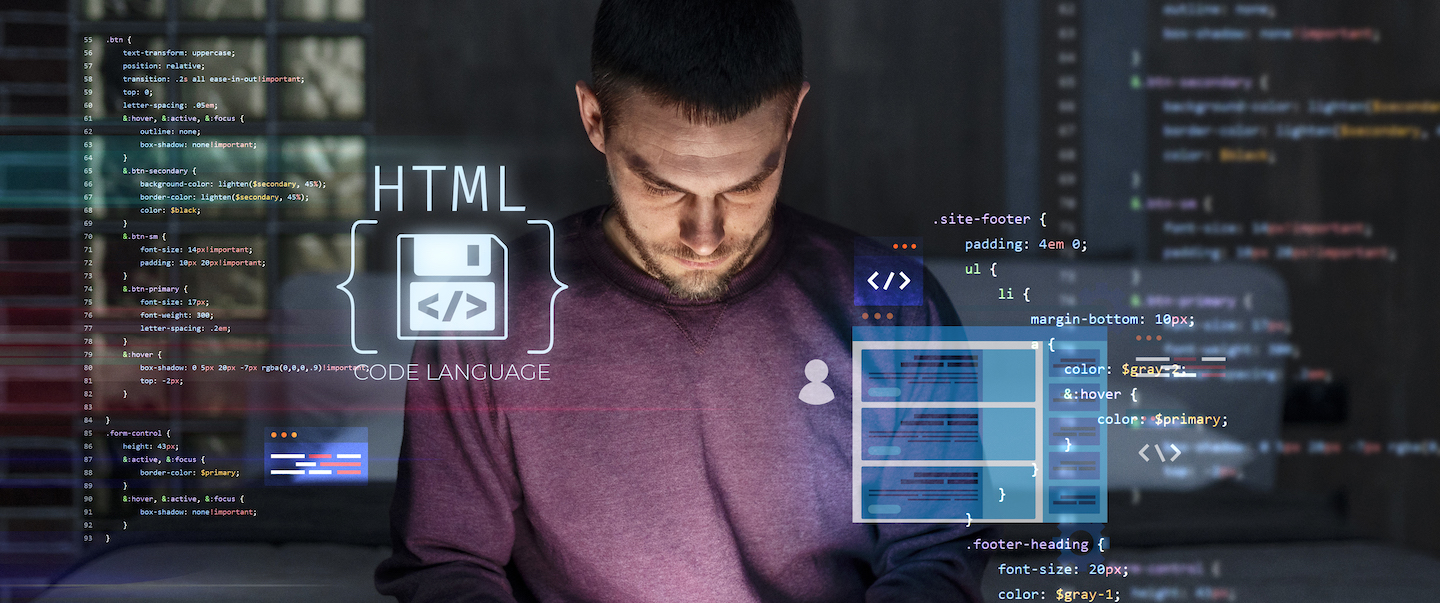
- 18th Dec 2023
- 21:37 pm
- Admin
Object-Oriented Programming (OOP) is a fundamental programming paradigm that emphasises the use of objects as major program components. C++ evolves as a dynamic and powerful programming language that strongly supports OOP concepts. Let's start with the basics of C++ Object-Oriented Programming:
- Objects and Classes
In C++, classes serve as blueprints, specifying both the structure and behaviour of objects. A class is a logical entity that has properties (data) and methods (functions). Objects, or instances of these classes, are representations of real-world entities that embody the attributes and behaviours described. Code can be organised into modular and reusable components using this technique. Objects interact with one another, and their behaviour is governed by the methods provided in their specific classes. C++ uses classes and objects to implement encapsulation concepts, providing a foundation for building well-structured and maintainable software.
- Encapsulation:
Encapsulation is a key idea in C++ Object-Oriented Programming that emphasises data and action grouping within a class. This concept forbids direct access to an object's internal state and instead provides control using access modifiers such as "public," "private," and "protected." The encapsulated data is kept private by the class, and interactions occur through well-defined interfaces (public methods). This safeguards implementation details, increases code security and promotes modularity. Encapsulation enables effective data concealment, the prevention of undesirable changes, and the creation of robust, maintainable, and scalable software systems.
- Inheritance:
In the world of C++ Object-Oriented Programming, inheritance is extremely important. It allows for the construction of new classes by expanding on current ones. A derived class inherits traits and actions from its parent class, allowing for code reuse and feature development. This relationship establishes a "is-a" relationship, which means that objects of the derived class are also objects of the base class. Inheritance introduces polymorphism, which allows objects of different classes to be treated consistently via a single interface. Among the benefits that contribute to the establishment of modular and extensible software systems in C++ Object-Oriented Programming are improved code structure, promoting hierarchy, and expediting the implementation of shared capabilities.
- Polymorphism:
Polymorphism, a fundamental notion in C++ Object-Oriented Programming, allows for the consistent treatment of various object types via a single interface. By enabling many functions with the same name but different arguments, function overloading enables compile-time polymorphism. By allowing a base class reference to refer to objects of derived classes and dynamically binding method calls, virtual functions enable runtime polymorphism. Polymorphism increases code flexibility, simplifies implementation, and enables the creation of extendable software. It promotes the design idea of "one interface, multiple implementations," making modular and adaptive C++ programming structures possible.
- Abstraction:
In C++, abstraction entails simplifying complicated systems by concentrating on key features while concealing unneeded details. It is a fundamental Object-Oriented Programming idea realized through abstract classes and interfaces. Abstract classes serve as a pattern for other classes and may have abstract methods that are not implemented. Objects interact with abstract types, with the emphasis on what an object does rather than how it does it. Abstraction assists developers in managing complexity, improving code readability, and developing scalable and adaptive systems. By providing a high-level view, abstraction encourages the development of software that is easier to understand, maintain, and grow.
- Constructor and Destructor:
Constructors and destructors are critical components of C++ Object-Oriented Programming. Constructors ensure that objects are in a correct state when they are created, whereas destructors handle the cleanup process when an object exits the scope or is deliberately destroyed. They have the same name as the class and can be passed parameters. Destructors, whose names begin with a tilde (`~`), free up resources as objects exit scope, making memory management simpler. Constructors and destructors aid in object lifecycle management, appropriate initialization, and resource release, all of which increase C++ program resilience and speed. Their exact implementation is essential for producing dependable and well-behaved object instances in a program.
- Operator Overloading:
In C++, operator overloading allows programmers to modify the behaviour of standard operators for user-defined data types. This handy feature can be used by classes to construct unique implementations of operations such as addition, subtraction, and comparison. Overloading operators enhances code readability and expressiveness, making user-defined objects easier to deal with. By simplifying difficult operations, promoting code consistency, and allowing for a more natural representation of user-defined types, this feature increases the beauty and flexibility of C++ programs. However, it should be utilized with caution to maintain clarity and avoid unintended consequences.
- Dynamic Memory Allocation:
In C++, dynamic memory allocation is a mechanism that allows programs to request and manage memory while they are running. The 'new' operator is used to allocate memory for objects on the heap, giving you more control over your memory resources. This allows for the creation of data structures with variable size and longevity. To avoid memory leaks, proper deallocation is required, which is achieved with the 'delete' operator. Dynamic memory allocation is advantageous when the amount of data is uncertain at compile time, providing efficiency and adaptability in memory consumption, especially when working with dynamic arrays or generating objects with different lifetimes.
C++ Object-Oriented Programming is a popular software development paradigm because it allows for modular, scalable, and maintainable code. Because of the language's flexibility, developers can blend OOP concepts with procedural and generic programming, resulting in a more diverse approach to software development.
Applications of C++ Object-Oriented Programming
Object-Oriented Programming (OOP) in C++ has various benefits, including the ability to design, build, and maintain strong and modular applications. Here are a few examples of major OOP applications in C++:
- Modularity and Reusability: OOP promotes modularity by breaking down complex systems into smaller, more manageable classes. These classes can be reused throughout the application or in other projects to increase code reuse. Modularity facilitates maintenance and updates because modifications can be constrained to certain classes.
- Ease of Maintenance: OOP ideas help with code maintenance. Encapsulation and abstraction make code easier to understand and modify. Furthermore, the modular structure and detailed class hierarchies facilitate the discovery and resolution of problems without affecting other parts of the system.
- Flexibility and Extensibility: OOP allows present code to be expanded by introducing new classes or updating existing ones. This versatility comes in handy when it comes to adding features or adjusting software to meet changing needs. OOP is widely used to achieve the open/closed idea, which encourages contributions while not requiring changes to existing code.
- Improved collaboration: OOP improves collaboration among project developers. Because each class can be written and tested independently, numerous team members can work on different areas of the system concurrently. The use of well-defined interfaces also helps with effort coordination.
- Real-World Simulation: Because OOP is so closely tied to real-world modelling, expressing entities, connections, and actions in software is much easier. This alignment improves communication between developers and domain experts, leading to more effective and precise software design.
- Hierarchy and Classification: OOP enables the creation of class hierarchies to represent object connections. This hierarchical architecture allows for the classification of objects based on shared characteristics and activities, which improves the system's conceptual clarity.
OOP in C++ takes advantage of these characteristics to provide a robust and adaptable paradigm for designing and implementing software systems, promoting excellent software engineering practices, and allowing the development of scalable and maintainable applications.
Example of C++ Object-Oriented Programming
a simple C++ program that demonstrates the use of Object-Oriented Programming (OOP) principles. In this example, we'll create a basic system to model a library with books.
```cpp
#include
#include
// Class representing a Book
class Book {
private:
std::string title;
std::string author;
public:
// Constructor to initialize book details
Book(const std::string& _title, const std::string& _author) : title(_title), author(_author) {}
// Function to display book information
void displayInfo() const {
std::cout << "Title: " << title << "\nAuthor: " << author << std::endl;
}
};
// Class representing a Library
class Library {
private:
std::vector books;
public:
// Function to add a book to the library
void addBook(const Book& book) {
books.push_back(book);
}
// Function to display all books in the library
void displayBooks() const {
std::cout << "Books in the Library:\n";
for (const Book& book : books) {
book.displayInfo();
std::cout << "------------\n";
}
}
};
int main() {
// Creating books
Book book1("The Catcher in the Rye", "J.D. Salinger");
Book book2("To Kill a Mockingbird", "Harper Lee");
Book book3("1984", "George Orwell");
// Creating a library
Library myLibrary;
// Adding books to the library
myLibrary.addBook(book1);
myLibrary.addBook(book2);
myLibrary.addBook(book3);
// Displaying all books in the library
myLibrary.displayBooks();
return 0;
}
```
Explanation:
- Book Class: The 'Book' class represents a book and has the properties 'title' and 'author'. It has a constructor to initialise these attributes and a function to display book information ('displayInfo()').
- Library Class: The 'Library' class represents a library with several books. It includes a vector ('books') for storing Book objects. It offers utilities for adding books to the library and displaying all books ('addBook()' and 'displayBooks()').
- Main Function:
- In the `main()` function, three Book objects are created representing different books.
- We begin by creating an instance of the Library class (`myLibrary`).
- Books are added to the library using the `addBook()` function.
- To showcase the library's collection, the `displayBooks()` function is invoked.
This program demonstrates the essential ideas of object-oriented programming through realistic demonstrations of encapsulation, abstraction, and class interaction. The classes 'Book' and 'Library' encapsulate data and functionality, resulting in a modular and organised framework. The programme simulates a simple scenario, showcasing the power of OOP in code design and organisation.