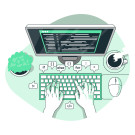
- 16th Nov 2023
- 22:35 pm
- Admin
In C++, control statements facilitate the execution flow of a program, enabling decision-making, looping, and altering control within code blocks. These statements guide the program's path based on certain conditions, ensuring a structured and controlled flow of instructions.
What are C++ Control Statements?
C++ Control Statements are pivotal in managing program flow, enabling decision-making, iteration, and control alterations within code blocks. These statements offer conditional, looping, and jump constructs, determining how a program progresses. Conditional statements evaluate conditions for execution, loops repeat code segments based on conditions, and jump statements alter control directly.
By incorporating these constructs, programmers gain the ability to structure and regulate program execution based on various conditions and criteria. Understanding and effectively utilizing these control statements is fundamental in creating robust and efficient C++ programs.
Types of Control Statements in C++
C++ incorporates various types of control statements, each fulfilling specific roles within programs. These encompass conditional, looping, and jump statements.
- Conditional Statements: These statements execute code blocks based on specified conditions, such as 'if', 'if-else', 'if-else-if', and 'switch'. They enable selective execution based on varying conditions.
- Looping Statements: Iterative in nature, looping statements repeat blocks of code until specified conditions are met. Common looping constructs include 'for', 'while', and 'do-while' loops.
- Jump Statements: Jump statements provide mechanisms to alter control flow. They encompass 'break', 'continue', and 'goto', allowing programmers to exit loops, skip code execution, and transfer control directly within a program. Comprehending these different types of control statements in C++ leads to efficient programming and logical control flow within the code.
Syntax of Control Statements in C++
Control statements in C++ are essential for structuring programs that can make decisions, iterate, and manage the flow of a program. These statements vary in their forms, with distinct syntax and purposes.
- If Statement
The 'if' statement is a fundamental structure used for conditional control in C++. It executes a block of code if a specified condition is true.
The syntax for the 'if' statement is as follows:
if (condition) {
// Code block to be executed if the condition is true
}
The 'if' statement allows for conditional execution of code based on a single condition. For instance:
int x = 10;
if (x > 5) {
cout << "x is greater than 5" << endl;
}
- Switch Statement
The 'switch' statement facilitates various execution paths according to the value of a variable or an expression. It evaluates an expression and directs the program to the corresponding 'case' depending on the evaluated value.
Here is the syntax for using the 'switch' statement:
switch (expression) {
case constant1:
// Code block
break;
case constant2:
// Code block
break;
default:
// Default code block
}
The 'switch' statement offers a means of branching based on different cases.
For example:
int choice = 2;
switch (choice) {
case 1:
cout << "Selected option 1" << endl;
break;
case 2:
cout << "Selected option 2" << endl;
break;
default:
cout << "Selected another option" << endl;
}
- For Loop
The 'for' loop is used to repeat a set of instructions for a particular count of iterations. It consists of three essential parts: initialization, condition, and increment/decrement.
The structure of the 'for' loop is showcased as follows:
for (initialization; condition; increment/decrement) {
// Code block to be executed
}
The 'for' loop is frequently used for traversing through a series of values. For example:
for (int i = 0; i < 5; i++) {
cout << i << " ";
}
- While Loop
The 'while' loop repeatedly executes a block of code as long as a specified condition holds true.
The 'while' loop syntax is showcased below:
while (condition) {
// Code block to be executed
}
The 'while' loop is beneficial for situations where a condition must be assessed before the execution of a block of code:
int count = 0;
while (count < 5) {
cout << count << " ";
count++;
}
- Do-While Loop
The 'do-while' loop runs a block of code at least once before checking the condition.
The structure of the 'do-while' loop is exemplified here:
do {
// Code block to be executed
} while (condition);
The 'do-while' loop guarantees that the code block executes at least once, regardless of the condition:
int num = 10;
do {
cout << num << " ";
num--;
} while (num > 0);
- Break Statement
The 'break' statement is utilized to exit from a loop or switch statement. It allows for an early exit from a loop's body.
For example:
for (int i = 0; i < 5; i++) {
if (i == 3) {
break; // Exit the loop when i equals 3
}
cout << i << " ";
}
- Continue Statement
The 'continue' statement is utilized to bypass the remaining code within a loop for the current iteration and move on to the subsequent iteration.
For example:
for (int i = 0; i < 5; i++) {
if (i == 2) {
continue; // Skip the current iteration when i equals 2
}
cout << i << " ";
}
- Goto Statement
The 'goto' statement is employed to move control to a labeled statement within a function.
The syntax for using the 'goto' statement is demonstrated below:
goto label;
// ...
label:
// Code block
While the 'goto' statement is available in C++, it is generally discouraged due to its potential to complicate program logic and readability. Other structured control statements like loops and conditionals are preferred in most cases.