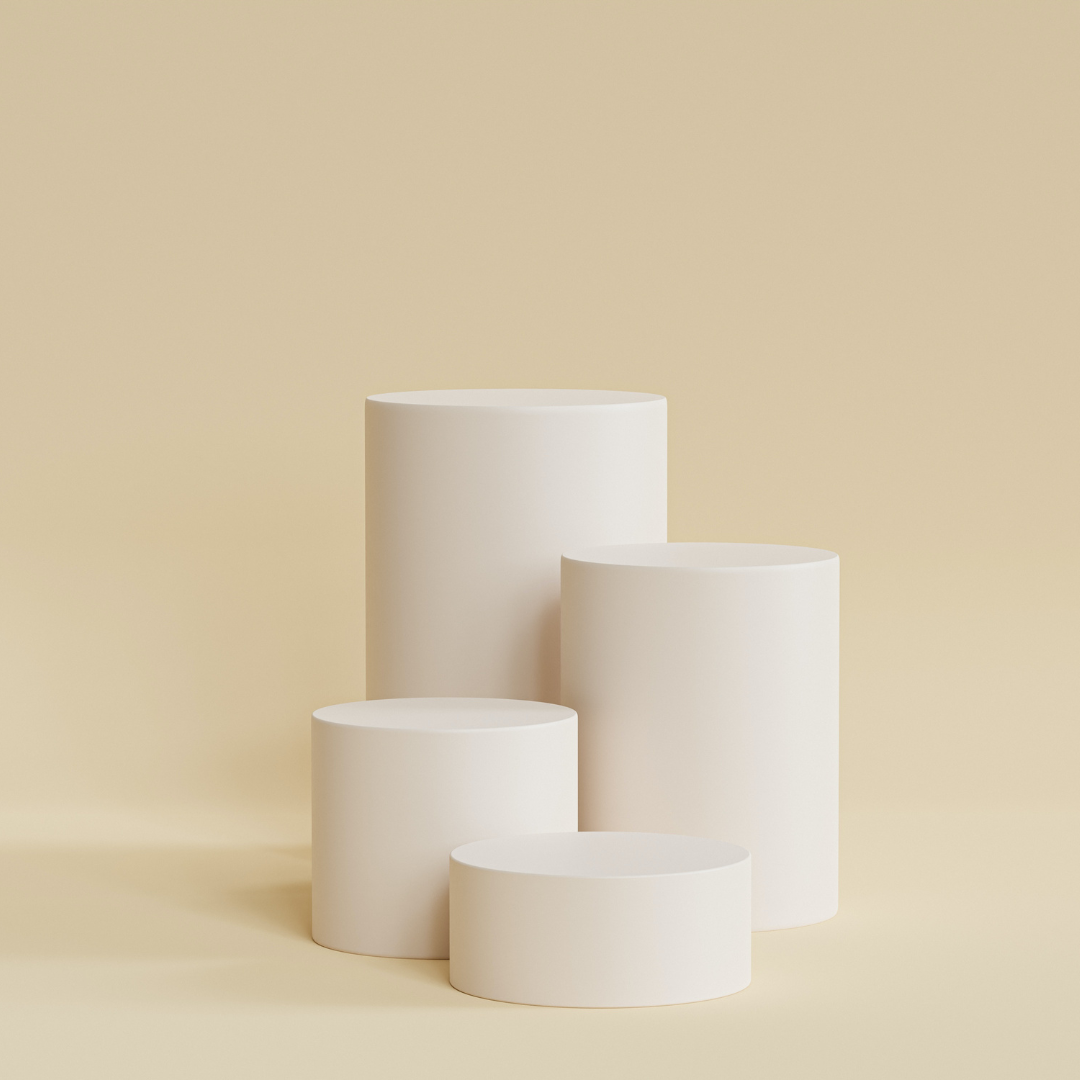
- 3rd Apr 2023
- 09:29 am
- Admin
Java Homework Question
Calculating Volume and Area of Cylinder (Java Math class) - Write an app that generates a random integer radius (r) and height (h) for a cylinder in the range 1 to 10, inclusive, and then computes the volume and surface area of the cylinder.
Java Homework Solution
Here's the Java code for the app that generates a random integer radius and height for a cylinder in the range 1 to 10 inclusive, and then computes the volume and surface area of the cylinder using the Math class:
import java.util.Random;
public class CylinderCalculator {
public static void main(String[] args) {
Random random = new Random();
// Generate random integers for the radius and height of the cylinder
int radius = random.nextInt(10) + 1;
int height = random.nextInt(10) + 1;
// Calculate the volume of the cylinder using the formula V = πr^2h
double volume = Math.PI * Math.pow(radius, 2) * height;
// Calculate the surface area of the cylinder using the formula A = 2πrh + 2πr^2
double surfaceArea = 2 * Math.PI * radius * height + 2 * Math.PI * Math.pow(radius, 2);
// Print the volume and surface area of the cylinder
System.out.printf("For a cylinder with radius %d and height %d:\n", radius, height);
System.out.printf("Volume: %.4f\n", volume);
System.out.printf("Surface area: %.4f\n", surfaceArea);
}
}
When you run this code, the program generates random integers for the radius and height of the cylinder in the range 1 to 10 inclusive using the Random class, calculates the volume and surface area of the cylinder using the Math class, and displays them to four decimal places. For example:
For a cylinder with radius 6 and height 8:
Volume: 904.7787
Surface area: 452.3893
Note that we used the Math.pow() method to calculate the power of a number and the Math.PI constant to represent the value of π.