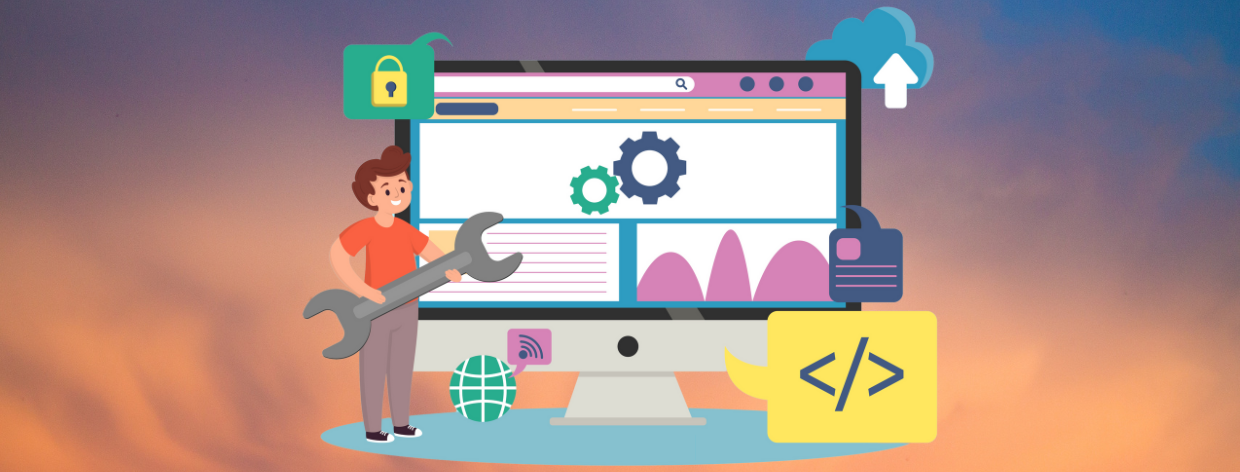
- 22nd Feb 2024
- 22:29 pm
Proper capitalization isn't just about following grammar rules; it's about clarity, consistency, and efficiency in both writing and computer-mediated communication. Python offers various methods to achieve this, enabling developers to manipulate the first letter of strings effectively.
This concept, known as "capitalize first letter," refers to the process of converting the initial character to uppercase while leaving the remaining text unchanged. However, its applicability extends beyond this basic functionality.
- Data Cleaning and Formatting: Imagine working with datasets containing inconsistencies in capitalization for names, titles, or other textual elements. Python's capitalization techniques provide a solution, ensuring uniformity and facilitating accurate data analysis.
- Natural Language Processing (NLP): In NLP tasks such as Named Entity Recognition, for example, one would want to pick out proper nouns (these are often found in capitalized form). Consistency of capitalization in any piece of text equally raises the efficiency and effectiveness of the models developed on it.
- User Input and Interaction: When dealing with user-generated content like names, addresses, or product titles, ensuring proper capitalization enhances user experience and maintains consistency.
- Text Processing Automation: Chatbots, automated responses, or even programmatic text generation can leverage capitalization techniques to produce consistent and professional communication, mirroring human writing styles.
- Code Readability and Maintainability: Capitalizing the first letter of function names, classes, or variables promotes better code readability and maintainability, facilitating understanding for both you and other developers.
By mastering these techniques, you gain the ability to manipulate text effectively within your Python projects. With our Python Assignment Help and Python Homework Help service, we ensures consistent, well-formatted data, unlocking valuable applications across various domains.
Methods for Capitalizing the First Letter
In Python, where text manipulation reigns supreme, mastering the art of capitalization unlocks diverse applications and streamlines your workflow. This article delves into various methods for capitalizing the first letter in Python, equipping you with the knowledge to choose the right tool for the job.
- Using str.capitalize():
This versatile method elevates the first character to uppercase while keeping the rest lowercase. Think of it as a gentle nudge towards proper form.
text = "hello world"
capitalized = text.capitalize()
print(capitalized)
# Output: Hello world
Remember, it only affects the first character and ignores non-alphabetic elements.
- Using str.title():
This method goes a step further, capitalizing the first letter of each word. Imagine transforming a sentence into a title case masterpiece.
text = "hello world, how are you?"
text.title()
# Output: 'Hello World, How Are You?'
Keep in mind it might capitalize unwanted words like articles or prepositions.
- Using string.capwords():
Though less common, this method behaves similar to str.title() but preserves original capitalization for acronyms and initialisms.
text = "NASA is a space agency"
capwords = string.capwords(text)
print(capwords)
# Output: Nasa Is A Space Agency
While valuable for specific use cases, its application might be limited compared to other methods.
- Using upper(), lower(), and title():
By wielding upper(), lower(), and title() strategically, you can achieve fine-grained control over capitalization.
text = "hello World!"
# Capitalize specific words
first_word_upper = text[:6].upper() + text[6:]
print(first_word_upper)
# Output: HELLO World!
# Convert to lowercase, then title case
all_lowercase = text.lower()
title_case = all_lowercase.title()
print(title_case)
# Output: Hello World!
- Using istitle() and isupper():
While not directly capitalizing letters, istitle() and isupper() act as sentries, verifying if your string adheres to title case or uppercase rules.
# Title case check
text1 = "This Is A Title"
print(text1.istitle())
# Output: True
# Uppercase check
text2 = "ALL UPPERCASE"
print(text2.isupper())
# Output: True
Remember, these methods report, not alter, leaving the capitalization responsibility to other techniques.
- Using String Slicing and upper():
For more control, this approach extracts the first character, capitalizes it, and combines it with the remaining string.
text = "hello world"
def capitalize_first(text):
return text[0].upper() + text[1:]
capitalized = capitalize_first(text)
print(capitalized)
# Output: Hello world
While versatile, it might require additional logic for complex scenarios.
- Using The capitalize() function:
This method typically capitalizes the first letter of each word, but its behavior can vary depending on implementation. It's generally recommended to use alternative methods for word-level capitalization.
def custom_capitalize(text):
# Customize logic here, e.g., lowercase all words, then capitalize first letter
words = text.lower().split()
capitalized_words = [word.capitalize() for word in words]
return " ".join(capitalized_words)
text = "hello world, how are you?"
custom_capitalized_text = custom_capitalize(text)
print(custom_capitalized_text)
# Output: Hello World, How Are You?
- Using title() Function:
This built-in function acts similarly to str.title(), capitalizing the first letter of each word, ensuring consistency for titles or proper nouns.
text = "this is a sentence"
titled = text.title()
print(titled)
# Output: This Is A Sentence
Remember, it might capitalize unwanted words like articles or prepositions.
Advanced Techniques for Capitalizing the First Letter in Python
Beyond the fundamental methods covered earlier, delve into these advanced techniques for tackling complex capitalization requirements in Python:
Regular Expressions for Granular Control:
Harness the power of regular expressions to target specific patterns within strings for precise capitalization adjustments. This approach empowers you to:
- Capitalize words based on specific criteria:
Identify and capitalize words starting with vowels, containing double consonants, or belonging to a defined list.
import re
text = "Hello, my name is Alice. I am a data scientist."
# Capitalize words starting with vowels
def capitalize_vowel_words(text):
return re.sub(r"\b[aeiouAEIOU]\w+", lambda match: match.group().capitalize(), text)
vowels_capitalized = capitalize_vowel_words(text)
print(vowels_capitalized)
# Output: Hello, My name Is Alice. I Am a data scientist.
- Handle mixed-case acronyms and initialisms:
Preserve existing capitalization within acronyms while capitalizing the first letter, ensuring accuracy and consistency.
text = "NASA stands for National Aeronautics and Space Administration."
def capitalize_mixed_case_acronyms(text):
return re.sub(r"\b([A-Z][a-z]+\s?)+", lambda match: match.group().title(), text)
acronyms_preserved = capitalize_mixed_case_acronyms(text)
print(acronyms_preserved) # Output: NASA Stands for National Aeronautics and Space Administration.
Remember, mastering regular expressions requires practice and consideration for potential edge cases.
Custom Functions for Tailored Solutions:
For highly specific needs or intricate edge cases, craft custom functions that encapsulate your desired capitalization logic. This grants you absolute control and flexibility.
def capitalize_excluding_articles(text):
articles = ["a", "an", "the"]
words = text.lower().split()
capitalized_words = [
word.capitalize() if word not in articles else word
for word in words
]
return " ".join(capitalized_words)
text = "The quick brown fox jumps over the lazy dog."
capitalized_no_articles = capitalize_excluding_articles(text)
print(capitalized_no_articles)
# Output: The Quick Brown Fox Jumps Over the Lazy Dog.
Remember, well-documented and modular custom functions enhance code maintainability and understanding.
Leveraging External Libraries:
Explore specialized libraries like nltk or spaCy offering advanced text processing capabilities, including tokenization, named entity recognition, and potential built-in capitalization functionalities.
import nltk
# Tokenize and capitalize named entities
text = "Barack Obama, the 44th president of the United States, delivered a speech."
tokens = nltk.word_tokenize(text)
named_entities = nltk.ne_chunk(tokens)
capitalized_entities = [
token.capitalize() if isinstance(token, nltk.Tree) else token
for token in named_entities
]
print(" ".join(capitalized_entities))
# Output: Barack Obama, the 44th President of the United States, Delivered a Speech.
Be mindful of library dependencies and potential learning curve associated with these tools.
Applications of Capitalizing the First Letter in Python
While often associated with proper grammar and readability, capitalizing the first letter in Python holds immense value beyond mere aesthetics. From streamlining data processing to enhancing text analysis, mastering this technique unlocks various practical applications:
- Data Cleaning and Standardization: Inconsistent capitalization within datasets can hinder analysis and machine learning tasks. Techniques like str.title() or custom functions ensure consistent title case, facilitating data cleaning and preparation.
- Text Analysis and Information Extraction: Capitalization cues can aid in identifying named entities like person names, locations, or organizations. Regular expressions or custom functions targeting capitalized words can improve information extraction accuracy.
- Natural Language Processing (NLP) Tasks: Consistent capitalization aids in tasks like sentiment analysis, where understanding sentence structure and emphasis is crucial. Capitalization-aware tokenization or custom preprocessing steps can enhance NLP model performance.
- User Interface Design and Interaction: Capitalizing input fields, labels, and error messages creates a visually consistent and user-friendly interface. Built-in functions like str.capitalize() or custom formatting logic can ensure proper capitalization in UI elements.
- Code Readability and Maintainability: Consistent capitalization within your code will improve readibility and understanding for both yourself and other developers. Following established style guides or creating your own guidelines ensures clarity and maintainability.
- Document Generation and Formatting: Creating professional and visually appealing documents often involves manipulating capitalization for various elements. Tools like str.title() or custom functions can be leveraged for Headers and Titles, Proper Nouns and Acronyms and Table Formatting.