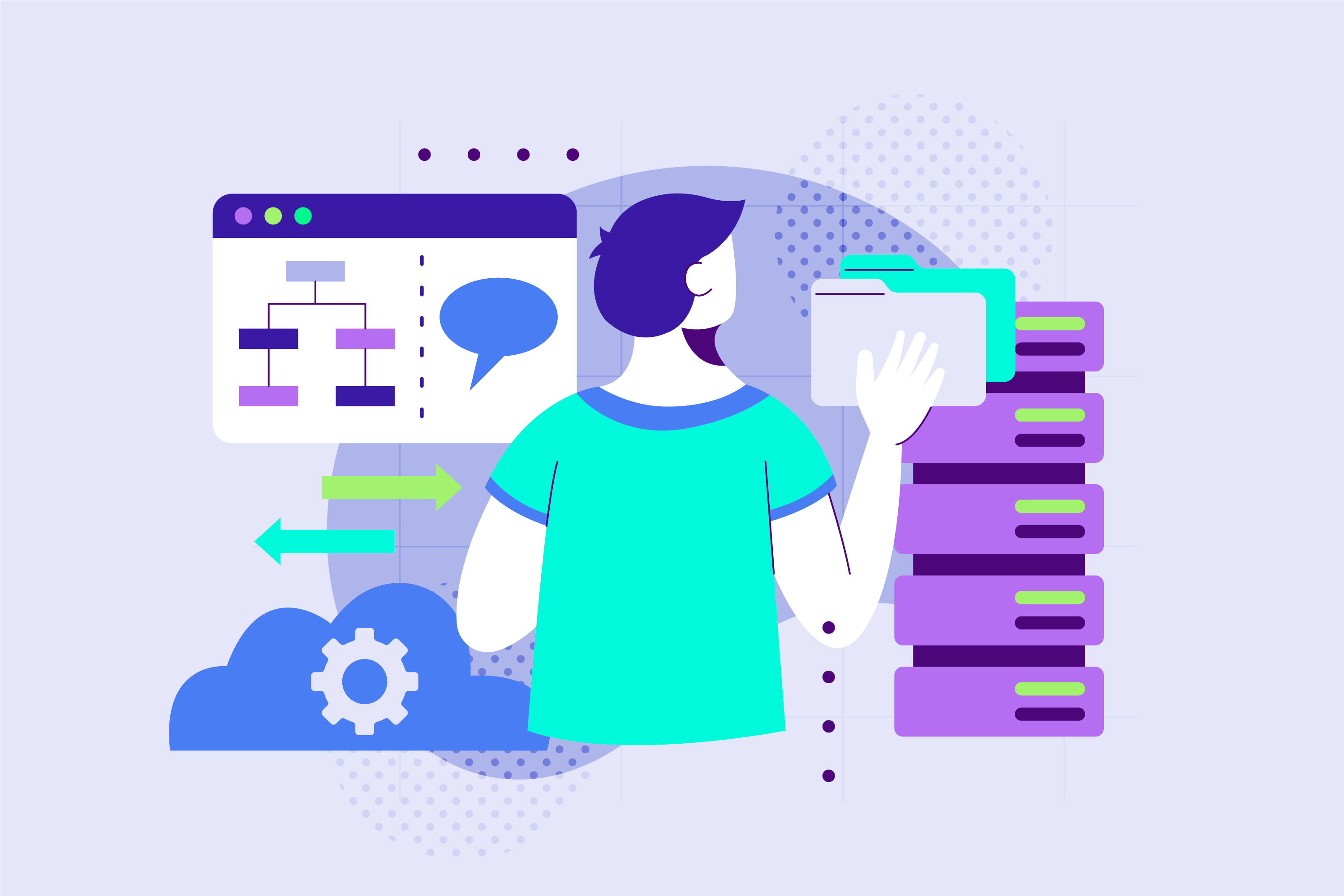
- 9th Feb 2024
- 14:28 pm
Comparing strings is fundamental in Python programming, from validating user input to searching data and performing text analysis. But it's more than just simple matches with equality operators. This guide delves into various comparison methods and highlights potential complexities like encoding, case sensitivity, and advanced pattern matching.
From basic lexical comparisons, where order reigns supreme, to substring checks using in and regular expressions for powerful pattern matching, you'll explore diverse tools for tackling different comparison needs. However, don't underestimate the intricacies! Case sensitivity can alter results, and encoding differences across platforms might lead to unexpected mismatches. Understanding these factors empowers you to write robust and versatile string comparison logic in Python.
Basic String Comparisons
In Python, strings are essential building blocks, and comparing them accurately is crucial for various tasks. Let's explore fundamental comparison methods, starting with exact and alphabetical matches:
Equality and Inequality:
- ==: This operator checks for identical content and order of characters. "Python" == "python" is False due to case sensitivity.
- !=: The opposite of equality, it's True when strings differ in any way. "apple" != "banana" is True.
Lexical Comparisons:
- <, >: These operators compare strings alphabetically, character by character. "dog" < "elephant" is True.
- <=, >=: Inclusive versions allowing equality. "hello" <= "hello" is True.
Substring Checks:
- in: Checks if a substring exists within another string. "ing" in "string" is True.
- not in: Returns the opposite, useful for filtering. "python" not in "apple" is True.
Challenges to Avoid:
- Leading/trailing whitespace: Watch out for spaces or tabs at the beginning or end, as they affect comparisons. Use strip() to remove them.
- Case sensitivity: By default, Python string comparisons are case-sensitive. Use lower() or upper() for case-insensitive checks.
Case Sensitivity Considerations
Python's default string comparisons are case-sensitive, meaning "Hello" and "hello" are distinct entities. But fear not! We have tools to tame this chameleon:
- lower() and upper(): These methods convert entire strings to lowercase or uppercase, enabling case-insensitive checks. For example, name.lower() == "johndoe" becomes true after conversion.
- casefold(): This Unicode-aware champion handles languages with complex character sets. It converts both uppercase and lowercase equivalents of characters to their base form, ensuring accurate comparisons across languages.
Handling Encodings and Unicode
Beyond case sensitivity, another hurdle awaits string encodings. Just like languages have different alphabets, strings can be stored in various ways depending on platforms and characters used. This can lead to unexpected mismatches if ignored.
- Enter encode() and decode(): these methods convert strings between different encodings. For example, text.encode('utf-8') ensures the string uses the familiar UTF-8 encoding.
The key is consistency! When comparing strings, make sure they share the same encoding. Otherwise, characters might appear different, causing errors. Imagine comparing "café" encoded in one system and "caf\xe9" in another - disaster!
Regular Expressions for Powerful Matches
While basic comparisons lay the foundation, string comparisons in Python truly soar with regular expressions (regex). Imagine searching for specific patterns within strings, extracting phone numbers from text, or validating email formats - regex makes it possible.
Building Regex Blocks:
- .: Matches any single character. Think of it as a wildcard.
- *: Matches zero or more repetitions of the preceding character. "c*at" matches "cat", "cattt", or even just "a".
- +: Matches one or more repetitions of the preceding character. "c+at" matches "cat" or "cattt", but not just "a".
- ^: Matches the beginning of the string. "^hello" only matches strings starting with "hello".
- $: Matches the end of the string. "world$" only matches strings ending with "world".
Common Use Cases:
- Email validation: Ensure email addresses follow the correct format with "[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$".
- Phone number extraction: Grab phone numbers with "\d{3}-\d{3}-\d{4}".
- Password strength checks: Use regex to enforce specific password complexity requirements.
Advanced Comparison Techniques:
While standard methods tackle precise matches, real-world data often holds imperfections. Enter fuzzy matching algorithms like the Levenshtein distance, measuring the "edit distance" - the number of changes needed to transform one string into another. Imagine comparing "teh cat" and "the cat" - just one edit!
Libraries like difflib offer handy tools like SequenceMatcher for calculating similarity or difference ratios. These come in handy for tasks like spell checking or finding similar text snippets.
Advanced Techniques:
- Soundex and Metaphone: Encode phonetically similar words based on pronunciation, useful for name matching or searching with typos.
- Token-based comparisons: Split strings into tokens (words or characters) and compare them individually, allowing for flexibility when dealing with variations in word order or spacing.