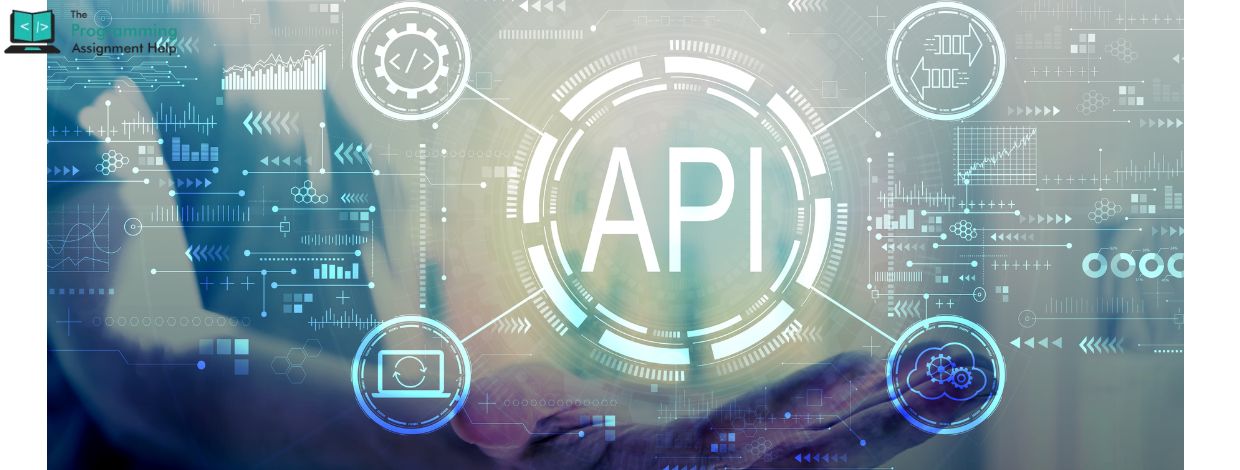
- 12th Jul 2024
- 18:01 pm
- Adan Salman
In this network setup, implement a custom ping application where Host1 acts as the client and Host2 as the server. Host1 sends 5 UDP packets with custom data to Host2, which responds accordingly, without relying on ICMP protocol. You need to create a ping application using the custom packets with the following specifications:
- Host1 (101) is the client and Host2 (102) is the server.
- Host1 (101) will send 5 ping messages to the server using the UDP protocol and custom packets with the packet type as request (pick a number for this type).
- The data in the custom packet will be ‘This is assignment 5!’
- At each hop, the packet will be decapsulated, recreated with the specific field types and sent to the next hop
- A packet from Host1 when reaches to Host2, Host2 finds its own ID in the DST field and therefore should create a new packet with the response type (pick a number for this type) without changing the sequence number
- You will not use ICMP protocol
Custom Packets and APIs - 16:332:423/544 - Get Assignment Solution
Please note that this is a sample assignment solved by our Python Programmers. These solutions are intended to be used for research and reference purposes only. If you can learn any concepts by going through the reports and code, then our Python Tutors would be very happy.
- Option 1 - To download the complete solution along with Code, Report and screenshots - Please visit our Programming Assignment Sample Solution page
- Option 2 - Reach out to our Python Tutors to get online tutoring related to this assignment and get your doubts cleared
- Option 3 - You can check the partial solution for this assignment in this blog below
Free Assignment Solution - Custom Packets and APIs - 16:332:423/544
from mininet.node import CPULimitedHost
from mininet.topo import Topo
from mininet.net import Mininet
from mininet.log import setLogLevel, info
from mininet.node import RemoteController
from mininet.cli import CLI
from mininet.link import TCLink
import socket
class Simple3PktSwitch(Topo):
"""Simple topology example."""
def __init__(self, **opts):
"""Create custom topo."""
# Initialize topology
super(Simple3PktSwitch, self).__init__(**opts)
#Topo.__init__(self)
# Add hosts and switches
h1 = self.addHost('h1')
h2 = self.addHost('h2')
h3 = self.addHost('h3')
opts = dict(protocols='OpenFlow13')
# Adding the Router
s1 = self.addRouter('R1', dpid="0000000000000001")
s2 = self.addRouter('R1', dpid="0000000000000002")
s3 = self.addRouter('R1', dpid="0000000000000003")
# Add links
self.addLink(h1, R1)
self.addLink(h2, R2)
self.addLink(h3, R3)
def get_Host1_IP():
try:
Host1 = socket.gethostname()
host_ip = socket.gethostbyname(host_name)
print("Hostname : ",host_name)
print("IP : ",192.168.1.1)
except:
print("Unable to get Hostname and IP")
def get_Host2_IP():
try:
Host2 = socket.gethostname()
host_ip = socket.gethostbyname(host_name)
print("Hostname : ",host_name)
print("IP : ",192.168.2.1)
except:
print("Unable to get Hostname and IP")
def get_Host3_IP():
try:
Host3 = socket.gethostname()
host_ip = socket.gethostbyname(host_name)
print("Hostname : ",host_name)
print("IP : ",192.168.3.1)
except:
print("Unable to get Hostname and IP")
def installStaticFlows(net):
for sw in net.Router:
info('Adding flows to %s...' % sw.name)
Router.dpctl('add-flow', 'in_port=1,actions=output=2')
Router.dpctl('add-flow', 'in_port=2,actions=output=1')
info(Router.dpctl('dump-flows'))
# Driver code
get_Host_name_IP() #Function call
def run():
c = RemoteController('c', '0.0.0.0', 6633)
net = Mininet(topo=Simple3PktSwitch(), host=CPULimitedHost, controller=None)
net.addController(c)
net.start()
# installStaticFlows( net )
CLI(net)
net.stop()
# if the script is run directly (sudo custom/optical.py):
if __name__ == '__main__':
setLogLevel('info')
run()
Get the best Custom Packets and APIs - 16:332:423/544 assignment help and tutoring services from our experts now!
About The Author - Dr. Rohan Desai
Dr. Rohan Desai is an expert in network protocols and distributed systems, specializing in custom network applications and communication efficiency. With a focus on UDP, TCP/IP, and real-time data transmission, Dr. Desai brings practical insights into advanced networking concepts. Passionate about education, he guides students through hands-on assignments, bridging theory and practice, and fostering a deeper understanding of network topologies and protocol customization.