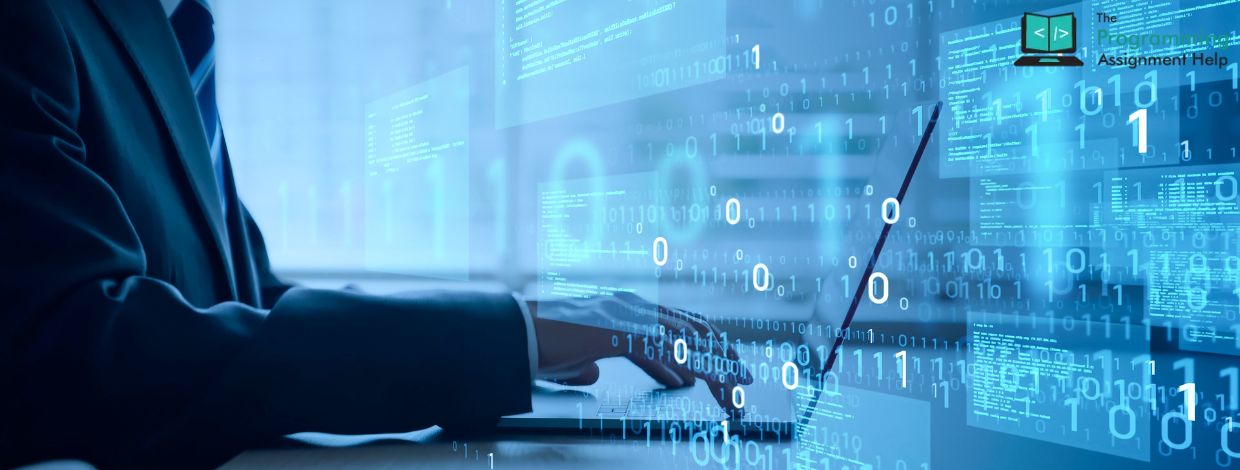
- 6th Jul 2024
- 20:05 pm
- Admin
In this project, you will use the authlog.txt file to create a Python script with a GUI front end. The script will allow users to input a date range and retrieve logon attempts within that range. The extracted date, time, IP address, and username will be displayed in a scrollable box for easy review. Use the authlog.txt file and prepare a Python script which does the following:
- Using the authlog.txt file provided here please prepare a GUI front end that will allow a user to enter a beginning date into a input box and an ending date into an input box.
- Using the two dates entered write a Python script that opens the file andretrieves all the logon attempts within the date range provided.
- Extract the following from each line: date, time, IP address and username. These are to be placed into a scroll box so that the user can scroll forward and back to review the data.
CSCI 1523 - Programming - Get Assignment Solution
Please note that this is a sample assignment solved by our Python Programmers. These solutions are intended to be used for research and reference purposes only. If you can learn any concepts by going through the reports and code, then our Python Tutors would be very happy.
- Option 1 - To download the complete solution along with Code, Report and screenshots - Please visit our Programming Assignment Sample Solution page
- Option 2 - Reach out to our Python Tutors to get online tutoring related to this assignment and get your doubts cleared
- Option 3 - You can check the partial solution for this assignment in this blog below
Free Project Solution - CSCI 1523 Programming Task
#import all the necessarry modules
from datetime import datetime
import re
# import tkinter module
from tkinter import *
from tkinter.ttk import *
from tkinter import messagebox
# declaring the regex pattern for IP addresses
pattern = re.compile(r'(\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3})')
#define a function which will call when we click the submit button
def submit():
#convert start date and end date in datetime
try:
#convert string date into datetime object
start = start_var.get()
start = datetime. strptime(start,'%b %d')
end = end_var.get()
end = datetime. strptime(end,'%b %d'
except Exception:
messagebox.showinfo("showinfo", "Enter valid dates")
return
login_entry.delete('1.0', END)
try:
file = open("1650798400409_authlog_(1).txt","r")#open the file in reading mode
line = file.readline()#read the first line
while(line):#repeat until end of line
result = ""
temp = line.split()
date = temp[0] + " " +temp[1] + " " +temp[2] #extract date and time
file_date = datetime. strptime(date,'%b %d %H:%M:%S')#convert string date into datetime objecr
if start <= file_date and file_date <= end:#if date is in the range
result = date
try:#extract ip address and add it to the result
result += " " + pattern.search(line)[0]
except Exception:
pass
#extract username from the line
if "user" in line:
userTemp = line[line.find("user"):]
if "user=" not in userTemp:
result += " " + (userTemp[5:(userTemp.find(" ",5))])
result += "\n"
login_entry.insert(END, result)#add the result to the end of text widget
line = file.readline()
except Exception as ex:
messagebox.showinfo("showinfo", ex)
win = Tk()
#define variable to get the strat date and end date
start_var = StringVar()
end_var= StringVar()
#define all the label
head_label = Label(win, text = 'Csci 1523 - Search authkog.txt file', font=('calibre',10, 'bold'))
start_label = Label(win, text = 'Start Date: ', font=('calibre',10, 'bold'))
end_label = Label(win, text = 'End Date: ', font=('calibre',10, 'bold'))
login_label = Label(win, text = 'Login Attempts ', font=('calibre',10, 'bold'))
#define all the entry
start_entry = Entry(win,textvariable = start_var, font=('calibre',10,'normal'))
end_entry = Entry(win,textvariable = end_var, font=('calibre',10,'normal'))
login_entry = Text(win, height = 5, width = 32)
# creating a button using the widget Button to call the submit function
sub_btn=Button(win, text = 'Search', command = submit)
#grid everything to the tkinter oject
head_label.grid(row = 0,column = 1,padx = 2,pady=4)
start_label.grid(row=1,column=0,padx = 2,pady=4)
end_label.grid(row=2,column=0,padx = 2,pady=4)
start_entry.grid(row=1,column=1,padx = 2,pady=4)
end_entry.grid(row=2,column=1,padx = 2,pady=4)
sub_btn.grid(row = 1,column = 3,rowspan = 2,padx = 2,pady=4)
login_label.grid(row = 3,column = 1,padx = 2,pady=4)
login_entry.grid(row = 4,column = 1,padx = 2,pady=4)
win.mainloop()#start the loop
Get the best CSCI 1523 - Programming project help and tutoring services from our experts now!
About The Author - Rekha Seth
Rekha Seth is a seasoned software developer with extensive experience in Python scripting and GUI design. Specializing in data extraction and user interface creation, Rekha has developed a user-friendly application for processing and visualizing logon attempt data. Her expertise ensures a seamless experience, enabling users to easily enter date ranges, extract relevant log information, and review it efficiently.