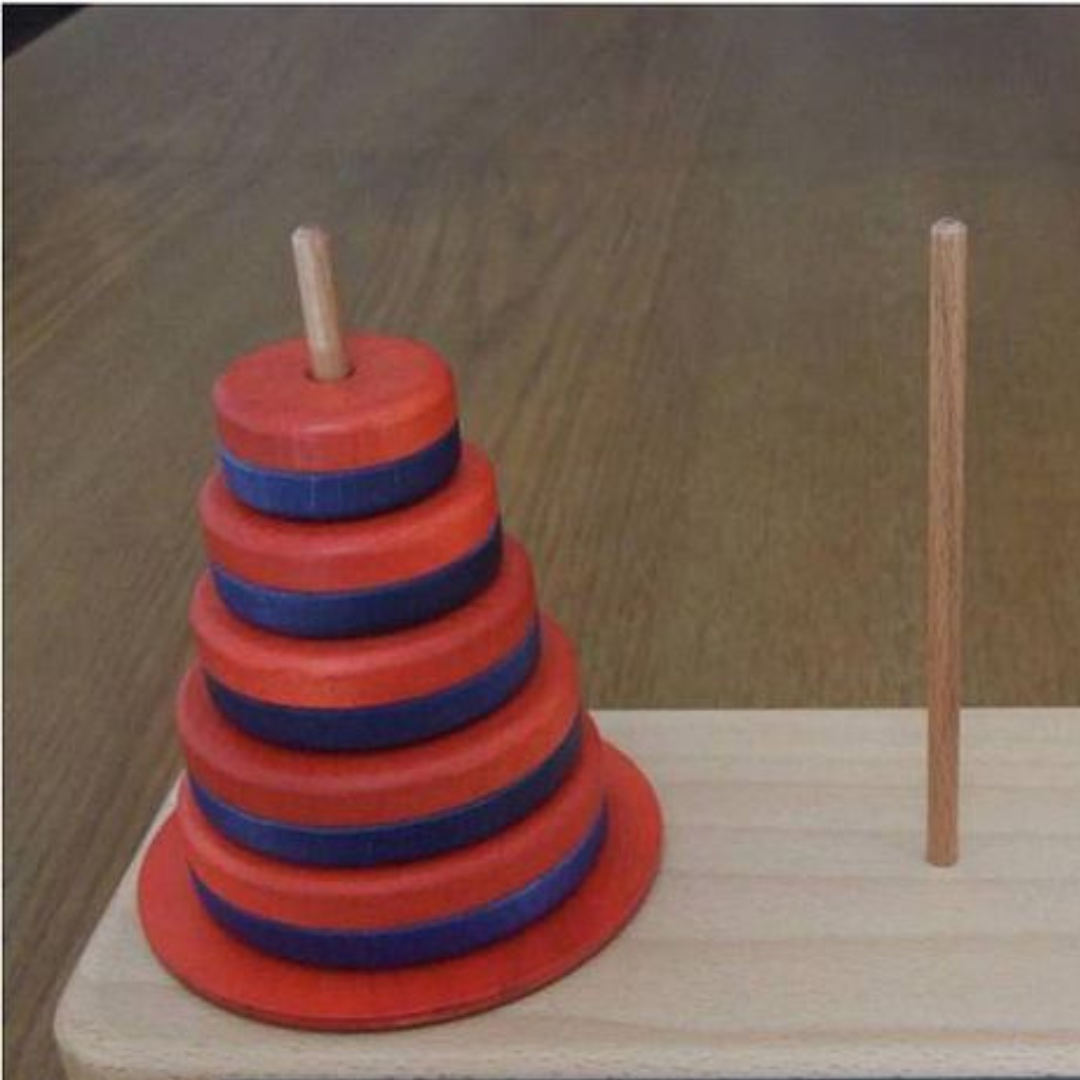
- 6th Apr 2023
- 09:23 am
C# Homework Question - C# Logical Puzzles, Games, and Algorithms: Tower of Hanoi
C# Homework Solution
Here's an example C# program for the Tower of Hanoi puzzle:
using System;
namespace TowerOfHanoi
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Enter the number of disks: ");
int n = int.Parse(Console.ReadLine());
Console.WriteLine("The sequence of moves involved in the Tower of Hanoi are: ");
TOH(n, 'A', 'C', 'B');
Console.ReadKey();
}
static void TOH(int n, char from_rod, char to_rod, char aux_rod)
{
if (n == 1)
{
Console.WriteLine("Move disk 1 from rod " + from_rod + " to rod " + to_rod);
return;
}
TOH(n - 1, from_rod, aux_rod, to_rod);
Console.WriteLine("Move disk " + n + " from rod " + from_rod + " to rod " + to_rod);
TOH(n - 1, aux_rod, to_rod, from_rod);
}
}
}
In this program, we take input from the user for the number of disks in the puzzle. Then, we call the TOH method to solve the puzzle and print the sequence of moves required to solve it.
The TOH method takes three arguments: n is the number of disks to be moved, from_rod is the rod from which the disks are to be moved, to_rod is the rod to which the disks are to be moved, and aux_rod is the auxiliary rod used for moving the disks.
If n is 1, we simply move the disk from from_rod to to_rod and return.
Otherwise, we move n-1 disks from from_rod to aux_rod using to_rod as the auxiliary rod. Then, we move the remaining disk from from_rod to to_rod. Finally, we move the n-1 disks from aux_rod to to_rod using from_rod as the auxiliary rod.