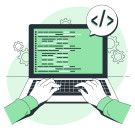
- 16th Nov 2023
- 23:04 pm
- Admin
C++ Programming functions serve as modular units of code, enabling task-specific functionalities and facilitating organized, reusable code. They encompass user-defined and standard library functions, operating through different parameter-passing mechanisms and promoting code modularity and reusability within C++ programming paradigms.
What Are C++ Functions?
C++ functions are encapsulated blocks of code designed to perform specific tasks, fostering modularity, reusability, and improved code organization. Functions in programming languages allow the breakdown of complex operations into smaller, more manageable units. This practice significantly enhances the readability and maintenance of code.
By segmenting logic into discrete functional blocks, functions enable code to be efficiently reused across the program, minimizing redundancy. These modular components facilitate easier comprehension of program flow and structure, promoting better code maintenance, debugging, and scalability. Ultimately, C++ functions serve as vital building blocks for developing efficient, structured, and easily maintainable programs.
Types of Functions in C++
C++ offers distinct types of functions, categorized into user-defined and standard library functions.
- User-Defined Functions
User-Defined Functions, created by programmers, serve to execute particular tasks, promoting modularity and enabling code reusability within programs. These functions are tailored to fit unique application requirements, breaking complex operations into manageable sections.
- Standard Library Functions
Standard Library Functions are pre-established functions available within the C++ Standard Library. They encompass a broad spectrum of functionalities including mathematical operations, string manipulation, and input/output tasks, providing predefined solutions for common programming challenges. This collection saves time by offering readily accessible and tested functions for a variety of standard operations, significantly enhancing programming efficiency and productivity.
Parameter Passing to C++ Functions
Parameter passing in C++ functions involves different methodologies: pass by value and pass by reference.
- Pass by Value
With pass by value, function parameters receive copies of the actual arguments, ensuring the original data remains unchanged within the function scope. Modifications performed on the parameter do not alter the original value, thereby preserving the integrity of the data.
- Pass by Reference
Pass by Reference method passes the memory address of the arguments, allowing direct manipulation of original data within the function. Changes made to the reference parameters reflect in the actual arguments, improving efficiency and reducing memory overhead.
C++ Function Definition
In C++, function definitions encapsulate specific tasks in named blocks, promoting code modularity and reusability. Creating a function encompasses defining its objective, setting parameters, and constructing the logic within the function body. This separation ensures the function can be conveniently invoked from various segments of the program, thereby improving code organization and readability.
Proper function definition is fundamental to harnessing the benefits of modularity and enhancing program structure in C++.
#include
using namespace std;
// Function Definition
void displayMessage() {
cout << "Hello, this is a user-defined function!" << endl;
}
int main() {
displayMessage(); // Calling the displayMessage function
return 0;
}
C++ Functions Using Pointers
In C++, functions using pointers enable direct memory manipulation. By passing memory addresses, functions access and modify original data, offering efficiency and reduced resource consumption. This method bypasses the need for creating additional copies, making it beneficial for managing large datasets and performing memory-level operations within functions.
Understanding pointer usage within functions is crucial for optimized memory handling and enhanced data manipulation.
#include
using namespace std;
void changeValue(int *ptr) {
*ptr = 30;
}
int main() {
int val = 20;
changeValue(&val);
cout << "Updated value: " << val << endl;
return 0;
}
Main C++ Function
In C++, the main function acts as the program's entry point, initiating the execution process.
- Without Parameters
The default 'main()' function, devoid of parameters, serves as the program's entry point, initializing the execution process. It orchestrates the program's tasks, controls its flow, and manages the application's logic.
#include
using namespace std;
int main() {
cout << "Main function without parameters" << endl;
return 0;
}
- With Parameters
The 'main()' function can accept command line arguments, enabling interaction between the program and the operating system. By receiving arguments during program execution, the 'main()' function adapts the program's behavior, customizing functionalities based on user inputs.
#include
using namespace std;
int main(int argc, char *argv[]) {
cout << "Arguments: " << argc << endl;
for (int i = 0; i < argc; ++i) {
cout << "Argument " << i << ": " << argv[i] << endl;
}
return 0;
}
Passing Array to C++ Function
In C++, when arrays are passed to functions, the array name is transformed into a pointer to the first element of the array. This transition allows functions to access and operate directly on the array's contents. This method facilitates efficient handling and manipulation of array elements within the program.
Manipulating arrays through functions enables the modification of array elements, calculations, sorting, or other operations without returning the modified array. The ability to pass arrays to functions streamlines code, as operations can be performed on array elements without creating multiple copies. Understanding how arrays are passed to functions using pointers is crucial for efficient array processing and optimized memory utilization in C++ programs.
C++ Overloading (Function)
C++ allows for two key types of overloading: function overloading and operator overloading.
- Function Overloading:
In function overloading, multiple functions share the same name but vary in the number or types of parameters they accept. This process allows these functions to execute different tasks based on the arguments provided. It enhances code readability and reduces redundancy by consolidating similar operations under a single function name.
- Operator Overloading:
Operator overloading in C++ enables the redefinition of operators like '+', '-', '*', and '/' for user-defined data types. By doing so, user-defined objects can utilize these operators, offering a more intuitive approach to handling these custom data types. This feature allows developers to create more flexible and expressive code using C++.
Advantages of Functions in C++
Functions in C++ offer numerous advantages crucial for efficient programming:
- Modularity: Functions decompose intricate operations into smaller, more manageable components, improving the organization and readability of code.
- Reusability: Functions allow for the reuse of the same logic across various sections in the program, diminishing redundancy and fostering efficiency.
- Simplified Maintenance: Segregating code into smaller, distinct parts enables easier debugging and maintenance.
- Abstraction: Functions abstract complex logic into simpler, callable units, improving code understanding and readability.
- Scalability: They provide a structured approach, allowing the addition of new functionalities or modifications without affecting the entire program.
Disadvantages of Functions in C++
In C++, functions, while pivotal, come with certain limitations:
- Complexity: Excessive function calls may lead to code complexity, reducing readability and understanding.
- Overhead: Function calls incur a slight performance overhead due to stack management and parameter passing.
- Memory Usage: Functions involve stack memory usage, potentially leading to stack overflow with deeply nested function calls.
- Maintenance Issues: Functions might pose maintenance challenges in large projects, especially if scattered across numerous files, leading to difficulties in tracking and debugging.
- Abstraction Complexity: Overuse of functions can create excessive abstractions, making the code more challenging to comprehend for newcomers.