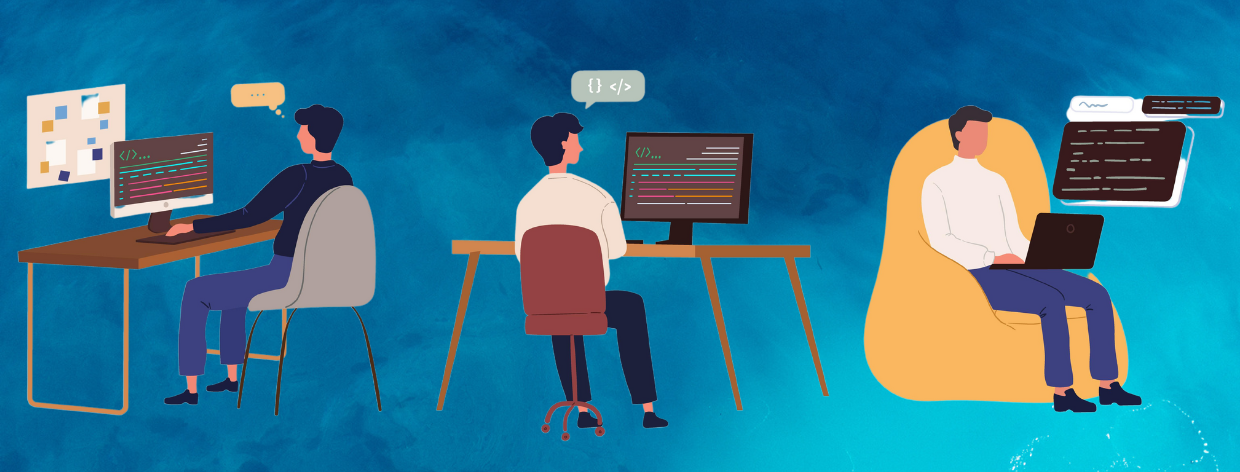
- 21st Feb 2024
- 14:56 pm
- Admin
In the world of Python programming, ensuring data fidelity through meticulous type verification is paramount. This article focuses on a specific yet vital aspect: verifying whether a string accurately represents an integer. Mastering this skill unlocks diverse applications across various domains, including:
- Data Analysis: Extracting numerical values from sensor readings, financial data, or user input often stored as strings necessitates reliable integer verification for accurate analysis.
- Input Validation: Preventing errors and ensuring data integrity becomes crucial when users provide input. Verifying if inputs like age or quantity are valid integers safeguards against downstream issues.
- Numerical Operations: Performing calculations on user-provided values or data parsed from text sources requires confidence in their integer representation to ensure accurate results.
Python offers an array of methods to tackle this verification challenge:
Built-in Functions:
- isinstance(string, int): Offers a straightforward approach by determining if the string belongs to the int class. While quick, it might miss edge cases like strings with leading/trailing spaces or decimals.
- string.isdigit(): Verifies if the string consists solely of digits, making it efficient for basic integer checks. However, it excludes negative signs and decimals.
Regular Expressions:
- Harnessing the power of the re module and regular expressions allows for crafting precise patterns to identify valid integer formats. This method excels at handling complex scenarios with negative numbers, decimals, or specific delimiters.
Error Handling:
- Implementing try-except blocks adds robustness to your code. This gracefully handles potential exceptions like ValueError that might arise during conversion attempts, preventing program crashes and providing informative error messages.
Selecting the optimal method hinges on several factors:
- Data Size: For massive datasets, vectorized operations in libraries like numpy prioritize efficiency.
- Complexity: Regular expressions excel at handling intricate formats and edge cases.
- Performance: Consider the trade-off between accuracy and speed based on your specific use case.
Master Check-String-is-Integer in Python and unlock accurate data analysis, robust programs, and efficient code. Dive into built-in methods, regular expressions, custom functions, and error handling with our expert Python Assignment Help or Python Homework Help. Level up your Python skills today!
Built-in Methods
In the vibrant world of Python programming, ensuring correct data types is crucial for accurate results and smooth program execution. This article explores two built-in methods for verifying if a string represents an integer: isinstance() and isdigit(). While they offer simplicity and efficiency, understanding their limitations is essential for informed decision-making.
1. isinstance():
Imagine you have a string variable and want to confirm if it belongs to the int data type. This is where isinstance() comes to the rescue. It takes two arguments: the object to be checked and the data type we're interested in. In this case, it would be:
is_integer = isinstance(string_variable, int)
If string_variable contains a valid integer representation, is_integer will be True. However, be mindful of the following:
Edge Cases:
- Empty strings: Even though technically strings, empty strings are not integers, and isinstance() will return False.
- Unicode characters: Characters outside the standard ASCII range might not be recognized as integers, even if they represent numerical digits.
Demonstration:
string_1 = "123"
string_2 = ""
string_3 = "???" # Arabic digits
print(isinstance(string_1, int)) # True
print(isinstance(string_2, int)) # False
print(isinstance(string_3, int)) # False
2. isdigit():
This method directly checks if a string consists only of digits (0-9). It's faster than isinstance() for straightforward integer checks but has its own limitations:
- Negative Numbers and Decimals: It considers strings like "-123" or "3.14" as non-integers, even though they represent valid numerical values.
- Locale-Specific Formatting: If your string uses a decimal separator other than a dot, like a comma in some locales, isdigit() might misinterpret it as a non-digit.
Demonstration:
string_1 = "123"
string_2 = "-123"
string_3 = "3.14"
print(string_1.isdigit()) # True
print(string_2.isdigit()) # False
print(string_3.isdigit()) # False
Limitations and Beyond:
While both methods offer quick checks, they have limitations. For more complex scenarios involving negative numbers, decimals, or locale-specific formatting, consider regular expressions or custom functions for tailored verification. Remember, choosing the right method depends on your specific needs and data characteristics.
Regular Expressions
While built-in methods offer convenience for basic integer verification, intricate scenarios demand more powerful tools. Enter the realm of regular expressions, where patterns reign supreme to match complex text structures. In this exploration, we'll harness this power to craft a regular expression for identifying valid integer strings in Python.
1. Regular Expressions:
Think of regular expressions as microscopic detectives, meticulously examining strings to identify specific patterns. They use special characters and symbols to define these patterns, enabling flexible and precise matching beyond simple character checks.
2. Crafting the Pattern:
A regular expression pattern for valid integer strings can look like ^[-+]?\d+$. This pattern matches the beginning of the string, optional negative signs, one or more digits, and the end of the string.
Let's dissect its components:
- ^: Matches the beginning of the string.
- [-+]?: Optional group (zero or one occurrence) matching either a hyphen - or a plus sign + for negative numbers.
- \d+: Matches one or more digits (0-9).
- $: Matches the end of the string.
3. re module:
We'll use the re module to work with regular expressions:
import re
string_1 = "123"
string_2 = "-456"
string_3 = "abc"
string_4 = "1.23"
is_integer_1 = re.match(pattern, string_1)
is_integer_2 = re.match(pattern, string_2)
is_integer_3 = re.match(pattern, string_3)
is_integer_4 = re.match(pattern, string_4)
print(is_integer_1) # True
print(is_integer_2) # True
print(is_integer_3) # None (doesn't match)
print(is_integer_4) # None (doesn't match)
As you can see, the regular expression correctly identifies both positive and negative integer strings but excludes non-numeric characters or decimals.
4. Advantages of Regular Expressions:
- Precise Control: You can tailor the pattern to match specific formats, including leading/trailing spaces, specific delimiters, or even a range of allowed digits.
- Handling Edge Cases: They excel at addressing complex scenarios like negative numbers, decimals with specific separators, or even scientific notation.
- Flexibility: You can adapt the pattern based on your evolving needs without changing the core verification logic.
5. Advanced Topics: Capturing Specifics
- Regular expressions can also capture specific parts of the matched string. Imagine you want to extract the actual integer value from a string like "+123". You can modify the pattern and use capture groups to achieve this:
pattern = r"(^[-+]?)(\d+)"
match = re.match(pattern, "+123")
if match:
sign, integer = match.groups()
print(f"Sign: {sign}, Integer: {integer}")
This opens up further possibilities for data extraction and manipulation based on your specific requirements.
Custom Functions and Advanced Techniques
While built-in methods and regular expressions offer powerful tools for verifying string integers, intricate scenarios often demand a more tailored approach. Enter custom functions, empowering you to craft a verification process that precisely aligns with your specific needs.
Crafting a Robust Custom Function:
Here's an example function incorporating multiple methods for robust verification:
def is_valid_integer(string):
"""
Verifies if a string represents a valid integer, handling various edge cases.
Args:
string: The string to be checked.
Returns:
True if the string represents a valid integer, False otherwise.
"""
# Check for empty string or non-string types
if not isinstance(string, str):
return False
# Remove leading/trailing whitespaces
string = string.strip()
# Check if the string consists only of digits and optional sign
if not string.isdigit() and not re.match(r"^[-+]?\d+$", string):
return False
# Handle edge cases like decimals, scientific notation, or locale-specific formatting
# using additional logic or regular expression patterns as needed
# If all checks pass, return True
return True
This function leverages isinstance(), isdigit(), and regular expressions for basic checks. The commented section highlights the opportunity to add custom logic for handling specific data formats or negative numbers based on your requirements.
Advanced Techniques and Benefits:
- Specific Data Formats: Craft intricate regular expression patterns to match specific delimiters, thousands separators, or allowed digit ranges.
- Negative Number Handling: Implement custom logic to differentiate between valid negative numbers and non-numeric characters like hyphens.
- Error Handling: Include try-except blocks to gracefully handle potential exceptions like ValueError during conversion attempts.
Custom functions offer several advantages:
- Flexibility: Adapt the verification process to your exact needs without relying on limitations of built-in methods.
- Control: Tailor the function to handle specific edge cases or data formats relevant to your use case.
- Readability: Break down complex verification logic into well-defined functions, enhancing code clarity and maintainability.
Error Handling in Python
Verifying string representations of integers is crucial for data integrity and accurate computations in Python. But what happens when unexpected input throws your program into disarray? This is where error handling becomes your guardian angel, preventing crashes and ensuring smooth execution.
Harnessing the try-except Block:
Imagine you have a function to convert strings to integers:
def string_to_int(string):
return int(string)
This works well for valid integers, but what if someone enters "abc"? It would raise a ValueError, potentially crashing your program. Here's how we can implement a try-except block to gracefully handle such situations:
def string_to_int(string):
try:
return int(string)
except ValueError:
print(f"Error: '{string}' cannot be converted to an integer.")
return None # Or handle differently based on your needs
Now, if an invalid input occurs, the program prints an informative error message and continues execution without crashing.
Applications of Check-String-is-Integer in Python
Verifying whether a string represents an integer is a foundational skill in Python, but its applications extend far beyond basic data type checks. Here's a glimpse into various scenarios where this skill shines:
- Data Analysis: Sensor readings, financial data, or user surveys often store numerical values as strings. Accurately converting these strings to integers, using robust verification techniques, is crucial for meaningful analysis and accurate insights.
- Input Validation: User-provided data requires scrutiny. Verifying if their age, quantity, or other numerical inputs are valid integers safeguards against downstream errors and ensures data integrity.
- Numerical Operations: Performing calculations on user-entered values or data parsed from text sources necessitates confidence in their integer representation. Verification prevents inaccurate results and potential program crashes.
- Data Cleaning and Filtering: Working with large datasets often involves filtering rows based on numerical criteria. Precise integer verification ensures relevant data is selected and irrelevant entries are excluded.
- String Parsing and Extraction: Extracting specific numerical values from complex strings, like dates or timestamps, requires identifying and verifying the integer portions embedded within them. String-to-integer verification becomes a key step in this process.
- Custom Data Formats: Handling proprietary data formats or specific delimiters often necessitates crafting tailored verification logic based on integer patterns. Mastering this skill empowers you to work with diverse data structures with confidence.
- Advanced Algorithms and Machine Learning: Many algorithms and machine learning models rely on numerical features. Verifying these features as integers ensures compatibility and prevents unexpected errors during training or prediction.