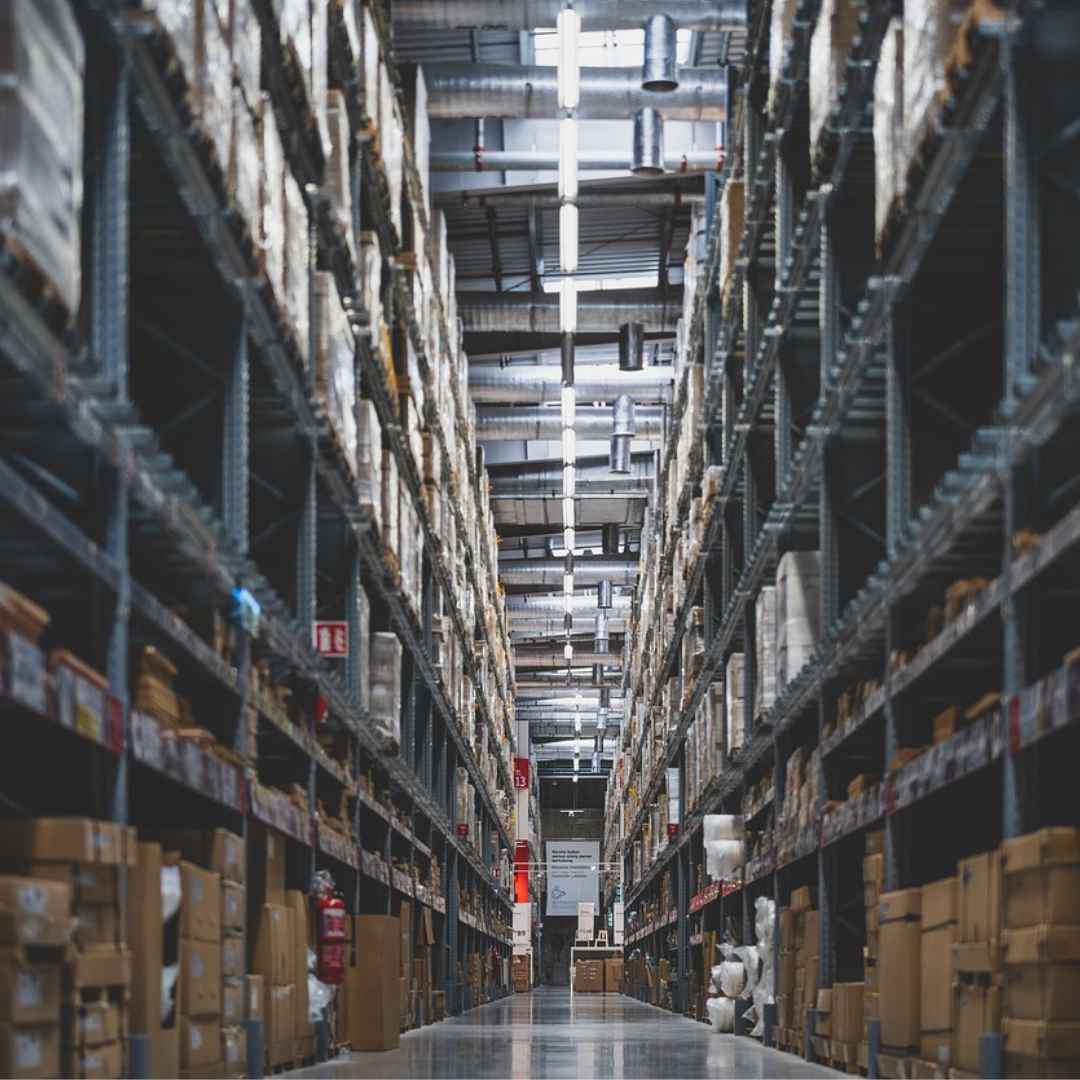
- 2nd Apr 2023
- 05:52 am
C++ Homework Help
C++ Homework Question
C++ Calculate Markup Cost With Functions - Write a program that asks the user to enter an item's wholesale cost and its markup percentage. It should then display the item's retail price. The program should have a function named calculate Retail that receives the wholesale cost and the markup percentage as arguments and returns the retail price of the item. Input Validation: Do not accept negative values for either the wholesale cost of the item or the markup percentage.
C++ Homework Solution
#include
#include
using namespace std;
// Function to calculate the retail price
double calculateRetail(double wholesaleCost, double markupPercentage) {
if (wholesaleCost < 0 || markupPercentage < 0) {
// Input validation: negative values are not allowed
return -1;
} else {
double markupAmount = wholesaleCost * (markupPercentage / 100);
double retailPrice = wholesaleCost + markupAmount;
return retailPrice;
}
}
int main() {
double wholesaleCost, markupPercentage, retailPrice;
// Ask the user for input
cout << "Enter the wholesale cost of the item: ";
cin >> wholesaleCost;
cout << "Enter the markup percentage: ";
cin >> markupPercentage;
// Calculate the retail price using the function
retailPrice = calculateRetail(wholesaleCost, markupPercentage);
if (retailPrice < 0) {
// Input validation failed, display an error message
cout << "Invalid input, please enter non-negative values." << endl;
} else {
// Display the retail price with two decimal places
cout << fixed << setprecision(2);
cout << "The retail price is $" << retailPrice << endl;
}
return 0;
}
This solution defines a function named calculateRetail() that takes in the wholesale cost and markup percentage as arguments, and returns the retail price of the item. Inside the function, we first perform input validation to make sure that the wholesale cost and markup percentage are not negative. If input validation passes, we calculate the markup amount and add it to the wholesale cost to get the retail price.
In the main function, we ask the user for the wholesale cost and markup percentage, and call the calculateRetail() function to get the retail price. If input validation fails inside the function, we display an error message. Otherwise, we display the retail price with two decimal places using fixed and setprecision().