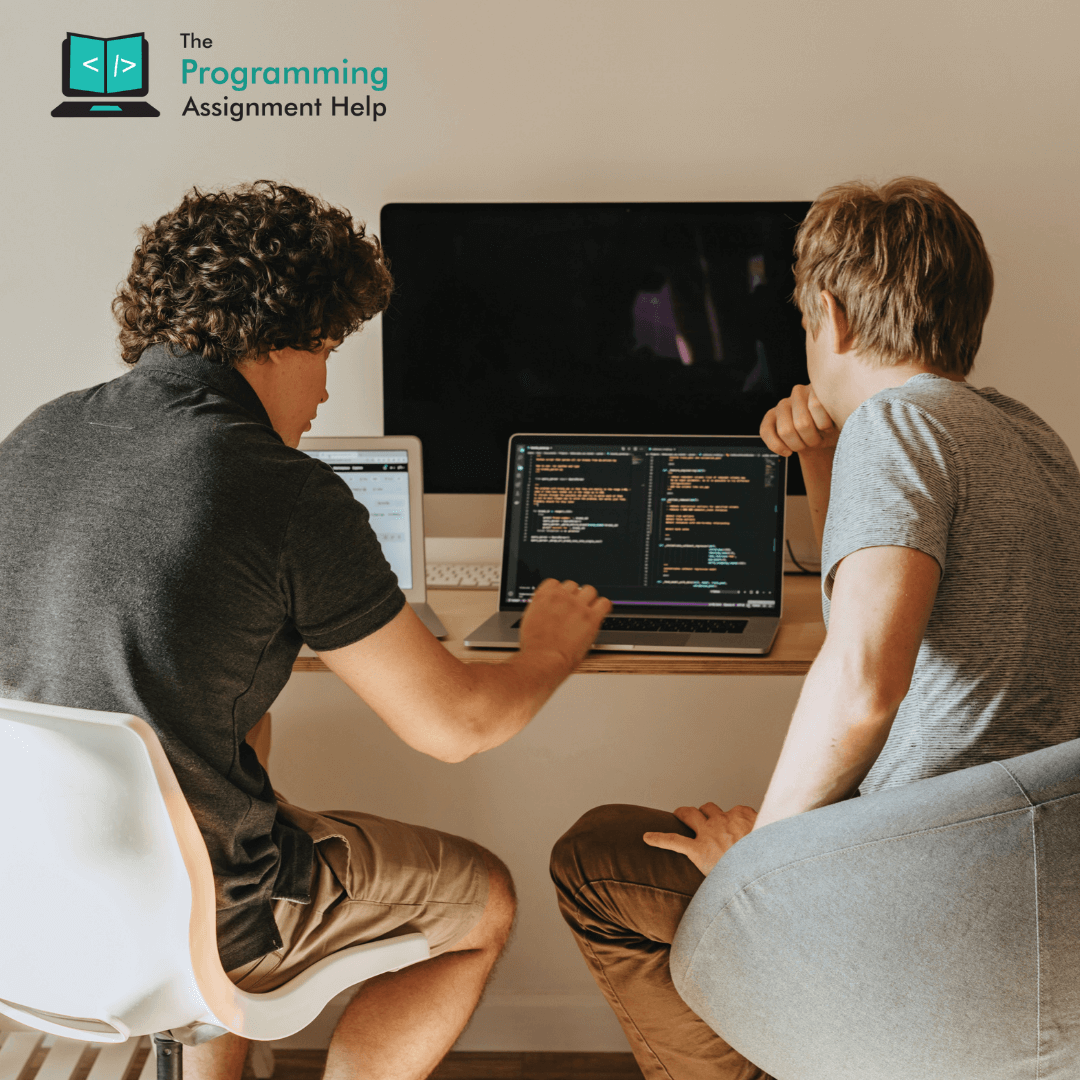
- 4th Feb 2022
- 04:37 am
- Admin
The provided code snippets seem to be two separate C++ programs. Let's discuss each program individually:
1. First Program:
This program generates random numbers using the Box-Muller transform to produce normally distributed random variables. It seems like the program generates 50 pairs of random numbers (ra, rb) and stores them in the 2D array r
.
#include #include #include #include #include using namespace std; # define M_PI 3.14159265358979323846 /* pi */ int main (){ int n; /*cin >> n; double *r; r=new double[n];*/ double r[50][2];//because n=100 double xa=0,xb=0; double pi=M_PI; double ra=0 ,rb=0; for(int i=0;i<50;i++){ xa=(rand()%10001)/10000.0; xb=(rand()%10001)/10000.0; ra=sqrt(-2*log(xa))*cos(2*M_PI*xb); rb=sqrt(-2*log(xa))*sin(2*M_PI*xb); r[i][0]=ra; r[i][1]=rb; cout<<"r"< cout << endl; } return 0; }
2.Second Program:
This program seems to be calculating the probability density function (PDF) of the standard normal distribution for different values of x
. It computes and prints the PDF values for seven different x
values, which are equally spaced between 0.125 and 0.875.
#include #include #include #include #include using namespace std; # define M_PI 3.14159265358979323846 /* pi */ int main (){ int n; double xa; double xb; int k[7]={1,2,3,4,5,6,7}; double fx[7]={0}; for(int k=0;k<7;k++){ xa=0.125*(k+1); fx[k]=(1/(sqrt(2*M_PI))) * exp((-1/2) * pow(xa,2)); cout<< fx[k]< } return 0; }
Overall, both programs appear to perform mathematical computations, one for generating random numbers following a normal distribution and the other for calculating the PDF of the standard normal distribution for specific x
values.