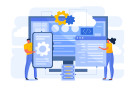
- 17th Nov 2023
- 00:07 am
- Admin
C++ arrays are collections of elements with the same data type, enabling the storage of multiple values under a single name. They're declared by defining the data type and array name, specifying the array's size in square brackets. Arrays facilitate easy access and traversal of elements through indexing. They offer an efficient means to manage and organize data in C++ programs.
Properties of Arrays in C++
In C++, arrays function as containers for elements of the same data type, offering ordered storage and easy access via index-based referencing. These structures store multiple values under a single identifier, allowing sequential storage and retrieval of data.
Arrays have a fixed size, defined at declaration, and are accessed via numeric indices, starting from zero. Their properties enable efficient manipulation and organization of data, making them fundamental components in C++ programming for handling collections of data elements.
Array Declaration in C++
In C++, array declaration involves specifying the data type and the array's identifier followed by square brackets enclosing the array size. For example, 'int numbers[5];' declares an integer array named 'numbers' with a size of 5. Arrays hold elements of the same data type and possess a fixed size determined at compile-time.
The declared size dictates the maximum number of elements the array can store, influencing memory allocation and the array's operational characteristics within the program.
#include
using namespace std;
int main() {
int numbers[5];
return 0;
}
Initialization of Array in C++
The various methods of array initialization in C++ cater to different scenarios and offer versatility. Understanding these techniques is crucial for initializing arrays with specific values, filling elements post-declaration using loops, or conveniently initializing arrays partially or entirely with zeros.
In C++, arrays can be initialized in multiple ways, allowing for quick and efficient population of array elements.
- Initialize Array with Values in C++:
Values can be directly assigned to the array elements during declaration, such as int numbers[3] = {1, 2, 3};.
- Initialize Array with Values and without Size in C++:
The size of the array can be omitted, and the compiler will infer the size from the number of initialization values.
- Initialize Array after Declaration (Using Loops):
Arrays can be filled with values after declaration, using loops like 'for' or 'while' to assign elements incrementally.
- Initialize an array partially in C++:
Portion of the array can be initialized, leaving the rest to be filled later.
- Initialize the array with zero in C++:
The array can be completely initialized with zeros, such as int numbers[5] = {0};.
int arr1[] = {1, 2, 3, 4, 5};
int arr2[3] = {10, 20, 30};
Accessing an Element of an Array in C++
Accessing elements within a C++ array involves utilizing index notation. For instance, 'arr[2]' accesses the third element in the array 'arr' (arrays are zero-indexed). Elements can be read, modified, or used within expressions by directly referencing their index.
Array elements are accessed and manipulated using square brackets, enabling precise targeting of individual elements, allowing data manipulation, comparison, or passing elements to functions as needed within a program.
int arr[] = {10, 20, 30};
int element = arr[1];
Traverse an Array in C++
Traversing an array in C++ entails iterating through its elements, typically using loops like 'for' or 'while'. Loop constructs allow sequential access to each array element using indices. For instance, 'for (int i = 0; i < size; ++i)' provides an effective means to traverse an array where 'size' represents the array's length.
This method enables the iteration through the array's elements, facilitating operations, such as reading, modifying, or performing computations on each element systematically. Traversing arrays is fundamental in many data manipulation and processing tasks within C++ programs.
int arr[] = {2, 4, 6, 8, 10};
for (int i = 0; i < 5; ++i) {
cout << arr[i] << " ";
}
Size of an Array in C++
In C++, determining the size of an array can be achieved through the 'sizeof' operator. For instance, 'sizeof(arr)' fetches the entire array's memory size in bytes.
By dividing the total memory occupied by a single element ('sizeof(arr[0])') into the array's total memory, the array size in terms of elements can be calculated. Yet, once an array is passed into a function, retrieving its size becomes challenging since arrays decay into pointers, losing their size information.
int numbers[7];
int size = sizeof(numbers) / sizeof(numbers[0]);
Passing Array to Function in C++
Passing arrays to functions in C++ can be accomplished through various methods:
- Passing Array as a Pointer:
Arrays are often passed as pointers to their first elements. The pointer can access the entire array, enabling changes directly within the function.
- Passing Array as an Unsized Array:
Arrays are passed without specifying their size, but a marker is used to signify the array's end. For instance, using a terminator like null or a separate argument to indicate the array's length.
void printUnsizedArray(int *arr) {
while (*arr != 0) {
cout << *arr++ << " ";
}
}
- Passing Array as a Sized Array:
Arrays can be passed to functions along with their size explicitly stated, permitting direct access and processing of the array elements within the function.
Multidimensional Arrays in C++
In C++, multidimensional arrays allow data organization in multiple dimensions. For example, a 2D array organizes elements in rows and columns, whereas a 3D array adds layers to rows and columns, creating a cuboid structure. These arrays are suitable for storing complex spatial data in programming.
#include
using namespace std;
int main() {
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
cout << "Element at [1][1]: " << matrix[1][1] << endl;
return 0;
}
Two-Dimensional Array in C++
In C++, a two-dimensional array comprises rows and columns, forming a grid-like structure. For instance, 'int matrix[2][3]' represents a 2x3 matrix, enabling element storage and access.
Each element is accessed using row and column indices, allowing the representation of matrices, tables, or grids within programming applications.
#include
using namespace std;
int main() {
int matrix[2][2] = {{1, 2}, {3, 4}};
cout << "Element at [0][1]: " << matrix[0][1] << endl;
return 0;
}
Three-Dimensional Array in C++
In C++, a three-dimensional array introduces an additional dimension to the structure, creating a cube-like array. For example, 'int cube[2][3][4]' declares a 3D array with two 'sheets,' each containing three 'rows' and four 'columns.'
Accessing elements in a 3D array involves specifying indices for sheets, rows, and columns to retrieve specific values.
#include
using namespace std;
int main() {
int cube[2][3][4] = {
{{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}},
{{13, 14, 15, 16}, {17, 18, 19, 20}, {21, 22, 23, 24}}
};
cout << "Element at [1][2][3]: " << cube[1][2][3] << endl;
return 0;
}
Advantages of using arrays in C++
Arrays offer several advantages in C++ programming:
- Data Organization: Arrays efficiently organize data of the same type, simplifying storage and access.
- Sequential Access: Elements within arrays are accessed sequentially via indexing, allowing for quick access and manipulation.
- Compact Storage: They facilitate the storage of multiple elements under a single name, conserving memory and enhancing data management.
- Iterative Operations: Arrays are conducive to looping structures, enabling iterative operations, like traversal and computation on array elements.
- Matrix Representation: Multidimensional arrays efficiently represent matrices and tables, essential for mathematical and structural operations.
Disadvantages of Arrays in C++
Arrays in C++ possess limitations, including:
- Fixed Size: Arrays have a fixed size determined at declaration, challenging dynamic memory requirements. Resizing or altering an array’s size during runtime isn’t straightforward.
- Memory Wastage: When an array has unused space, memory is wasted, impacting efficiency, especially when storage requirements fluctuate.
- Homogeneous Data: Arrays store elements of the same data type, limiting their adaptability for complex data structures with different data types.
- Lack of Bounds Checking: Arrays may lack automatic boundary checking, leading to potential overflow or underflow issues when not carefully managed.
- Inflexible Pointers: Pointers and arrays are closely related in C++, which can be both advantageous and limiting when handling arrays in complex scenarios.
Blog Author Profile - Radhika Joshi
Radhika Joshi is a seasoned programming expert with a profound academic background in Computer Science and Machine Learning. Her dedication to the field has been fueled by her relentless pursuit of knowledge and her commitment to pushing the boundaries of technology. PhD in Computer Science from a prestigious university in the United States. Her doctoral research focused on cutting-edge advancements in advanced machine learning algorithms and techniques.