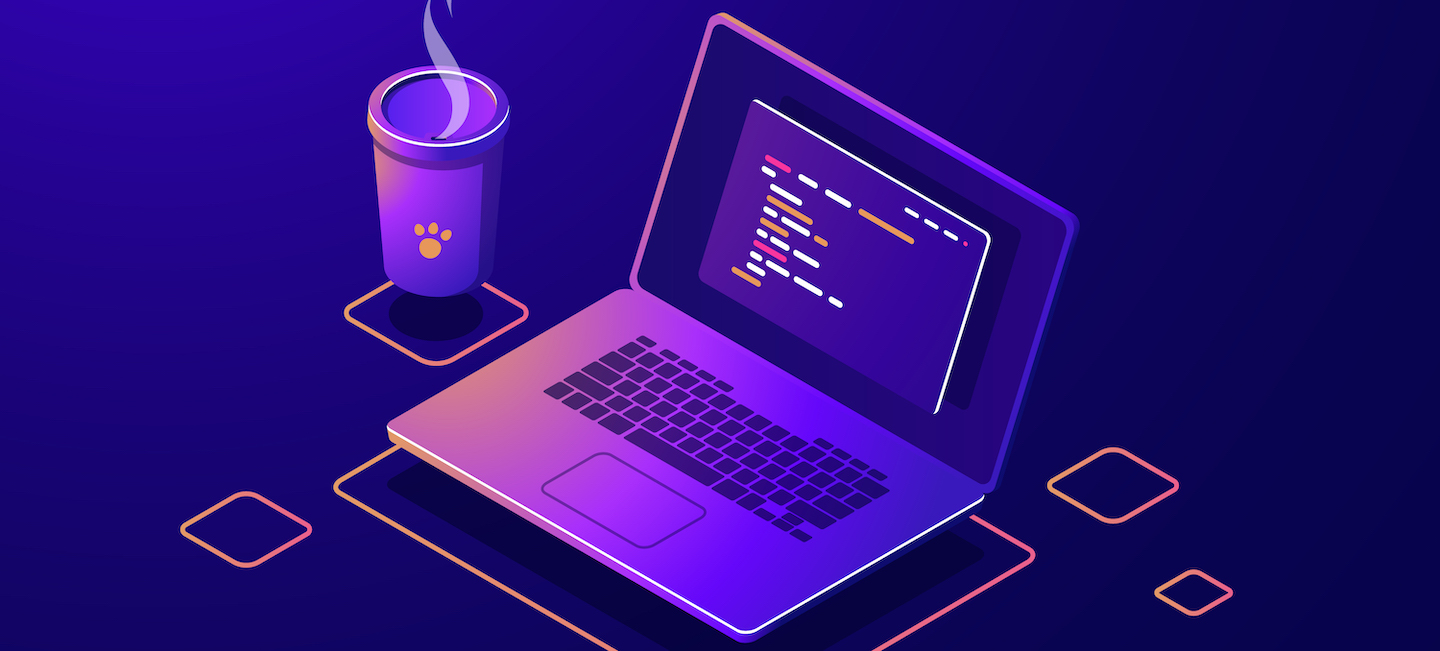
- 18th Dec 2023
- 14:38 pm
In Python, changing a hex representation to a string means turning the hex values into regular letters. Python makes this easy with 'bytes.fromhex()', creating a bytes thing from a hex string. Once we have this bytes thing, we can turn it into a string using 'decode()'.
Here, 'bytes.fromhex(hex_string)' turns the hex string into a bytes thing. 'decode('utf-8')' then changes this bytes thing into a string. This is super useful, especially when dealing with hex versions of text or binary data. People often use this in areas like encryption, networking, or managing files.
The following Python program snippet shows how to convert a hexadecimal string to a string:
def hex_to_string(hex_string):
# Convert hexadecimal string to bytes
hex_bytes = bytes.fromhex(hex_string)
# Decode bytes into a string using UTF-8 encoding
text_string = hex_bytes.decode('utf-8')
return text_string
# Example usage
hex_string_example = "48656c6c6f20576f726c64" # Hex representation of "Hello World"
resulting_string = hex_to_string(hex_string_example)
print("Original Hex String:", hex_string_example)
print("Converted String:", resulting_string)
In this example, the 'hex_to_string()' function gets a hex string, changes it to bytes, and then turns it into a string. The result is the text version of the original hex data.
To turn a hex string into a string in Python, we just change the hex values to regular letters, and that's it! This program's main methods are 'bytes.fromhex()' and 'decode()'.
1. Converting Hex to Bytes:
- It begins with a hex string like '"48656c6c6f20576f726c64"', representing the ASCII values for "Hello World."
- The 'bytes.fromhex(hex_string)' method takes this hex string and transforms it into a bytes thing. It does this by reading the string as pairs of hex values. So, for our example, it creates a bytes thing with the ASCII values for "Hello World."
2. Decoding Bytes to String:
- The bytes thing we get is turned into a string using 'decode('utf-8')'. We use 'utf-8' because it's a common way to show text in Unicode.
- With 'decode()', each byte is read as UTF-8 encoded text, turning every byte into its matching Unicode character. The string we get now shows the original hex data.
Example Usage:
The programme contains an example where the hexadecimal string '"48656c6c6f20576f726c64"' is provided to the 'hex_to_string()' method.
The function processes the input and returns the string "Hello World."
- General Applicability:
General Applicability program isn't just for the given example; you can use it for any hex string, including ASCII or binary data. It's super helpful, especially in areas like cryptography, networking, and dealing with files, where data often shows up in hex form.
- Modularity of Function:
The functionality is enclosed within the 'hex_to_string()' function, encouraging modularity and reusability. You can easily use this function in your projects to turn hex data into strings.
The program efficiently changes hex data to strings, making it handy for different applications where data is in hex form. The 'bytes.fromhex()' and 'decode()' methods work together to make sure hex data becomes its right string form.