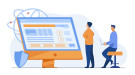
- 14th Nov 2023
- 15:23 pm
- Admin
Depth-First Search (DFS) in Python is an algorithm utilized for traversing a graph or tree by systematically exploring each branch's depth before backtracking. It operates by systematically visiting all vertices, starting from a selected node. Depth-First Search (DFS) is an algorithm implemented using either a stack or recursion, starting at the initial vertex and exploring as far as possible along each branch. This Python-based algorithm tracks visited nodes and explores unvisited neighbors, following a systematic depth-first approach.
What is DFS?
Depth-First Search (DFS) is a fundamental graph traversal algorithm used to systematically explore a graph or tree's vertices and edges. This algorithm follows a depth-first approach, diving as deep as possible along each branch before backtracking. The main goal of DFS is to systematically visit each node in the graph by following a depth-first manner.
In Depth-First Search (DFS), the process begins by selecting a starting vertex, and then it systematically explores as deeply as it can along each pathway before it doubles back and backtracks to examine the remaining options. It uses a stack or recursion method to track visited nodes and explore unvisited neighbors, making the approach systematic. This process is continued until every reachable node has been visited.
One characteristic of DFS is its simplicity, making it easy to understand and implement. However, it's crucial to manage the visited nodes and avoid infinite loops in cyclic graphs. This algorithm is highly versatile and has diverse applications in various fields such as data analysis, network flow analysis, and solving puzzles.
DFS Algorithm
The Depth-First Search (DFS) algorithm operates by exploring a graph or tree, starting from a chosen source node. It delves as deeply as feasible into each branch before retracing its steps or backtracking. The algorithm utilizes a stack or recursion method to maintain a record of visited nodes and explore unvisited neighbors. This process continues until all reachable nodes have been visited.
The algorithm's depth-first approach provides simplicity and a systematic traversal method. To avoid infinite loops in cyclic graphs, it manages visited nodes efficiently. The core principle of DFS lies in its stack or recursive method, allowing for an orderly exploration of the graph's nodes.
DFS Pseudocode
Depth-First Search (DFS) is an algorithm used to traverse through tree or graph structures. It commences at a designated node and thoroughly explores each branch before backtracking. This traversal strategy delves deeply into the structure, aiming to visit the farthest nodes first, which lends the algorithm its name. By maintaining a stack or recursive approach, DFS proceeds to explore all available paths, allowing for a detailed examination of the graph or tree structure. This strategy helps in understanding the depth and structure of the network while efficiently searching for specific targets.
The pseudocode for DFS is:
DFS(vertex):
visited[vertex] = true
for each neighbor in vertex's neighbors:
if neighbor is not visited:
DFS(neighbor)
DFS Implementation in Python, Java and C/C++
The Depth-First Search (DFS) algorithm can be implemented in various programming languages like Python, Java, and C/C++. The approach primarily involves employing a stack or a recursive method to systematically explore the graph or tree data structures. Utilizing the respective syntax and structures of each language, DFS can be coded efficiently. For Python, it often involves using a list as a stack or implementing a recursive function. In Java, the algorithm may be implemented with a stack or recursion. In C/C++, DFS can be coded using arrays, linked lists, and recursive functions, ensuring the exploration of the graph's vertices and edges.
Here are the codes in the preferred sequence:
- Python Implementation:
def DFS(graph, vertex, visited):
visited[vertex] = True
for neighbor in graph[vertex]:
if not visited[neighbor]:
DFS(graph, neighbor, visited)
# Example Usage
graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F']
}
visited = {node: False for node in graph}
DFS(graph, 'A', visited)
- Java Implementation:
import java.util.*;
class DFS {
void DFS(int vertex, boolean[] visited, ArrayList> graph) {
visited[vertex] = true;
for (int neighbor : graph.get(vertex)) {
if (!visited[neighbor]) {
DFS(neighbor, visited, graph);
}
}
}
public static void main(String[] args) {
ArrayList> graph = new ArrayList<>();
boolean[] visited = new boolean[graph.size()];
// Initialize the graph
// Perform DFS
}
}
- C/C++ Implementation:
#include
#include
#define MAX 1000
bool visited[MAX];
void DFS(int vertex, int graph[MAX][MAX], int vertices) {
visited[vertex] = true;
for (int i = 0; i < vertices; i++) {
if (graph[vertex][i] && !visited[i]) {
DFS(i, graph, vertices);
}
}
}
int main() {
// Initialize graph
// Perform DFS
return 0;
}
Time Complexity of DFS Implementation in Python
- Time Complexity: The time complexity of the Depth-First Search (DFS) algorithm in Python is O(V + E), where 'V' represent the number of vertices and 'E' represents the number of edges in the graph. The algorithm traverses through each vertex and examines its edges exactly once. This linear time complexity accounts for visiting all vertices and edges, making it efficient for graph traversal and searching.
- Space Complexity: The space complexity for DFS in Python is O(V), where 'V' stands for the number of vertices. This space complexity is due to the utilization of auxiliary data structures and the stack/recursion approach in DFS traversal, necessitating space proportional to the number of vertices.
Application of DFS
Depth-First Search (DFS) offers a broad spectrum of applications across different fields. It excels in various scenarios such as:
- Graph Traversal: DFS assists in traversing graphs and provides a basis for topological sorting algorithms.
- Cycle Detection: By identifying cycles within graphs, DFS is crucial in applications that require cycle detection, like deadlock detection in operating systems.
- Connected Components Identification: It is utilized to determine connected components in a graph, distinguishing separate subsets.
- Maze Generation: DFS is utilized to generate mazes efficiently.
- Puzzle Solving: In solving puzzles like mazes or crosswords, DFS can be a fundamental tool.
- Pathfinding Algorithms: Algorithms like the A* algorithm often use DFS to explore paths.
- Network Analysis: For analyzing and discovering structures within networks, DFS is widely applicable.
- Compiler Design: Compilers use DFS in syntax analysis, for instance, in code parsing and translation.
- Network-Related Tasks: In computer networks, DFS is used for tasks such as routing, broadcast, and searching.