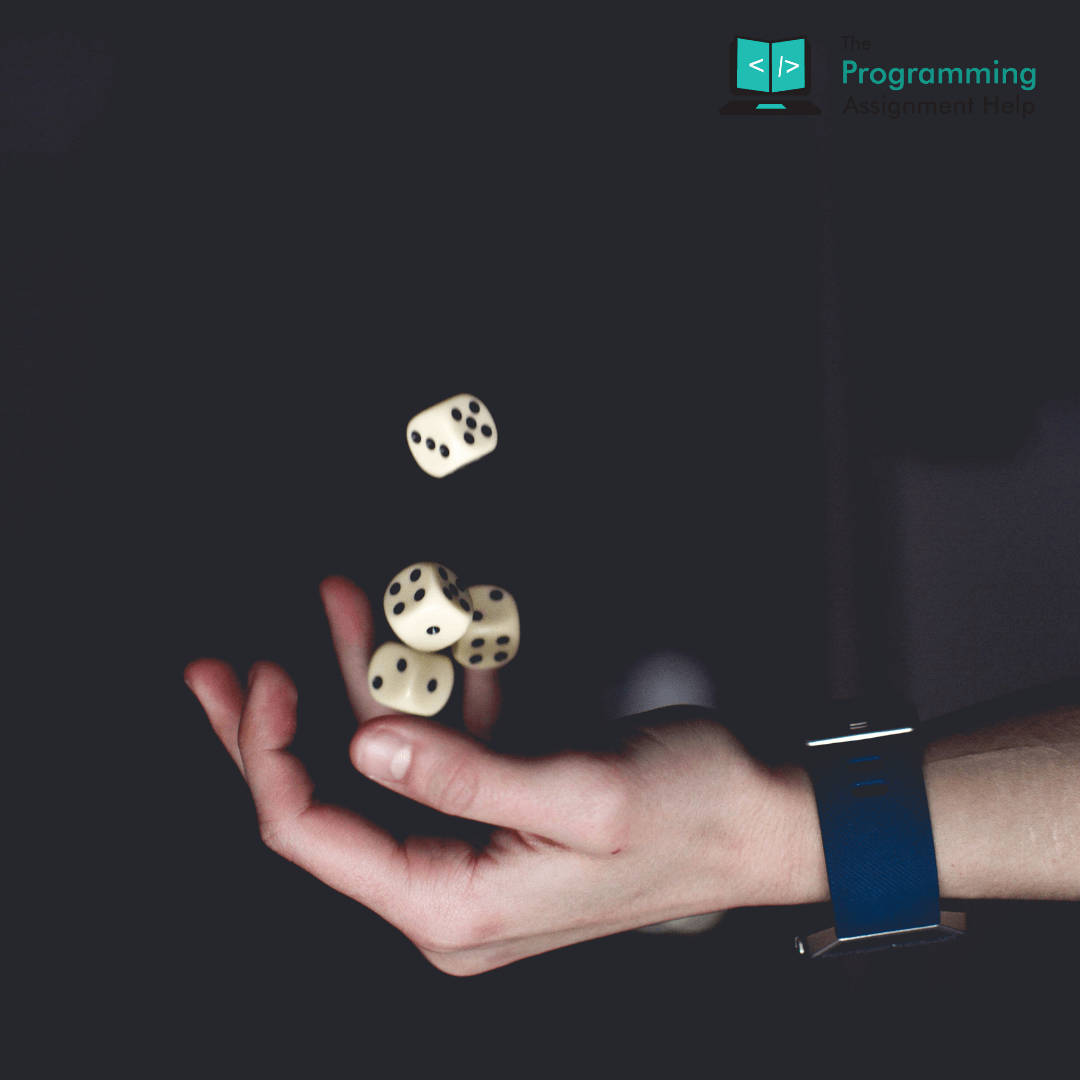
- 2nd Jun 2022
- 10:59 am
- Admin
The program defines several preprocessor commands and global variables related to the game's menu options and dice rolls. #include //preprocessor command to include header file stdio.h #include //preprocessor command to include header file stdlib.h #include //preprocessor command to include header file time.h int RULES=1; // initialize global variable RULES=1 int GAME=2;// initialize global variable GAME=2 int EXIT=3;// initialize global variable EXIT=3 int ROLLS=4;// initialize global variable ROLLS=4 int displayGameMenu();// function declaration function name : displayGameMenu void displayRandomDice();// function declaration function name : displayRandomDice int rollDie();// function declaration function name : rollDie void gameRules();// function declaration function name : gameRules, return type : void, parameter : no parameters passed void clearScreen();// function declaration function name : clearScreen, return type : void, parameter : no parameters passed int main()// main function with return type int { int play=1;//initialize play=1 srand(time(0));//call seed function while (play==1)//check whether play is equal to 1 or not { int disp=displayGameMenu();// call displayGameMenu function switch(disp)//switch case { case 1: gameRules();//call gameRules function break; case 2: clearScreen();//call clearScreen function displayRandomDice();//call displayRandomDice function break; case 3: printf("\nThank you for playing!"); play=0;// set play=0 break; default: printf("\nIncorrect option, hit enter and try again"); char enter; fflush(stdin);//flush the input scanf("%c",&enter); break; } } return 0;// return type of main function that is int } void displayRandomDice() { int numberOfRolls;//declare numberOfRolls int firstDie;//declare firstDie int secondDie;//declare secondDie int thirdDie;//declare thirdDie int fourthDie;//declare fourthDie int fifthDie;//declare fifthDie for(int i=0;i { firstDie=rollDie();//call function rollDie() & store it's value in firstDie secondDie=rollDie();//call function rollDie() & store it's value in secondDie thirdDie=rollDie();//call function rollDie() & store it's value in thirdDie fourthDie=rollDie();//call function rollDie() & store it's value in fourthDie fifthDie=rollDie();//call function rollDie() & store it's value in fifthDie printf("|------------------------------------------------|\n"); printf("| | | | | |\n"); printf("| %d | %d | %d | %d | %d |\n",firstDie,secondDie,thirdDie,fourthDie,fifthDie); printf("| | | | | |\n"); printf("|------------------------------------------------|\n"); } } int displayGameMenu() { int sheet=0;//initialize sheet =0 do { printf("\n%d. Display game rules",RULES); printf("\n%d. Start the game of yahtzee",GAME); printf("\n%d. Exit\n",EXIT); scanf("%d",&sheet);//get the user input }while(sheet<0 || sheet>4);//check whether user enter b/w 1 - 3 or not, if it's not repeat the loop. return sheet;//return value of sheet } int rollDie() { int dieValue=0;//initialize dieValue =0 dieValue=1+(rand()%6);//generate random number between 1 and 6 return dieValue;//return dievalue } void gameRules()// function definition of gameRules() { // print the rules of the game using a series of printf() statements with \n used for new lines printf("\nRULES OF THE CAME:"); printf("\n\t1. The scorecard used for Yahtlee is composed of an upper section and a lower section."); printf("\n\t2. A total of 13 scoring combinations are divided amongst the sections."); printf("\n\t3. The upper action consists of boxes that are scored by summing the value of tho dice notching the faces of t box."); printf("\n\t4. If a player rolls four 3's, then the score placed in the 5's box is the sum of the dice which is 12."); printf("\n\t5. Once a player has chosen to score a box, it may not be changed and the combination is no longer in play for uture rounds."); printf("\n\t6. If the sun of the scores in the upper section is greater than or equal to 63, then 35 more points are added to the players overall score as a bonus. The lower section contains a number of poker like combinations."); } void clearScreen()// function definition of clearScreen() { printf("\n Hit To Continue! ");// this statement is used to display the text on the screen char c;// declaring a variable of type character fflush(stdin); scanf("%c",&c);// storing the input given by user in c system("cls");// calling function system with "cls" passed as argument as it clears the screen }
Our Programming Assignment Help Expert Comments on the code
The code has some limitations and room for improvement:
- The game only simulates rolling the dice; it doesn't implement scoring, keeping track of rounds, or other game mechanics.
- There is no validation for user input, such as entering incorrect options or non-integer values when choosing from the menu.
- The program lacks error handling for invalid user input or file I/O errors (if applicable).
This program can serve as a starting point for implementing a more complete Yahtzee game with additional features, scoring, and user interactions.
If you have any specific questions or need further assistance, feel free to ask!