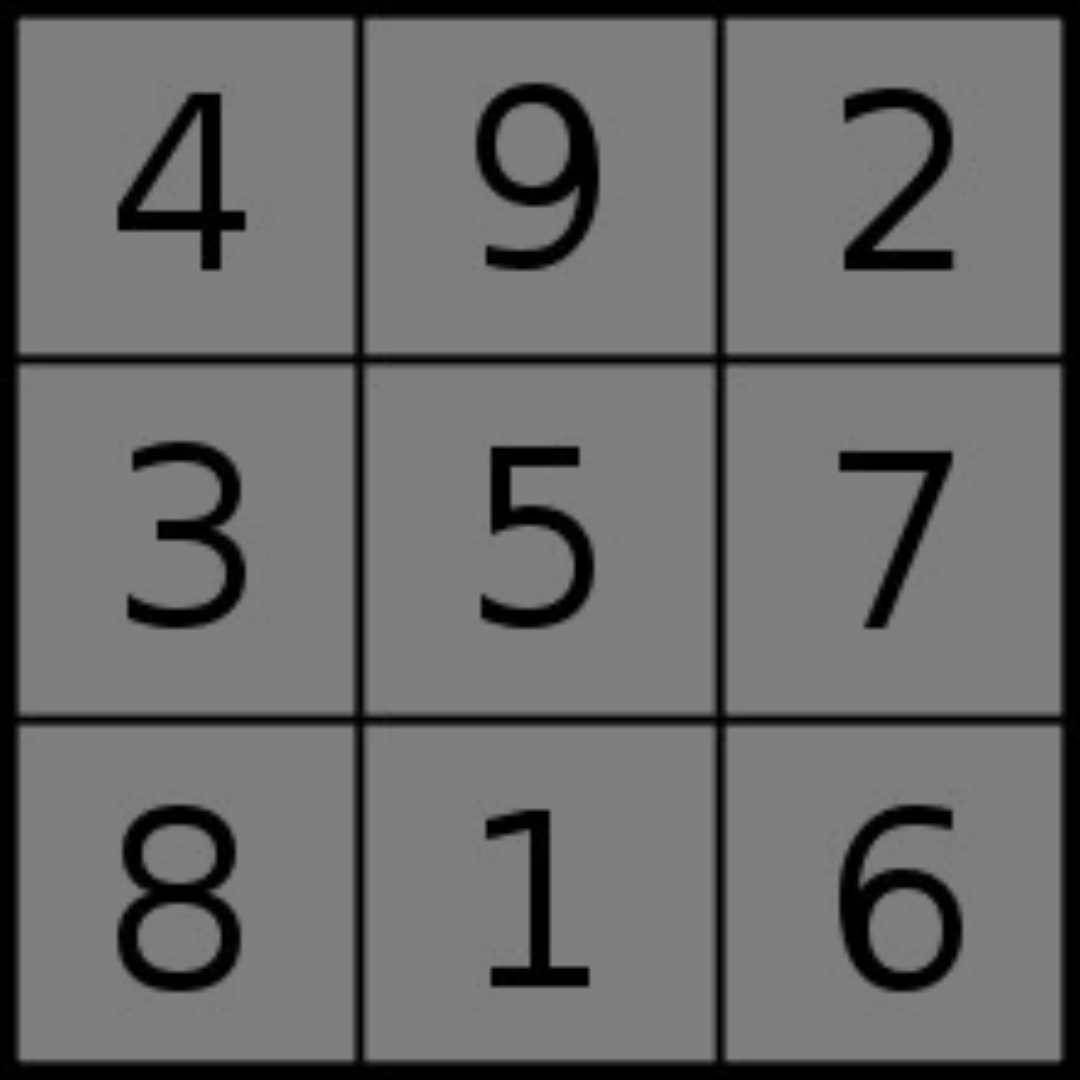
- 7th Apr 2023
- 08:21 am
- Admin
C# Homework Question - C# Logical Puzzles, Games, and Algorithms: Lo Shu Magic Square
C# Homework Solution
Here’s an example C# program for the Lo Shu Magic Square:
using System;
class MagicSquare
{
static void Main(string[] args)
{
int[,] square = new int[3, 3];
int num = 1;
// Initialize the square with zeros
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
square[i, j] = 0;
}
}
// Start at the center of the first row
int row = 0;
int col = 1;
// Fill in the square with the numbers 1-9
while (num <= 9)
{
square[row, col] = num;
// Move diagonally up and to the right
row--;
col++;
// If we go off the top or right edge, wrap around to the bottom or left edge
if (row < 0)
{
row = 2;
}
if (col > 2)
{
col = 0;
}
// If the next spot is already filled, move down one spot
if (square[row, col] != 0)
{
row++;
}
num++;
}
// Print out the magic square
Console.WriteLine("The Lo Shu Magic Square is:");
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
Console.Write("{0} ", square[i, j]);
}
Console.WriteLine();
}
// Check if the square is magic (sum of each row, column, and diagonal is 15)
if (square[0, 0] + square[0, 1] + square[0, 2] == 15 &&
square[1, 0] + square[1, 1] + square[1, 2] == 15 &&
square[2, 0] + square[2, 1] + square[2, 2] == 15 &&
square[0, 0] + square[1, 0] + square[2, 0] == 15 &&
square[0, 1] + square[1, 1] + square[2, 1] == 15 &&
square[0, 2] + square[1, 2] + square[2, 2] == 15 &&
square[0, 0] + square[1, 1] + square[2, 2] == 15 &&
square[0, 2] + square[1, 1] + square[2, 0] == 15)
{
Console.WriteLine("The Lo Shu Magic Square is magic!");
}
else
{
Console.WriteLine("The Lo Shu Magic Square is not magic.");
}
Console.ReadLine();
}
}
This program creates a 3x3 array representing the Lo Shu Magic Square, and fills it in using a specific algorithm. Then it checks if the square is a magic square (meaning the sum of each row, column, and diagonal is the same number), and prints a message indicating whether it is or not.