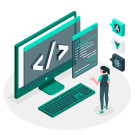
- 14th Nov 2023
- 21:48 pm
- Admin
Encapsulation in Java refers to the bundling of data and methods within a single class, shielding the data from direct external access and preserving the object's internal state. This approach is crucial for enhancing security and controlling access to data within an object, ensuring proper functionality and reducing potential errors.
What is Encapsulation in Java?
In Java, encapsulation embodies the idea of bundling data (like variables) and functions together within a unified entity or class. It aims to shield the data from external interference and enforce secure access through methods. The primary objective is to preserve an object's internal state, safeguarding the integrity of its data and intended operations.
By encapsulating data, the internal workings are concealed, permitting controlled access to the object's attributes. This concept significantly contributes to the object-oriented programming (OOP) paradigm, enabling better management of complexity, reusability, and easier maintenance of code. Encapsulation fundamentally fosters the concept of information hiding, providing an additional layer of security and stability to the program.
Encapsulation is a technique that structures a class to protect data integrity by restricting direct access to certain elements within it. This approach enhances the ability to manage alterations to data and reduces the risk of unintentional interference from outside influences. This fundamental concept is a cornerstone of Java programming, promoting modularity, maintainability, and enhanced security in software development.
How Encapsulation is Achieved in Java
Within Java, encapsulation is carried out by marking class attributes as private, preventing direct access from external sources. Accessing these private attributes involves using particular methods known as getters and setters, which permit controlled interaction with these encapsulated elements. Getters allow retrieving the values of these attributes, while setters enable their modification. This approach maintains a strict separation between the class's internal data representation and the methods for interacting with that data.
When attributes are designated as private, their access is limited solely to within the class where they are defined. Public methods (getters and setters) allow controlled, secured access to these private attributes, preserving the data integrity and providing flexibility to validate, modify, or retrieve the data according to the defined conditions within the class.
The careful regulation of access to internal attributes guarantees that any alterations or interactions with the data pass through predefined methods. This maintains a clear, controlled pathway for managing and retrieving data, ensuring a strong and secure structure for the object's information.
Implementation of Encapsulation in Java
In Java, the process of encapsulation involves marking class variables as private and providing public methods to indirectly handle or access these variables. For instance, consider a class that has private variables (attributes) and public methods (getters and setters). These methods enable the manipulation and retrieval of private variables from external sources, allowing for the reading, modification, and setting of these encapsulated elements.
By maintaining variables as private and permitting access solely through methods, encapsulation guarantees data security while providing the flexibility to execute further operations such as validation, assignment, or modifications exclusively within the class. Such an approach strengthens the internal architecture of a class, establishing a protected way to interact with its attributes. This ensures data integrity and bolsters overall security within the program.
Demonstrate Encapsulation with Code Examples
The provided Java code snippets demonstrate encapsulation. They showcase private member variables (such as 'age' and 'name') along with public methods (getters and setters) to access and alter these variables. Encapsulation limits direct access to data, enabling controlled data manipulation via methods, thereby ensuring data integrity and security.
- Example 1:
public class Student {
private int age;
private String name;
public int getAge() {
return age;
}
public void setAge(int newAge) {
if (newAge > 0) {
age = newAge;
} else {
System.out.println("Invalid age");
}
}
public String getName() {
return name;
}
public void setName(String newName) {
if (!newName.isBlank()) {
name = newName;
} else {
System.out.println("Invalid name");
}
}
}
- Example 2:
public class BankAccount {
private double balance;
public double getBalance() {
return balance;
}
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
} else {
System.out.println("Invalid amount to deposit");
}
}
public void withdraw(double amount) {
if (amount > 0 && balance >= amount) {
balance -= amount;
} else {
System.out.println("Invalid amount to withdraw");
}
}
}
Advantages of Encapsulation in Java
Encapsulation in Java offers numerous advantages:
- Data Protection: Encapsulation protects the data within a class from external interference, safeguarding it from unauthorized access or modification.
- Controlled Accessibility: It allows controlled access to class variables, ensuring that sensitive data is not directly exposed.
- Modularity and Flexibility: Encapsulation promotes modularity by allowing changes within the class without affecting the code using the class, enhancing flexibility in design.
- Code Reusability: It facilitates reusing code and accelerates the development process by allowing the reuse of objects created in the class.
- Maintainability: Encapsulation improves code maintenance by concealing the internal mechanisms of a class, thereby simplifying the task of maintaining and altering the code.
- Enhanced Security: Encapsulation strengthens data security, minimizing the chances of unauthorized access and misuse.
Disadvantages of Encapsulation in Java
Encapsulation in Java, while offering numerous benefits, also has certain limitations:
- Complexity: Overuse of encapsulation might result in excessive complexity in the class structure, making it harder to comprehend and maintain.
- Reduced Direct Access: Encapsulation limits direct access to class variables, which might impede performance in scenarios where direct data manipulation is crucial.
- Additional Code Overhead: The utilization of getter and setter methods in implementing encapsulation may lead to increased overhead, potentially causing expansion of the codebase.
- Increased Indirection: Employing getter and setter methods can introduce additional layers of indirection, potentially diminishing code readability in specific scenarios.
- Increased Development Time: The incorporation of encapsulation demands extra design and coding effort, which can potentially lengthen the development process.