safe key-value mappings to enum constants. It is frequently used in circumstances where enum keys are required to construct mappings because it provides high-performance, type-specific storage and efficient iteration across enum constant entries.
Syntax for Enum in Java
The basic syntax for creating an enum type in Java is to use the 'enum' keyword followed by the name of the enumeration and a list of constants defining the members within the enum. The general syntax is as follows:
```
enum EnumName {
CONSTANT_1,
CONSTANT_2,
// ... additional constants
}
```
Here's a breakdown of the syntax elements:
- 'enum': A Java term that specifies an enumeration. It is followed by the name of the new enumeration type.
- 'EnumName': The name of the enum type, which represents a collection of constants or items.
- 'CONSTANT_1', 'CONSTANT_2', and other constants: These are the enum items that each serves as a named constant. By tradition, these constants are separated by commas and written in uppercase.
Example:
```
enum Days {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
}
```
In this example, an enum type named 'Days' is declared, with constants representing each day of the week. Enums allow you to create a fixed set of constants that are relevant inside the context of the program, which improves code readability and maintainability.
What are the Uses of Enum in Java
Enums are a powerful technique to describe a fixed collection of constants in Java and are frequently used for a variety of purposes:
- Improved Readability: Enumerations describe constant values more clearly, making code more intelligible and self-explanatory. For example, enum constants such as 'RED,' 'GREEN,' and 'BLUE' are more descriptive than numeric constants.
- Type Safety: Enums offer type safety by confining input to a defined set of constants, avoiding unexpected values. This decreases the likelihood of runtime problems caused by erroneous inputs.
- Improved Maintainability: Enums increase code maintainability by declaring a group of related constants in a single location. As changes are reflected across the codebase, it becomes easy to modify or add new constants.
- Switch Statements: Enums are commonly used with switch statements, providing a cleaner and more manageable structure for conditional logic. This helps handle different cases associated with enum constants.
- Better APIs: Enums are commonly used in APIs to construct parameters that accept a small and well-defined range of possibilities. This assures readability and limits input to known valid values.
- Singleton Pattern: Enum types may be used in Java to implement the singleton pattern, ensuring that a class only has one instance.
Enums are useful for encoding fixed sets of constants in Java because they increase code quality, readability, maintainability, and type safety while allowing for efficient and systematic handling of related constant values across the code.
Advantages of Enum in Java
Because of their specified collection of constants, enumerations (Enums) in Java have various advantages. Among the many advantages are:
- Type Safety: Enums offer type safety by allowing the compiler to notice problems during compilation and guaranteeing that only valid and specified constants are utilized.
- Readability and Clarity: Enums improve code readability by giving descriptive, self-explanatory constant names, which makes the code easier to comprehend and maintain.
- Reduced mistakes: Using Enums decreases the possibility of mistakes caused by mistyped or incorrect constants, enhancing the code's resilience and dependability.
- Enumeration of Constants: Enums make it easier to iterate over a defined set of constants, allowing for simple enumeration and simplifying jobs that need a range of constants.
- Avoidance of Magic Numbers: Enums aid in the elimination of "magic numbers" (hard-coded numerical values) in code by replacing them with descriptive and self-explanatory constant names.
- Code Consistency: Enums offer a single place for defining and managing a connected set of constants, supporting code consistency throughout the program.
- Switch-Case Enhancement: Enums complement switch-case statements, improving readability and organization by giving a simpler, more succinct approach to handle multiple scenarios.
Enums in Java help to write more manageable, comprehensible, and error-resistant code, enhancing code quality and lowering the risk of inconsistencies or runtime problems caused by the usage of arbitrary or ambiguous constants.
What is EnumMap and how it is different from Enum?
An EnumMap is a Java Map implementation that is specifically built to deal with enum keys. When enum constants are utilized as keys in a map structure, it provides exceptional performance and efficiency.
Key EnumMap Features:
- Strictly for Enum Keys: EnumMap is designed to exclusively function with enum types as keys. It is especially built to hold key-value pairs using enum constant keys.
- High Performance: The internal EnumMap implementation stores key-value pairs in an array, using the ordinal values of enum constants as array indices, resulting in quick access and retrieval.
- No Null Keys: EnumMap, like other Map implementations, does not support null keys. By only taking enum constants as keys, it maintains type safety.
- Preserves Enum Constant Order: - EnumMap preserves the natural order of enum constants, mirroring the order in which they are stated.
In Java, on the other hand, an enum specifies a fixed collection of constants that are handled as distinct types. Enums specify a set of linked constants, whereas EnumMap is a customized map implementation that works with these enum constants as keys.
While enums provide a set of related constants, EnumMap is used to effectively correlate values with these enum constants, providing a high-performance map structure optimized for enum-based key-value pairings.
Example EnumMap in Java
A Java program that demonstrates the use of an enum to represent different days of the week:
```
// Define an enum for Days of the Week
enum Day {
SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY
}
public class EnumExample {
public static void main(String[] args) {
// Access enum constants
Day today = Day.MONDAY;
// Switch-case based on the enum value
switch (today) {
case MONDAY:
System.out.println("It's Monday!");
break;
case FRIDAY:
System.out.println("It's Friday!");
break;
default:
System.out.println("It's not Monday or Friday.");
}
// Loop through all enum values
System.out.println("\nAll days of the week:");
for (Day day : Day.values()) {
System.out.println(day);
}
}
}
```
Explanation:
- An enum `Day` is defined to represent the days of the week.
- The `main` method demonstrates how to use the enum by assigning a day to `today` and using a switch-case to check and print a message based on the day.
- A loop iterates through all the enum values using the `values()` method to display all days of the week.
This program showcases the usage of enums in Java to define a set of related constants and highlights how enums can be used in switch-case constructs and iteration through all defined enum constants.