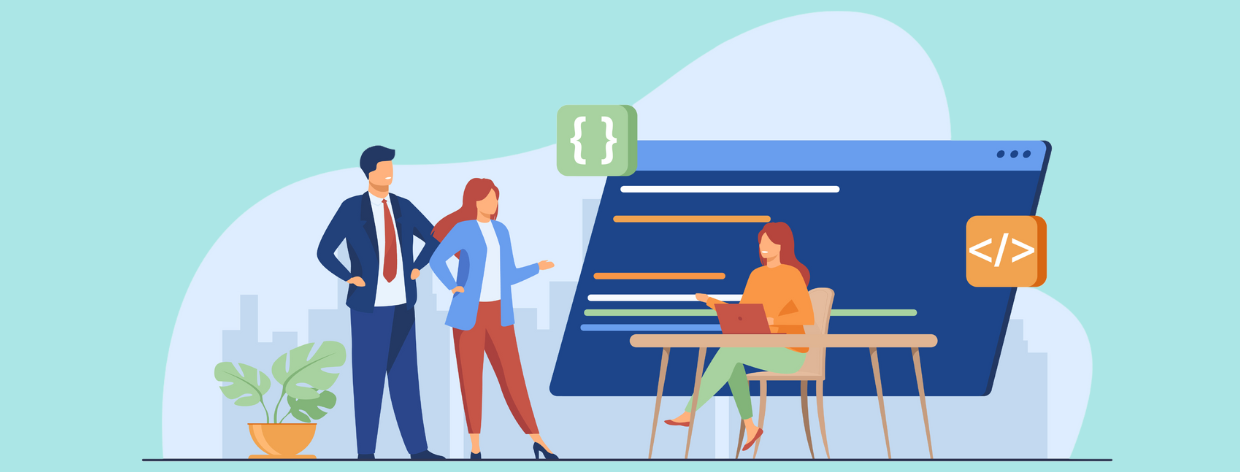
- 19th Feb 2024
- 16:11 pm
- Adan Salman
Data manipulation forms the backbone of countless Python applications. Whether you're analyzing scientific facts or developing websites, learning how to wrangle, manage, and change data is going to give you the power to gain insights and produce visuals that sell your message. Dictionary, with its flexible key-value pair, brings into focus a basic structure for storing and organizing data. However, when the need becomes more detailed—such as in deeper analysis or visualization—DataFrames, being the champions of data exploration in Python, come to the fore.
Think of a dictionary as a collection of unique items labeled with specific keys. Each item could represent a customer purchase, a sensor reading, or a social media post. While valuable individually, analyzing them in isolation can be challenging. DataFrames, in contrast, function like well-organized tables. Each row represents an item, and each column represents a specific attribute associated with it. Suddenly, comparing prices, identifying trends, or visualizing product popularity becomes significantly easier.
Converting dictionaries to DataFrames unlocks a treasure trove of analytical and visualization capabilities:
- Statistical analysis: Calculate averages, correlations, and other statistics across groups or the entire dataset, uncovering hidden patterns and trends.
- Data exploration: Filter, sort, and group data based on specific criteria, gaining deeper insights into relationships and anomalies.
- Seamless visualization: Leverage powerful libraries to create informative charts and graphs, transforming numbers into compelling narratives.
- Machine learning integration: Prepare your data for model training by extracting features, handling missing values, and ensuring compatibility with popular libraries.
This transformation empowers you to ask questions, discover answers, and tell captivating stories through your data. Understanding the different conversion methods available in Python will equip you to choose the most efficient and appropriate approach for your specific needs.
Want to master data manipulation in Python and unlock the power of DataFrames? Get personalized guidance and support with Python Assignment Help, Python Homework Help, and Python Tutoring Help.
Core Methods for Converting Dictionaries to DataFrames
In data exploration and analysis, Python's pandas library stands as a champion, empowering you to transform raw data into meaningful insights. But often, data resides in less structured formats like dictionaries, demanding transformation before unleashing its full potential. This is where the art of converting dictionaries to DataFrames, the workhorses of pandas, comes into play. Let's delve into the various methods at your disposal, emphasizing clarity, technical accuracy, and ethical considerations:
1. pandas.DataFrame.from_dict():
Method and Syntax: pd.DataFrame.from_dict(data, orient='columns', dtype=None, columns=None)
Explanation: This versatile method offers a streamlined approach for dictionary-to-DataFrame conversion. It accepts a dictionary data as input, where keys become column names and values become rows. The optional orient parameter lets you customize how keys are handled ('columns' is the default), while dtype and columns provide control over data types and custom column names, respectively.
Example: Simple dictionary with custom data types:
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 28]}
df = pd.DataFrame.from_dict(data, dtype={'age': int, 'name': str})
print(df)
Key Considerations:
- Duplicate keys raise errors by default. Use orient='index' or drop_duplicates=True to handle them responsibly.
- This method might create unexpected results with deeply nested structures. Evaluate alternative approaches for complex scenarios.
2. pandas DataFrame Constructor:
Syntax: pd.DataFrame(data, index=None, columns=None)
Explanation: This method empowers you to construct DataFrames directly from dictionaries, offering more flexibility than from_dict. By passing your dictionary to the constructor, you can explicitly define index and columns parameters to customize the DataFrame structure.
Examples:
Using dictionary keys as index: Leverage existing keys for indexing:
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 28]}
df = pd.DataFrame(data, index=data['name'])
Key Considerations:
- This method requires a clear understanding of your desired DataFrame structure.
- It can become cumbersome for complex dictionary structures or large datasets. Consider efficiency and maintainability, especially in academic or professional contexts.
3. Looping Through the Dictionary:
Explanation: While less common, you can iterate through the dictionary manually, creating lists of values and using them to construct the DataFrame.
Example:
data = {'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 28]}
names = data['name']
ages = data['age']
df = pd.DataFrame({'Name': names, 'Age': ages})
Key Considerations:
- It's prone to errors, especially with complex data structures, and can quickly become difficult to maintain for larger projects.
- Consider ethical implications of using less efficient methods when working on academic or professional projects. Prioritize responsible resource management and code clarity.
4. Alternative Libraries:
NumPy: Offers array-based DataFrames that can be converted to pandas DataFrames for specific use cases. For example, if your data is already stored in NumPy arrays, conversion to pandas DataFrames might be unnecessary depending on your analysis needs.
Dask: For handling extremely large datasets, Dask DataFrames leverage parallel processing for efficient conversion and analysis. Consider its suitability for resource-intensive tasks while adhering to ethical data handling practices.
Example (NumPy):
import numpy as np
data = {'x': np.array([1, 2, 3]), 'y': np.array([4, 5, 6])}
df = pd.DataFrame(data) # Convert NumPy array to DataFrame
Remember: The optimal method for converting dictionaries to DataFrames depends on your specific needs and data characteristics. Consider the following factors:
Dictionary structure: Simple, nested, or complex?
- Desired DataFrame structure: Row and column indexing, custom names, data types?
- Data size and complexity: Are you working with small, medium, or large datasets?
- Efficiency and scalability: How important is processing speed and memory usage?
- Ethical considerations: Are you working in an academic or professional setting with resource constraints?
By carefully evaluating these factors, you can choose the most appropriate method for your specific situation. As you delve deeper into data analysis and exploration, remember to use these powerful tools responsibly and ethically, contributing to a positive and sustainable data science ecosystem.
Advanced Techniques of Converting Dictionaries to DataFrames
- Missing Values: Missing data is a reality. Address it before conversion to avoid errors or biased analysis. Use fillna() in pandas.DataFrame.from_dict() to impute missing values with specific strategies, like filling with means or medians. For complex scenarios, consider custom functions within from_dict() to tailor missing value handling to your specific needs.
- Nested Secrets: Nested dictionaries require careful handling. Utilize tools like pd.json_normalize() to flatten nested structures into DataFrames. Alternatively, define custom recursive functions to traverse and extract data from complex nesting arrangements. Remember to maintain clarity and efficiency when dealing with intricate structures.
- Custom Transformations: Need specific data transformations during conversion? Leverage custom functions within from_dict() or the DataFrame constructor. These functions can perform calculations, data cleaning, or feature engineering, enriching your DataFrame with valuable insights from the get-go.
- Data Selection: Don't convert the entire dictionary if you only need a subset. Employ indexing and selection techniques like .loc and .iloc to extract specific rows, columns, or conditions based on your analysis needs. This optimizes performance and memory usage, especially when dealing with large datasets.
Choosing the Right Method for Converting Dictionaries to DataFrames
In the realm of Python data analysis, efficiently converting dictionaries into pandas DataFrames is a fundamental skill. While multiple methods exist, selecting the most suitable one requires careful consideration. Each approach possesses distinct strengths and weaknesses, influencing both the efficiency and effectiveness of the conversion process.
Understanding the Conversion Methods:
- pandas.DataFrame.from_dict(): This versatile method excels in flexibility. It readily handles simple dictionaries, accommodates basic nested structures, and allows customization of data types and column names. However, its control over complex DataFrame structure is limited.
- pandas DataFrame constructor: When precise control over the resulting DataFrame's structure is needed, the constructor shines. It empowers you to define specific indexing schemes for both rows and columns, ensuring your DataFrame aligns perfectly with your analysis needs. However, this method necessitates a clear understanding of the desired structure, and complex dictionaries can quickly become cumbersome.
- Looping through the dictionary: While less common, manually iterating through the dictionary offers maximum customization. You can implement intricate transformations before constructing the DataFrame, tailoring it to your specific requirements. However, tread carefully. This approach is often inefficient for large datasets, prone to errors, and can be difficult to maintain, especially in larger projects.
Guiding Your Method Selection:
- Dictionary Complexity: Are you dealing with straightforward key-value structures, or do you delve into the labyrinth of nested dictionaries? For simple scenarios, both from_dict() and the constructor are suitable. If nesting becomes intricate, from_dict() with orient='index' can handle basic cases, while custom functions or pd.json_normalize() might be required for more complex situations.
- Desired DataFrame Structure: Do you envision a basic table-like structure, or do you require specific column names and data types? from_dict() offers flexibility with its optional parameters, while both the constructor and from_dict() empower full customization. If you have specific indexing schemes in mind, the constructor grants you fine-grained control over both rows and columns.
- Performance Considerations: Efficiency becomes paramount when dealing with large datasets. For smaller data volumes, all methods are viable. As data size grows, prioritize efficiency. Generally, from_dict() outperforms the constructor, and looping should be avoided due to its inherent inefficiencies. Consider Dask DataFrames for extreme data sizes and explore potential parallelization options if performance becomes critical.
Applications of Converting Dictionaries to DataFrames
Converting dictionaries to DataFrames in Python unlocks a treasure trove of possibilities beyond mere data storage. With their structured format and powerful functionalities, DataFrames empower you to explore, analyze, and utilize data across various domains. Let's delve into the exciting applications:
Data Analysis and Exploration:
- Grouping, Aggregation, and Statistical Analysis: DataFrames excel at organizing data. Group data by specific attributes, calculate aggregate measures like mean, median, or standard deviation, and uncover hidden patterns. Imagine comparing sales across regions or analyzing customer demographics.
- Filtering, Sorting, and Summary Statistics: Isolate relevant data subsets using powerful filtering and sorting mechanisms. Calculate summary statistics like counts, percentiles, or quartiles to gain quick insights. Picture filtering user interactions by device type or calculating transaction averages over time.
- Data Visualization: Tell compelling stories with data! Create stunning visualizations using libraries like Matplotlib or Seaborn. Bar charts, line graphs, histograms, and more help you visualize trends, correlations, and distributions. Imagine plotting customer sentiment scores or visualizing product performance across categories.
Machine Learning and Data Science:
- Feature Engineering and Model Training: Feature engineering, or crafting meaningful features from raw data, forms the backbone of machine learning. DataFrames make this process efficient. Extract relevant features, transform them using scaling or encoding techniques, and prepare your data for modeling. Picture creating numerical features from text reviews or encoding categorical variables for classification tasks.
- Integration with Scikit-learn: Popular machine learning libraries like Scikit-learn seamlessly integrate with DataFrames. Load your prepared data directly into models like decision trees or linear regression algorithms. Train and evaluate models effectively, leveraging the rich functionality of both frameworks. Imagine predicting customer churn based on historical behavior or building sentiment analysis models using text data.
Web Development and APIs:
- Data Processing and Representation: APIs and web applications often return data in JSON format, which can be readily loaded into DataFrames for further manipulation and analysis. Clean, filter, and transform this data to suit your needs. Imagine analyzing API responses to understand user behavior or processing log data to identify website performance issues.
- Building Dashboards and Interactive Reports: Leverage libraries like Plotly or Bokeh to create interactive dashboards and reports directly from DataFrames. Allow users to filter, explore, and visualize data dynamically. Picture building real-time sales dashboards or interactive customer segmentation reports.