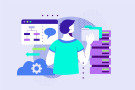
- 17th Nov 2023
- 22:15 pm
- Admin
R stands out as a robust programming language and environment, extensively employed for statistical computing and in-depth data analysis. R possesses a rich array of data structures, empowering users to organize and manipulate data efficiently. A profound comprehension of these structures is crucial for unleashing the full potential of R.
What are Data Structures in R Programming?
Data structures in R Programming are organizational formats for storing, managing, and manipulating data. They play a pivotal role in data analysis, providing a framework to represent different types of information. R offers a rich variety of built-in data structures, each tailored to handle specific data types and operations. These structures are essentially containers that enable the representation and manipulation of various types of information.
Data structures in R Programming not only store data but also facilitate seamless operations, making them instrumental in statistical computing and data analysis. Whether it's handling numeric values, character strings, factors, or complex multidimensional arrays, R's diverse set of data structures caters to the diverse needs of data scientists, statisticians, and analysts, providing a robust framework to extract insights from data in a systematic and coherent manner.
Vectors:
In R Programming, vectors play a foundational role, serving as one-dimensional arrays capable of storing elements of the same data type, be it numeric, character, or logical values. They are the simplest and most commonly used data structures in R, providing a compact way to store and operate on data.
- Numeric Vectors:
Numeric vectors, constructed with the c() function, are frequently employed in R to store numerical values efficiently. For example:
# Creating a numeric vector
numeric_vector <- c(1.5, 2.3, 3.7)
Numeric vectors facilitate numeric operations, making them essential for statistical computations. Element-wise operations, such as addition or multiplication, can be performed efficiently.
# Element-wise addition
result_vector <- numeric_vector + 2
This operation adds 2 to each element of the numeric vector.
- Character Vectors:
Character vectors in R store sequences of characters and can be generated using the c() function, providing a convenient way to handle textual data.
# Creating a character vector
character_vector <- c("apple", "banana", "orange")
Character vectors are valuable for handling textual data and categorical variables. They are frequently used in data manipulation and analysis.
- Logical Vectors:
Logical vectors are used to represent Boolean values (TRUE or FALSE). They find application in conditions and logical operations. Creating a logical vector is similar:
# Creating a logical vector
logical_vector <- c(TRUE, FALSE, TRUE)
Logical vectors are crucial for control flow and decision-making in programming.
Understanding vectors is foundational as they form the basis for more complex data structures in R. Proficiency in vector operations ensures efficient and concise code.
Lists
Lists in R serve as flexible and dynamic data structures capable of accommodating elements of varying data types. Unlike vectors, lists allow for a mix of numeric, character, and other types within a single structure. This flexibility makes lists suitable for handling complex data where elements may have diverse attributes.
- Creating Lists:
Lists are created using the list() function:
# Creating a list
my_list <- list(name = "John", age = 30, is_student = FALSE)
In this example, the list contains a character element ("John"), a numeric element (30), and a logical element (FALSE).
- Accessing List Elements:
List elements can be accessed using indices or names:
# Accessing list elements
name_element <- my_list[[1]]
age_element <- my_list[["age"]]
This flexibility makes lists suitable for storing complex data structures where elements have different attributes.
- List Operations:
Lists support various operations, such as appending elements or combining lists:
# Appending an element to a list
my_list <- c(my_list, city = "New York")
# Combining two lists
combined_list <- c(my_list, list(language = "R"))
Lists are widely used in scenarios where data is heterogeneous and may not fit into a uniform structure.
Understanding lists enhances the ability to manage and manipulate diverse data types effectively.
Dataframes
Dataframes in R function as tabular structures, similar to spreadsheets or SQL tables, and are adept at handling structured data with rows and columns. They prove invaluable in data analysis, offering a convenient format for performing statistical operations on datasets.
- Creating Dataframes:
Dataframes are often created using the data.frame() function:
# Creating a dataframe
student_data <- data.frame(
name = c("Alice", "Bob", "Charlie"),
age = c(25, 22, 23),
grade = c("A", "B", "A-")
)
In this example, the dataframe contains columns for "name," "age," and "grade."
- Accessing Dataframe Elements:
Accessing elements within a dataframe can be achieved using either column indices or column names:
# Accessing dataframe elements
first_student_name <- student_data[[1, "name"]]
grade_column <- student_data$grade
Dataframes are extensively utilized in the realms of data analysis and statistical modeling, owing to their tabular structure.
- Dataframe Operations:
Dataframes support various operations, including filtering, subsetting, and merging:
# Filtering dataframe based on age
young_students <- student_data[student_data$age < 25, ]
# Subsetting dataframe columns
selected_columns <- student_data[, c("name", "age")]
# Merging two dataframes
combined_data <- merge(student_data, other_data, by = "name")
Dataframes are indispensable for handling structured datasets in R, and proficiency in dataframe operations is essential for data scientists.
Matrices
R matrices are akin to two-dimensional arrays, serving as a means to store data of the same type. Conceptually, they can be envisioned as an arrangement of vectors in a grid. Matrices in R are particularly useful for numerical computations, frequently finding application in fields such as linear algebra and statistical modeling.
- Creating Matrices:
Matrices are created using the matrix() function:
# Creating a matrix
my_matrix <- matrix(c(1, 2, 3, 4, 5, 6), nrow = 2, ncol = 3)
In this example, the matrix has 2 rows and 3 columns.
Accessing Matrix Elements:
Matrix elements can be accessed using row and column indices:
# Accessing matrix elements
element_21 <- my_matrix[2, 1]
Matrices are efficient for numerical computations, especially in linear algebra and statistical modeling.
- Matrix Operations:
Matrices support various mathematical operations, such as addition, multiplication, and transposition:
# Matrix addition
sum_matrix <- my_matrix + my_matrix
# Matrix multiplication
product_matrix <- my_matrix %*% t(my_matrix)
Understanding matrix operations is essential for advanced statistical analysis and modeling in R.
Arrays
Arrays in R are versatile multi-dimensional structures capable of holding data of the same type. Unlike matrices, which are strictly two-dimensional, arrays in R can have more than two dimensions, providing additional flexibility in organizing and analyzing complex datasets.
- Creating Arrays:
Arrays are created using the array() function:
# Creating a 3D array
my_array <- array(c(1, 2, 3, 4, 5, 6, 7, 8, 9), dim = c(3, 3, 1))
In this example, the array has dimensions 3x3x1.
- Accessing Array Elements:
Array elements are accessed using indices corresponding to each dimension:
# Accessing array elements
element_123 <- my_array[1, 2, 3]
Arrays are powerful for handling complex data with higher-dimensional attributes.
- Array Operations:
Arrays support operations similar to matrices but extended to multiple dimensions:
# Array addition
sum_array <- my_array + my_array
# Array multiplication
product_array <- my_array * my_array
Understanding arrays is crucial for applications involving voluminous and multidimensional datasets.
Factors
Factors are used to represent categorical data in R. They are an essential data structure when dealing with variables that have distinct categories or levels. Factors provide a meaningful way to encode and analyze categorical variables, contributing to efficient statistical modeling.
- Creating Factors
Factors are created using the factor() function:
# Creating a factor
gender_factor <- factor(c("Male", "Female", "Male", "Female"))
In this example, the factor represents the gender of individuals.
- Accessing Factor Levels:
Factor levels can be accessed using the levels() function:
# Accessing factor levels
gender_levels <- levels(gender_factor)
Factors are integral for statistical modeling and analysis, especially in scenarios where categorical variables play a crucial role.
- Factor Operations:
Factors support operations like recoding levels or checking the frequency of each level:
# Recoding factor levels
revised_gender_factor <- factor(gender_factor, levels = c("Male", "Female", "Other"))
# Checking frequency of each level
level_counts <- table(gender_factor)
Understanding factors is essential for working with categorical data effectively in R.
Applications of Data Structures in R Programming
- Exploratory Data Analysis (EDA): Data structures in R are indispensable for EDA tasks, enabling researchers to organize and analyze data efficiently. For example, vectors and lists can be used to store and manipulate variables, while dataframes are ideal for tabular summaries.
- Statistical Modeling: Factors in R hold significant importance for representing categorical variables in statistical modeling. Matrices and arrays are commonly employed in structuring data for various statistical techniques, including regression analysis, analysis of variance (ANOVA), and similar modeling methodologies.
- Data Visualization: Different data structures offer diverse opportunities for data visualization. Vectors and matrices can be used in plotting, while dataframes provide a structured format for creating informative visualizations.
- Machine Learning: Data structures are foundational in machine learning tasks. Lists and arrays, for instance, can be used to structure input features and labels. Dataframes are often employed to organize training and testing datasets.
- Data Cleaning and Transformation: When dealing with messy datasets, dataframes are instrumental in cleaning and transforming data. Functions like dplyr and tidyr leverage dataframes for seamless data manipulation.
Blog Author Profile - Radhika Joshi
Radhika Joshi is a seasoned programming expert with a profound academic background in Computer Science and Machine Learning. Her dedication to the field has been fueled by her relentless pursuit of knowledge and her commitment to pushing the boundaries of technology. PhD in Computer Science from a prestigious university in the United States. Her doctoral research focused on cutting-edge advancements in advanced machine learning algorithms and techniques.