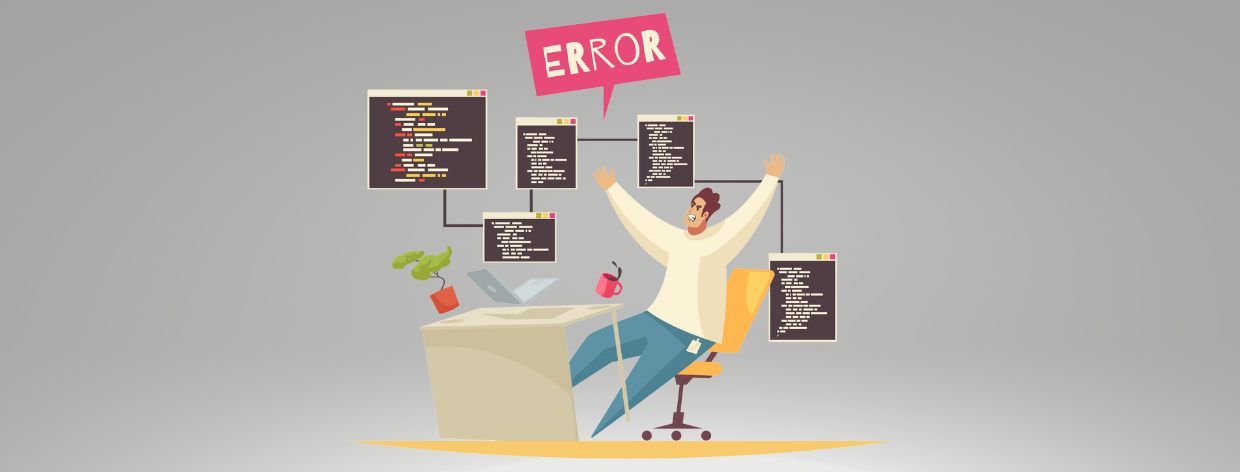
- 27th Feb 2024
- 23:02 pm
- Adan Salman
Python programming, like any other language, has certain specific rules that need to be followed for it to function correctly. These are syntax rules and have to be obeyed. Such kind of error acts as a roadblock to your code until you fix it and see your code running. Even though you face such errors, they are learning experiences that solidify in you the understanding of Python's basic structure.
However, encountering syntax errors is not enough. It needs to understand and take corrective effective actions for making the code to work. Otherwise, even the finest written program in Python shall not get executed.
Syntax error identification and troubleshooting are some of the first key things you need to know as a budding Python developer. In this way, it will guide you so that you can be able to confront mistakes and solve them, pushing yourself deeper into the professional world of programming. But understanding this will demystify those mistakes and turn them from inhibitors into stepping stones on the path to successful Python mastery.
Conquer common syntax errors in Python with ease! Master error identification, correction, and prevention strategies. Our experts offer comprehensive Python Assignment Help and Python Homework Help. Get started today and write error-free Python code!
Understanding Syntax Errors
Within the realm of Python programming, understanding and resolving syntax errors is crucial for ensuring smooth code execution. These errors, distinct from runtime errors that arise during program execution, act as gatekeepers, preventing code from running due to violations of the language's grammatical rules.
- Defining Syntax Errors:
In simple terms, syntax errors are general grammatical inconsistencies in your Python code that will lead to an obstruction of the capability of comprehensive interpretation and execution. They usually occur when an individual is bending the normal basic set-in-stone rules of Python, such as using incorrect punctuation, leaving out important keywords, or carrying on with improper indentation. They essentially act as the language's guardians, ensuring code adheres to its established structure.
- Differentiating from Runtime Errors:
It's vital to distinguish between syntax errors and runtime errors. Unlike syntax errors, which are detected during the parsing phase before code execution, runtime errors emerge during the actual execution of the program. These errors often arise due to logical flaws within the code, such as attempting to divide by zero or using an undefined variable.
- The Role of the Python Interpreter:
The Python interpreter is very good at finding and reporting syntax errors. It goes about its task of program interrogation line by line, looking for structural mismatches that will conflict with the syntax of the language. If it discovers such an error, the interpreter stops execution and issues a message that refers directly to the place in your program where it located the particular error. These messages are helpful tools for the programmer, which enable them to mend a breach in a jiff without much further ado.
This will give you a layman's guide in understanding what makes syntax errors different, how to tell them apart from runtime errors, and the role of the Python interpreter in pinpointing them.
Common Syntax Errors and Solutions
Syntax errors, the challenge of any aspiring programmer's existence, are obstacles encountered when your Python code deviates from the language's strict grammatical rules. Unlike runtime errors that emerge during program execution, syntax errors act as gatekeepers, preventing your code from even running. However, mastering the identification and rectification of these errors empowers you to navigate the world of Python with confidence. This guide delves into the most common syntax errors encountered by beginners, providing solutions and equipping you with the knowledge to overcome them effectively.
1. Indentation Errors:
Unlike most other programming languages, which make use of curly braces ({}), consistent indentation is key to how Python defines its code blocks: for functions, loop bodies, and conditional statements. Just one whiff of a bad space can throw a tab error, so perfection is necessary in your indentation.
Here are some common indentation errors and their solutions:
- Inconsistent indentation:
Ensure all lines within a code block are indented by the same number of spaces. Mixing tabs and spaces, or using different levels of indentation within the same block, are common mistakes.
# Incorrect: Inconsistent indentation
if x > 0:
print("Positive")
else:
print("Non-positive")
Solution:
# Correct: Consistent indentation
if x > 0:
print("Positive")
else:
print("Non-positive")
- Missing indentation:
Always indent the lines following a statement that introduces a code block, such as if, else, for, or while.
# Incorrect: Missing indentation
if x > 0:
print("Positive") # Incorrect, missing indentation
Solution:
# Correct: Proper indentation
if x > 0:
print("Positive")
- Mixing tabs and spaces:
While both spaces and tabs are technically valid for indentation, using a mixture of both can lead to unexpected errors due to inconsistent interpretation by different editors and environments. Always choose one and stick to it throughout your code.
2. Missing Colons (:)
Colons (:) serve as crucial indicators in various Python statements, signifying the start of a code block or the end of a label statement. Neglecting a colon in these contexts results in syntax errors.
Here are two common scenarios where missing colons cause errors:
- Missing colon after conditional statements:
The colon following an if, elif, or else statement marks the beginning of the code to be executed if the condition is true.
# Incorrect: Missing colon after if statement
if x > 0
print("Positive")
Solution:
# Correct: Colon after if statement
if x > 0:
print("Positive")
- Missing colon in for loop:
The colon following the for keyword introduces the code block to be executed for each iteration of the loop.
# Incorrect: Missing colon in for loop
for i in range(5):
print(i) # Incorrect, missing colon
Solution:
# Correct: Colon in for loop
for i in range(5):
print(i)
3. Mismatched Parentheses, Braces, and Quotes
Parentheses (), square brackets [], and curly braces {} are used for two reasons: the grouping of the expression while writing a function and to define data structures—that is, lists and dictionaries. It is quite essential in a Python program to ensure the open and closed characters are written properly and balanced.
Here are some examples of mismatched characters and their fixes:
- Mismatched parentheses:
Ensure each opening parenthesis has a corresponding closing parenthesis at the same nesting level.
# Incorrect: Mismatched parentheses
print("Hello" (world)) # Extra closing parenthesis
Solution:
# Correct: Balanced parentheses
print("Hello", world)
- Mismatched quotes:
Single quotes (') and double quotes (") can be used interchangeably for strings in Python, but mixing them within the same string is invalid.
# Incorrect: Mismatched quotes
name = "John's Book" # Mixing single and double quotes
Solution:
# Correct: Consistent quotes
name = "John's Book" # Double quotes used consistently
4. Undefined Variables
Variables in Python store data and can be referenced throughout your code. Before referencing a variable, it must be declared and assigned a value. Using an undeclared variable results in a syntax error.
Here's an example of an undefined variable error:
# Incorrect: Using an undefined variable
print(name) # Name is not defined yet
Solution:
# Correct: Variable declaration and assignment
name = "Alice"
print(name)
5. Syntax Errors with Special Characters
Special characters, including operators (+, -, *, /), punctuation marks (!, @, #, $, %, ^, &, *, (, ), [, ], {, }, |, ;, :, ', "), and whitespace, play specific roles in Python syntax. Using them incorrectly can lead to syntax errors.
Here are some examples of incorrect special character usage and their proper application:
- Extra spaces:
While Python allows for spaces around operators and keywords, excessive spaces within strings or variable names can cause unexpected errors.
# Incorrect: Extra spaces
message = " Hello, world! " # Extra spaces around the comma
Solution:
# Correct: Proper spacing
message = "Hello, world!"
- Incorrect use of escape sequences:
Python utilizes backslashes (\) as escape characters to represent special characters within strings. Forgetting the backslash or using an incorrect escape sequence can lead to errors.
# Incorrect: Missing backslash for a newline
print("This is line 1\nThis is line 2") # Missing backslash before n
Solution:
# Correct: Using escape sequence for newline
print("This is line 1\nThis is line 2")
By understanding these common syntax errors and their solutions, you equip yourself with essential knowledge to navigate the world of Python code with confidence. Remember, practice and consistent attention to detail are key to mastering error identification and correction, ultimately leading to smoother and more efficient Python programming experiences.
Debugging Tips and Strategies:
Syntax errors, though temporary roadblocks are inevitable encounters in the world of Python programming. However, by employing effective debugging strategies, you can transform them into valuable learning opportunities and write error-free code. This guide unveils some essential techniques to empower you on this journey.
- The Interactive Python Interpreter:
Imagine a line-by-line code execution environment – that's the power of the interactive Python interpreter! Simply type Python in your terminal and start testing code snippets. This allows you to isolate problematic sections and pinpoint errors efficiently.
- Code Editor Features as Allies:
Modern editors have features like syntax highlighting, which separates different elements of code visually in such a way that possible mistakes are easily caught. They also contain built-in error checking for throwing a possible syntax error even before the user is through with typing.
- The Art of Using print Statements:
The print statement is your debugging companion. By strategically inserting print statements, you can observe the values of variables at different points in your code. This helps track data flow and identify unexpected changes or inconsistencies that might indicate errors.
- Deciphering Error Messages:
Python throws an error message when it encounters a syntax error. Do not panic! Although the messages at first may look cryptic, they actually contain the keys to fixing whatever is wrong. They typically point to the line number and nature of the problem. By carefully reading the message and focusing on the highlighted line, you can often pinpoint the exact location and type of the error.