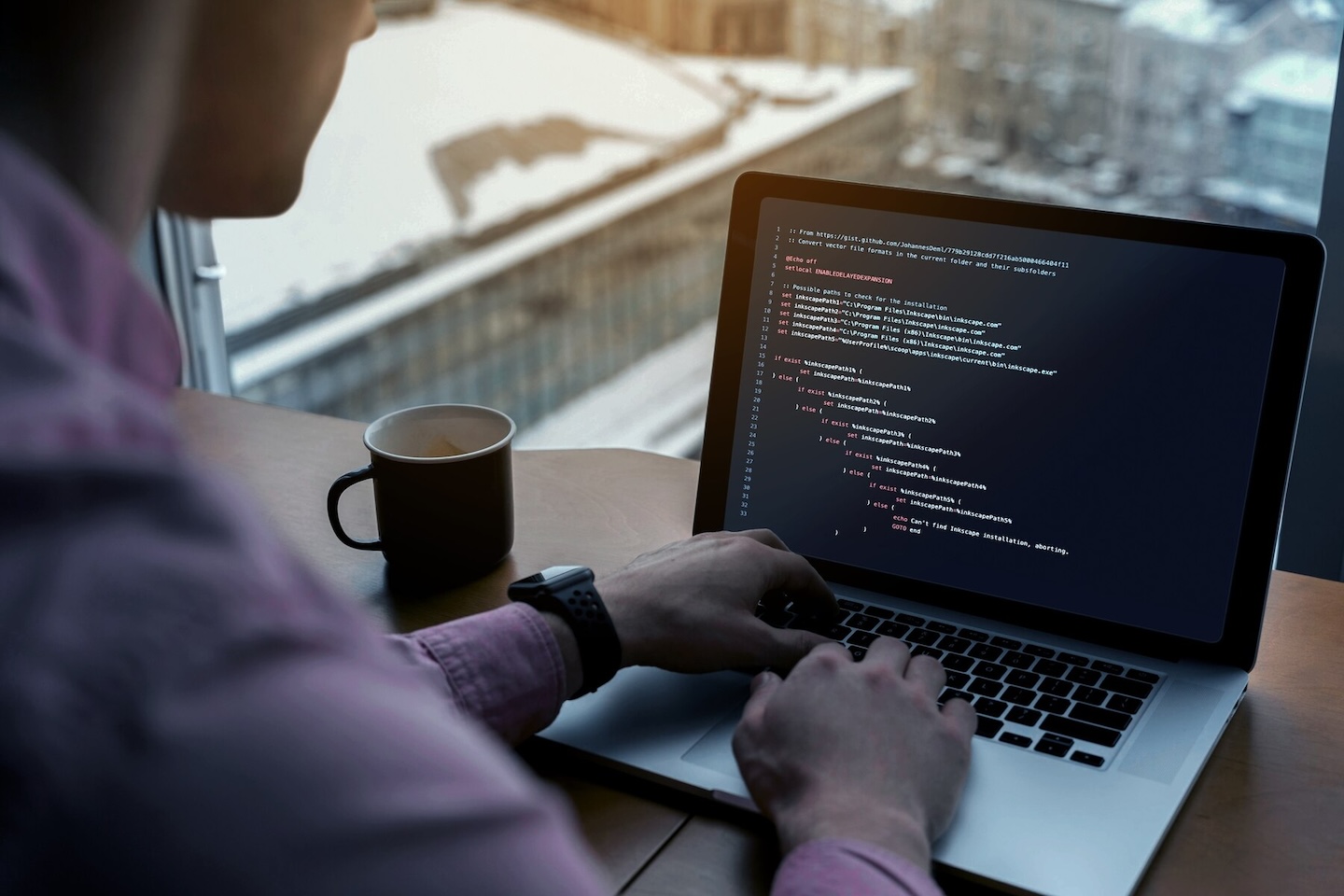
- 12th Dec 2023
- 23:47 pm
- Admin
Exception handling in C++ is a sophisticated tool that allows programs to handle and respond to unexpected runtime faults or exceptional conditions in a program in a gracious manner. Exception handling provides an organised approach to error management, making code more robust and preventing abrupt terminations.
By isolating the usual flow of code from error-handling logic, exception handling increases code dependability and maintainability. It allows developers to handle failures at the right levels of the program hierarchy and encourages the development of strong applications that gracefully handle unforeseen circumstances. Using exception handling correctly improves program stability by making it easier to identify, handle, and recover from mistakes.
Key Aspects of C++ Exception Handling
Here are key aspects of C++ exception handling:
- Exceptions Thrown:
When an error or exceptional event happens, a program can raise an exception by using the 'throw' keyword. Any data type, including fundamental types, pointers, and class types, can be an exception.
- Catching Exceptions:
The 'try' and 'catch' blocks are used to manage exceptions. The 'try' block contains the code that may throw an exception, whereas the 'catch' section explains how to handle the exception. To handle various types of exceptions, multiple 'catch' blocks can be utilised.
- The 'catch' Block:
Each 'catch' section corresponds to a distinct exception type. If that type (or a derived type) of exception is thrown in the 'try' block, the associated 'catch' block is executed. If no matching 'catch' blocks are found, the program exits or calls the 'terminate()' function.
- Exception Handling Hierarchy:
C++ allows an exception hierarchy. Catch blocks for base classes can catch derived classes. This enables the handling of exceptions with varying degrees of specificity.
- The 'throw' Statement:
The 'throw' statement is used to explicitly throw an exception. It can be followed by an expression containing the exception's value. The kind of the exception is determined by the type of the phrase.
- The 'try' Block:
The 'try' block contains code that may raise an exception. If an exception is thrown, the program goes to the first 'catch' block that matches.
- The 'finally' Block (Optional):
Unlike some other programming languages, C++ lacks a 'finally' block. Resource management, on the other hand, can be done in a 'catch' block or using RAII (Resource Acquisition Is Initialization) concepts.
- Unhandled Exceptions:
If no 'catch' blocks catch an exception, the'std::terminate()' function is executed, resulting in program termination. 'std::set_terminate()' can be used to create custom handling for unhandled exceptions.
C++ exception handling promotes more resilient and maintainable code by providing an organised and efficient mechanism to manage mistakes and exceptional conditions. It separates error detection code from error handling code, improving code readability and maintainability.
Major Uses of Exception Handling in C++:
- Error Propagation:
Exception handling allows errors to be propagated through the call stack. When an error happens in a lower-level function, it can throw an exception, which the program can catch and handle at a higher level. This encourages cleaner error handling by eliminating the need for substantial error-checking code at each level.
- Separation of Concerns:
Exception handling isolates error detection code from error handling code. This encourages a better organised and readable code structure. The program's usual flow is not obstructed by error-checking logic, and error-handling code is contained in particular 'catch' blocks.
- Robustness in Unforeseen events:
Exception handling improves a program's robustness by offering a means to elegantly manage unforeseen events. Rather than stopping the program abruptly, exceptions allow it to respond to mistakes in a controlled manner, potentially recovering from or logging errors before terminating.
- Resource Management:
Exception handling is essential for effective resource management. Exceptions ensure that cleaning code (such as destructors) is called even if an error occurs when resources such as memory, file handles, or network connections must be acquired and relinquished.
- Standardised Error Handling Across Layers:
Exception handling provides a consistent mechanism to manage failures across different components in applications with several levels or modules. This is especially useful in large software projects when maintaining a consistent error-handling method is critical for code maintainability.
- Exception Types for Different Scenarios:
C++ allows developers to define unique exception types, allowing them to construct specific exception classes for different problem scenarios. This enables more granular and expressive error reporting, making specific sorts of issues easier to understand and respond to.
- Graceful Degradation and Recovery:
Exception handling enables a program to gracefully degrade or recover from faults. Instead of crashing, the program can catch exceptions, log pertinent information, and take necessary actions to continue execution, resulting in a more pleasant user experience.
- Testing and Debugging:
Exception handling aids in the identification and resolution of unexpected conditions during the testing and debugging processes. Exception messages and stack traces are useful for troubleshooting and determining the root cause of failures.
Exception handling allows developers to create more resilient and maintainable software that gracefully handles mistakes and unexpected situations, contributing to overall software quality improvement.
Major Advantages of Exception Handling in Programming:
- Improved Code Readability:
Exception handling enables developers to decouple normal code flow from error-handling functionality. This split improves code readability by keeping the primary logic free of several error-checking conditions. As a result, the code is clearer, more intelligible, and easier to maintain.
- Increased Robustness:
Exception handling increases a program's robustness by offering a structured approach to handle unexpected events and failures. Exceptions allow developers to respond to problems in a controlled manner, either recovering from or logging faults before terminating the program.
- Simplified Error Propagation:
Errors can be propagated through the call stack via exception handling without the need for explicit error-checking at each level. This streamlines error propagation and makes handling problems at suitable levels of the code structure easy. It also eliminates the requirement for numerous error-checking conditions throughout the code.
- Standard Error Handling Strategy:
Exception handling enables the implementation of a standard error-handling strategy across an application. Developers can create distinct exception types for various mistake cases, ensuring a consistent approach to error reporting and handling across the codebase. This uniformity is beneficial to code maintenance and readability.
- Resource Cleanup and Management:
Exception handling is essential for resource management, particularly when resources must be obtained and relinquished. Developers can ensure that resources are correctly released even if an error happens by using destructors and cleaning code in 'catch' blocks, reducing resource leaks and enhancing overall system stability.
- Better Debugging and Troubleshooting:
Exception handling gives useful information while debugging and troubleshooting. When an exception is thrown, the system often produces a stack trace, which shows the sequence of function calls that resulted in the exception. This information is extremely useful for diagnosing problems, determining the cause of errors, and facilitating the debugging process.
- Graceful program Termination:
Exception handling enables a program to exit gracefully in the event of irrecoverable mistakes. Instead of crashing abruptly, the program can catch exceptions, log pertinent information, and take appropriate departure actions. This leads to a better user-friendly experience and aids in the identification and resolution of difficulties.
When implemented correctly, exception handling contributes to a software application's overall dependability, maintainability, and user experience by offering a systematic approach to addressing errors and exceptional conditions.
Example of Exception Handling in C++:
C++ program that uses exception handling
```
#include
int main() {
try {
// Get user input for two numbers
std::cout << "Enter the numerator: ";
double numerator;
std::cin >> numerator;
std::cout << "Enter the denominator: ";
double denominator;
std::cin >> denominator;
// Check for division by zero
if (denominator == 0) {
throw std::runtime_error("Error: Division by zero is not allowed.");
}
// Perform division and display the result
double result = numerator / denominator;
std::cout << "Result of division: " << result << std::endl;
} catch (const std::exception& e) {
// Catch and handle exceptions
std::cerr << "Exception caught: " << e.what() << std::endl;
}
return 0;
}
```
Explanation:
- A 'try' block is used in the program to contain code that may throw an exception.
- The program receives user input for the numerator and denominator within the 'try' section.
- Before dividing, the program checks to see if the denominator is zero. If it is, the program generates a'std::runtime_error' error with a detailed error message.
- The 'catch' block handles exceptions of type'std::exception' (and its related kinds). It outputs an error message to the standard error stream ('std::cerr') in this situation.
- If no exceptions are encountered, the program computes and shows the division result.
Using exception handling, the program gracefully manages the probable error of division by zero, presenting the user with a more controlled and informative answer.
This example shows the fundamental structure of C++ exception management and how it can be used to handle certain exceptional conditions.