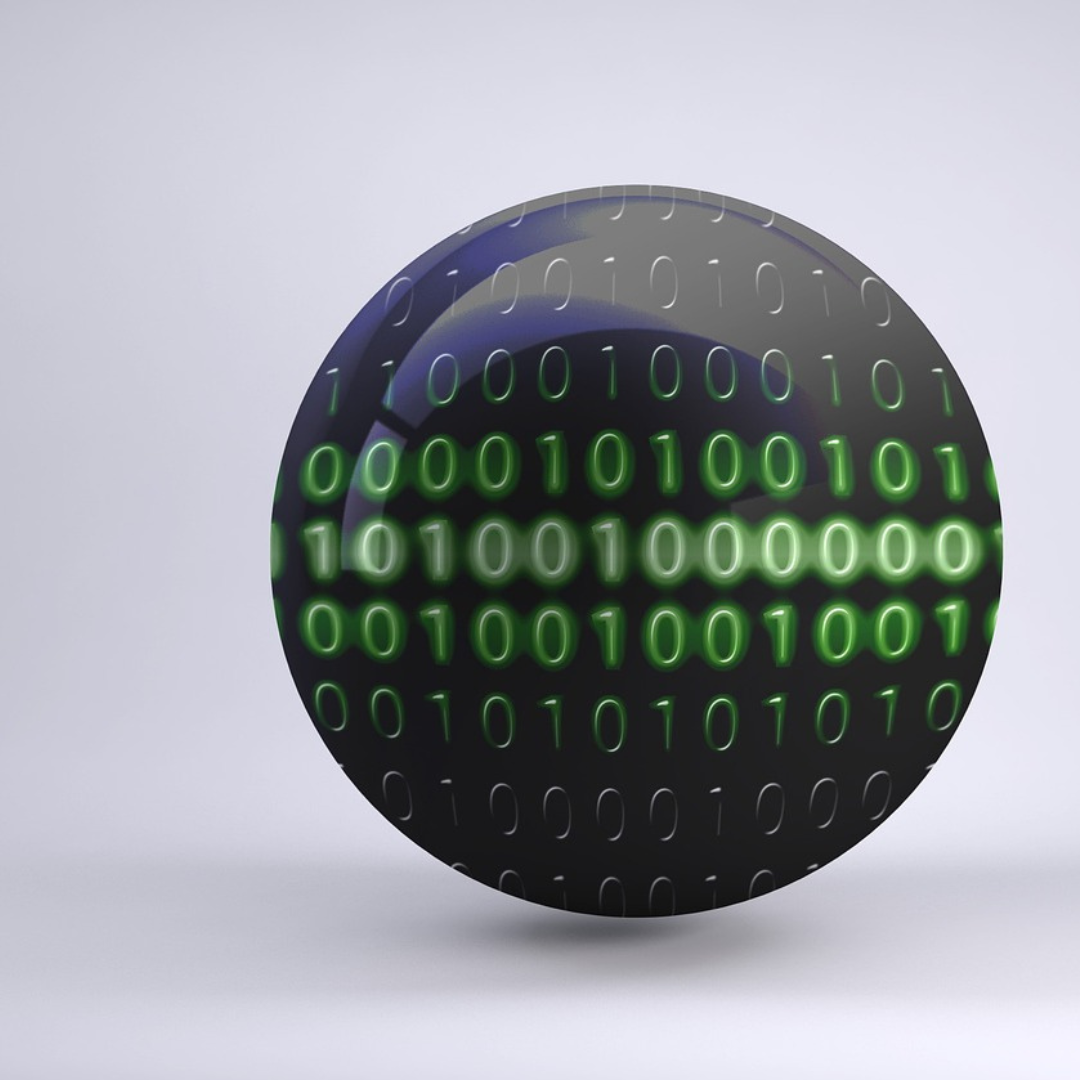
- 3rd Apr 2023
- 09:38 am
Java Homework Help
Java Homework Question
Creating Sphere Class (Java OOP, Classes, Objects, Math class, Methods) - Design and implement a class called Sphere that contains instance data that represents the sphere's diameter. Define the Sphere constructor to accept and initialize the diameter, and include getter and setter methods for the diameter. Include methods that calculate and return the volume and surface area of the sphere. Create a driver class called MultiSphere, whose main method instantiates and updates several Sphere objects. Volume = (4/3) * Pi * r(to the power of 3) Surface = 4 * Pi * r(to the power of 2)
Java Homework Solution
Here's the Java code for the Sphere class that contains instance data for the sphere's diameter, and methods to calculate its volume and surface area:
public class Sphere {
private double diameter;
public Sphere(double diameter) {
this.diameter = diameter;
}
public double getDiameter() {
return diameter;
}
public void setDiameter(double diameter) {
this.diameter = diameter;
}
public double getVolume() {
double radius = diameter / 2;
return (4.0 / 3.0) * Math.PI * Math.pow(radius, 3);
}
public double getSurfaceArea() {
double radius = diameter / 2;
return 4 * Math.PI * Math.pow(radius, 2);
}
}
This class has a constructor that accepts and initializes the diameter, and getter and setter methods for the diameter. It also includes methods to calculate the volume and surface area of the sphere using the formulas given in the problem statement.
Here's the Java code for the MultiSphere class that instantiates and updates several Sphere objects:
public class MultiSphere {
public static void main(String[] args) {
Sphere sphere1 = new Sphere(4.0);
Sphere sphere2 = new Sphere(6.0);
System.out.printf("Sphere 1: diameter = %.1f, volume = %.4f, surface area = %.4f\n",
sphere1.getDiameter(), sphere1.getVolume(), sphere1.getSurfaceArea());
System.out.printf("Sphere 2: diameter = %.1f, volume = %.4f, surface area = %.4f\n",
sphere2.getDiameter(), sphere2.getVolume(), sphere2.getSurfaceArea());
sphere1.setDiameter(8.0);
sphere2.setDiameter(10.0);
System.out.printf("Sphere 1 (updated): diameter = %.1f, volume = %.4f, surface area = %.4f\n",
sphere1.getDiameter(), sphere1.getVolume(), sphere1.getSurfaceArea());
System.out.printf("Sphere 2 (updated): diameter = %.1f, volume = %.4f, surface area = %.4f\n",
sphere2.getDiameter(), sphere2.getVolume(), sphere2.getSurfaceArea());
}
}
In the MultiSphere class, we create two Sphere objects with different diameters and print their initial volumes and surface areas. We then update the diameters of the spheres and print their new volumes and surface areas.
When you run this code, you should see output similar to the following:
Sphere 1: diameter = 4.0, volume = 33.5103, surface area = 50.2655
Sphere 2: diameter = 6.0, volume = 113.0973, surface area = 113.0973
Sphere 1 (updated): diameter = 8.0, volume = 268.0826, surface area = 201.0619
Sphere 2 (updated): diameter = 10.0, volume = 523.5988, surface area = 314.1593
Note that we used the Math.pow() method to calculate the power of a number and the Math.PI constant to represent the value of π.