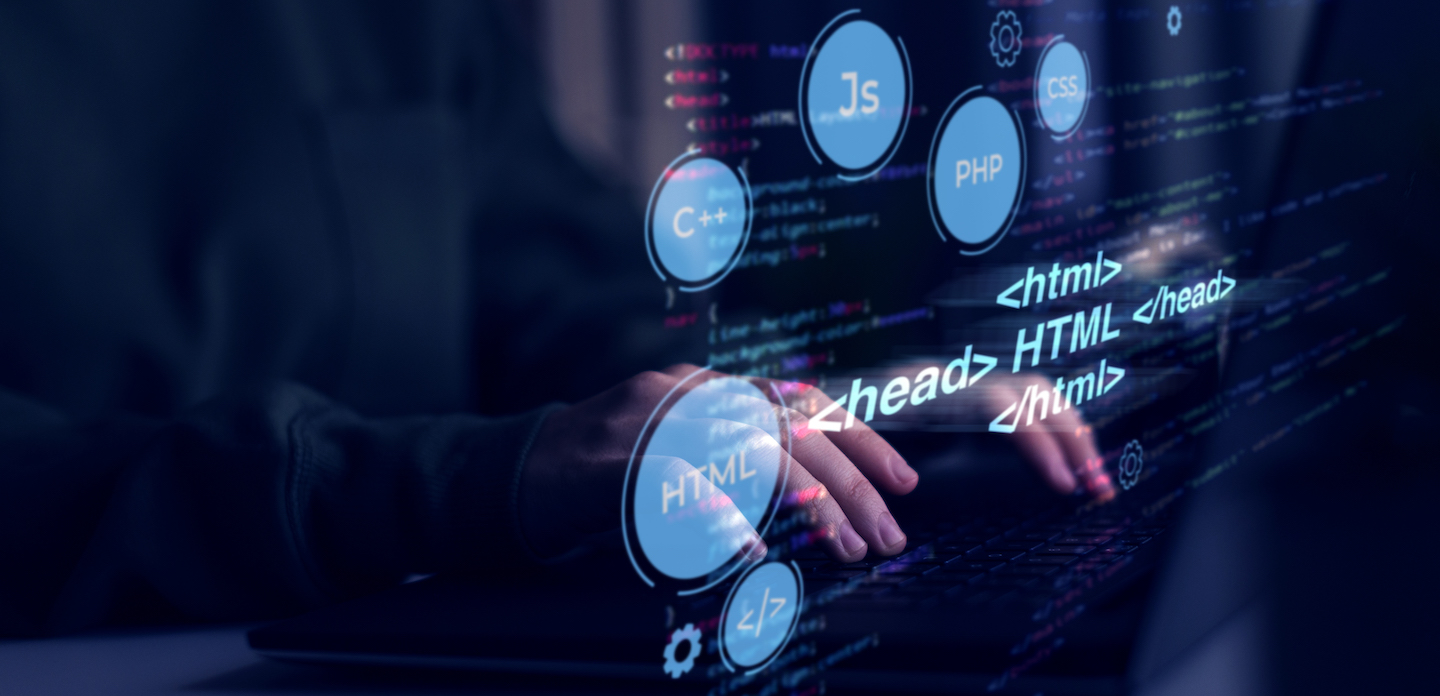
- 18th Dec 2023
- 23:30 pm
- Admin
In C++ and object-oriented programming (OOP), inheritance is an important idea. It helps make a new class (called derived or child class) by using things from an existing class (called base or parent class). The new class can then use and add more things to what the old class can do. It makes it simple to reuse, organize, and arrange code in an organized manner.
Inheritance establishes a link by stating that the new class "is a" subset of the existing class. The old class has some elements in common, and the new class can add or replace them as appropriate. In C++, inheritance allows us to create new objects based on what already exists, making our code more efficient and organized. In C++, there are two ways to inherit: The first is when a class inherits from only one other class (single inheritance), and the second is when a class inherits from numerous classes (multiple inheritance). This aids in the formation of various forms of links between classes.
Inheritance also aids in the creation of abstract classes and interfaces. These are tools that make it easier to use various aspects of a program. They support polymorphism, which is a method of making several things operate together.
So, in a nutshell, inheritance is critical in C++. It facilitates the clever use and sharing of code, making programs easier to understand and grow. It's like constructing with bricks, where each block has unique abilities that we can combine to make something incredible!
Difference between base class and Derived Class
A base class and a derived class are two important components that establish a hierarchical connection in C++ inheritance. Here are the fundamental distinctions between them:
Definition and Function:
- Base Class: A base class, also known as a parent class or superclass, is the class from which other classes (derived classes) receive their characteristics and behaviours. It acts as the basis, offering a standardised set of properties and actions.
- Derivative Class: A derived class, also known as a child class or subclass, inherits attributes and behaviours from a base class. It has the ability to expand or enhance the functionality of the underlying class by adding new features or overriding existing methods.
Direction of Inheritance:
- Base Class: It derives from no other classes. It is the root of the inheritance hierarchy.
- Derivative Class: It is descended from a base class. It gains access to the underlying class's members (attributes and methods).
Usage and Specialisation:
- Base Class: Typically reflects a generalised or commonly understood idea. It has shared properties and methods that apply to several derived classes.
- Derived Class: Represents a subset of the base class. It inherits the base class's common functionality and may add new features or alter existing ones to meet special needs.
Object Creation:
- Base Class: The base class can be used to construct objects directly. These objects will have access to the underlying class's properties and functions.
- Derivative Class: Objects are frequently generated using the derived class. They inherit the base class's features, but they can additionally display the specialised behaviour introduced in the derived class.
Relationship:
- Base Class: Is unaware of the derived classes. It is intended to be a self-contained class with general functionality.
- Derived Class: Recognises its connection to the base class. It adds to or changes the functionality passed down from the base class.
In C++ inheritance, a base class offers a common basis, and a derived class builds on it, producing a hierarchy that enables code reuse, modularity, and specialisation.
Types of Inheritance in C++
In C++, there are several types of inheritance, each serving specific purposes in the organization of class hierarchies. Here are the main types:
- Single Inheritance:
A derived class inherits from only one base class.
Example: Class `Car` (base) and class `SportsCar` (derived).
- Multiple Inheritance:
A derived class inherits from more than one base class.
Example: Class `Student` (derived from `Person` and `Course`).
- Multilevel Inheritance:
A derived class becomes a base class for another class, forming a chain.
Example: Class `Animal` (base), class `Mammal` (derived), and class `Dog` (derived from `Mammal`).
- Hierarchical Inheritance:
Multiple classes inherit from a single base class.
Example: Class `Shape` (base), class `Circle` (derived), and class `Rectangle` (derived).
- Hybrid Inheritance:
Combination of two or more types of inheritance.
Example: A class `ElectricCar` inheriting from `Car` (single inheritance) and implementing an interface `ChargingInterface` (interface inheritance).
- Virtual Inheritance:
Used to address the "diamond problem" in multiple inheritance by creating a shared base class.
Example: Classes `A`, `B`, and `C` where `B` and `C` virtually inherit from `A` to avoid ambiguity.
These inheritance types provide different ways to build classes in a program, allowing developers to represent relationships based on the specific demands of their application.
The Benefits of C++ Inheritance
Inheritance is a strong feature in C++ that provides various benefits, enhancing the efficacy and flexibility of object-oriented programming. Here are some significant benefits:
- Code Reusability: Inheritance allows code from existing classes to be reused. A base class's features and behaviours can be inherited by one or more derived classes, minimizing redundancy and promoting modular design.
- Modularity: Inheritance lends itself to a modular approach to software development. Base classes encompass common functionalities, which derived classes augment or specialise. This modular structure improves the code's maintainability and understandability.
- Polymorphism: Polymorphism is facilitated by inheritance, which allows objects of derived classes to be treated as objects of the base class. This permits dynamic binding and runtime polymorphism, increasing the program's flexibility and adaptability.
- Extensibility: By constructing derived classes, new functionalities can be added to a program without altering existing code in the base class. This extensibility makes it easier to incorporate new features and react to changing requirements.
- Hierarchy and Organisation: Inheritance enables for the establishment of class hierarchies, which provides the code with a clear and intuitive structure. This hierarchy depicts the links between distinct elements in the problem area, improving overall software organisation.
- Readability and Maintainability: Inheritance increases code readability by describing class relationships in a natural and intuitive manner. Changes to the base class are automatically propagated to derived classes, making maintenance and updates easier.
- Abstraction and Encapsulation: Base classes are frequently used to represent abstract notions by providing generalised characteristics and methods. Inheritance facilitates the encapsulation of these abstract concepts, letting developers to concentrate on high-level design rather than implementation concerns.
Inheritance in C++ improves software system design and implementation by encouraging code reuse, modularity, extensibility, and a clear hierarchy. It contributes to the creation of maintainable, legible, and adaptive code that adheres to object-oriented programming concepts.
Program using Inheritance
A simple example of a C++ program that demonstrates inheritance. In this example, there is a base class `Vehicle`, and two derived classes `Car` and `Motorcycle` that inherit from the base class.
```
#include
#include
// Base class
class Vehicle {
protected:
std::string brand;
public:
Vehicle(const std::string& b) : brand(b) {}
void displayInfo() const {
std::cout << "Brand: " << brand << std::endl;
}
};
// Derived class 1
class Car : public Vehicle {
private:
int numDoors;
public:
Car(const std::string& b, int doors) : Vehicle(b), numDoors(doors) {}
void displayCarInfo() const {
displayInfo();
std::cout << "Type: Car" << std::endl;
std::cout << "Number of Doors: " << numDoors << std::endl;
}
};
// Derived class 2
class Motorcycle : public Vehicle {
private:
bool hasSideCar;
public:
Motorcycle(const std::string& b, bool sideCar) : Vehicle(b), hasSideCar(sideCar) {}
void displayMotorcycleInfo() const {
displayInfo();
std::cout << "Type: Motorcycle" << std::endl;
std::cout << "Has Sidecar: " << (hasSideCar ? "Yes" : "No") << std::endl;
}
};
int main() {
// Creating objects of derived classes
Car myCar("Toyota", 4);
Motorcycle myMotorcycle("Harley Davidson", true);
// Displaying information using derived class methods
std::cout << "Car Information:" << std::endl;
myCar.displayCarInfo();
std::cout << "\nMotorcycle Information:" << std::endl;
myMotorcycle.displayMotorcycleInfo();
return 0;
}
```
Explanation:
- The `Vehicle` class is the base class, containing a member variable `brand` and a method `displayInfo()` to display the brand information.
- The `Car` and `Motorcycle` classes are derived from the `Vehicle` class using the `public` access specifier. They inherit the `brand` member and `displayInfo()` method from the base class.
- Each derived class has its own additional attributes (`numDoors` for `Car` and `hasSideCar` for `Motorcycle`) and methods (`displayCarInfo()` and `displayMotorcycleInfo()`) specific to the type of vehicle.
- In the `main` function, objects of the derived classes are created (`myCar` and `myMotorcycle`), and their information is displayed using the methods defined in the respective classes.
This program demonstrates the concept of inheritance, where the derived classes (`Car` and `Motorcycle`) inherit common functionality from the base class (`Vehicle`) and extend it with their own specific attributes and behaviors.
About the Author - Jane Austin
Jane Austin is a 24-year-old programmer specializing in Java and Python. With a strong foundation in these programming languages, her experience includes working on diverse projects that demonstrate her adaptability and proficiency in creating robust and scalable software systems. Jane is passionate about leveraging technology to address complex challenges and is continuously expanding her knowledge to stay updated with the latest advancements in the field of programming and software development.