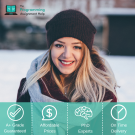
- 27th Oct 2023
- 20:48 pm
- Admin
What Is Breadth-First Search?
Breadth First Search (BFS) is an essential algorithm for systematically exploring graphs and networks. It's like mapping out a route, making it a critical tool in various applications such as web crawling, network analysis, and finding the shortest path.
BFS operates by starting at a central point and methodically examining its immediate connections before progressing to the next level of connections. It relies on a queue system to maintain the order of nodes to visit, ensuring a methodical exploration of options.
To understand and implement BFS effectively, resources such as "The Programming Assignment Help" provide valuable insights and guidance. Whether you're a student studying algorithms or a developer tackling real-world challenges, BFS is a crucial asset in your toolkit for efficient problem-solving and exploration.
Key Features of Breadth First Search (BFS)
Breadth First Search (BFS) is a graph traversal algorithm that systematically explores nodes in a graph. It is particularly useful for finding the shortest path and analyzing graph structures.
Here are some key points about BFS:
- Exploration Method: BFS explores a graph in a systematic manner, starting from the source node and moving to its neighbors before progressing to the next level of nodes.
- Queue Data Structure: It uses a queue to maintain the order of nodes to be visited, ensuring that nodes at the same level are processed before moving to the next level.
- Applications: BFS is widely used in various applications, including finding the shortest path in unweighted graphs, web crawling, social network analysis, and solving puzzles.
- Completeness: BFS is complete, meaning it can traverse all nodes in a connected graph or tree and find the shortest path if it exists.
- Memory Usage: While BFS guarantees optimality in finding the shortest path, it can consume more memory compared to Depth First Search (DFS), especially in large graphs.
- User-Friendly: Its systematic approach makes it easy to understand and implement, making it a valuable tool for both students and developers.
What Are Some BFS Examples Explained By Your Tutors?
Here are a few real-world examples of how the Breadth First Search (BFS) algorithm can be applied:
- Shortest Path in a Maze: BFS can be used to find the shortest path from a starting point to an exit in a maze. Each cell in the maze represents a node, and BFS helps explore and find the optimal route.
- Web Crawling: Search engines use BFS to crawl and index web pages. The web pages are represented as nodes, and BFS ensures that pages on the same level (in terms of links from the starting page) are explored before moving deeper into the web.
- Social Network Analysis: BFS can be used to analyze social networks. Starting from a person's profile, BFS explores connections (friends, friends of friends) in a social network to understand the network's structure or find the shortest path between individuals.
- Network Routing: In computer networking, BFS is applied to find the shortest path for data packets to traverse from one node to another within a network.
- Puzzle Solving: BFS is used to solve puzzles like the sliding puzzle, Rubik's Cube, or the Eight-Puzzle. It explores possible states or configurations to find a solution.
- Recommendation Systems: In recommendation systems, BFS can be used to explore user connections or preferences to make personalized recommendations.
- Geographical Mapping: BFS is employed to find the shortest routes between locations on a map. It's the basis for GPS and navigation systems.
- Software Testing: BFS can be used to systematically test software applications by exploring different states and inputs.
These examples demonstrate the versatility of BFS in solving a wide range of problems across various domains.
Explain the Implementation Of BFS In Python
Implementing the Breadth First Search (BFS) algorithm in Python is a common task. Here's a simple example of how to do it:
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def bfs(self, start):
visited = [False] * len(self.graph)
queue = []
result = []
visited[start] = True
queue.append(start)
while queue:
node = queue.pop(0)
result.append(node)
for neighbor in self.graph[node]:
if not visited[neighbor]:
visited[neighbor] = True
queue.append(neighbor)
return result
# Example usage:
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
start_node = 2
print("BFS starting from node", start_node, ":", g.bfs(start_node))
In this Python code, we create a Graph class that represents a graph using a defaultdict. It has methods for adding edges and performing BFS. Here's a step-by-step breakdown:
- Create a graph using a defaultdict.
- Add edges to the graph using the add_edge method.
- Implement the BFS method for performing BFS.
- Maintain a list visited to keep track of visited nodes and a queue for traversal.
- Start BFS from a given node, mark it as visited, and enqueue it.
- While the queue is not empty, dequeue a node, visit it, and enqueue its unvisited neighbors.
- Return the BFS result, which is a list of nodes visited in BFS order.
This code demonstrates a basic BFS implementation in Python. You can modify and expand upon it to suit your specific needs or use different data structures, depending on your application.
What Are The Applications of BFS?
Breadth First Search (BFS) is a versatile algorithm used in various applications across different fields. Some of the key applications of BFS include:
- Shortest Path Finding: BFS can find the shortest path between two nodes in an unweighted graph or maze, making it useful in navigation and route planning.
- Web Crawling: Search engines use BFS to systematically crawl and index web pages by following links from one page to another.
- Social Network Analysis: BFS helps analyze social networks by exploring connections between individuals, identifying clusters, or finding the shortest path between users.
- Network Routing: In computer networking, BFS can be employed to find the shortest path for data packets to traverse from one node to another within a network.
- Geographical Mapping: It is the basis for GPS and navigation systems, used to find the shortest routes between locations on a map.
- Puzzle Solving: BFS is used to solve various puzzles, such as sliding puzzles, mazes, Rubik's Cube, or the Eight-Puzzle, by exploring different states or configurations.
- Recommendation Systems: In recommendation engines, BFS can be used to explore user preferences and connections to make personalized recommendations for products, content, or friends.
- Software Testing: BFS is employed in software testing to systematically explore different states and inputs of an application, helping identify and fix bugs.
- Level Order Traversal in Trees: BFS is used to traverse and process all nodes at each level of a tree data structure, such as binary trees or multi-way trees.
- Connected Component Detection: BFS can find and label connected components in a graph, which is valuable in image processing and clustering algorithms.
- Game AI: BFS can be applied in game development for pathfinding and decision-making algorithms, ensuring characters or agents make informed moves.
- Bipartite Graph Testing: BFS can determine if a given graph is bipartite, which has applications in graph theory and network design.
These are just a few examples of the many applications of BFS. Its ability to systematically explore and analyze graphs and networks makes it a valuable tool in various domains, from computer science and data analysis to biology and game development.
The Programming Assignment Help offers valuable resources and assistance for students and developers seeking help with algorithms like Breadth First Search (BFS). With its experienced tutors and experts, the website provides guidance, tutorials, and practical examples to help users understand and implement BFS effectively. Whether you're a student looking to grasp the concept of BFS for assignments or a developer working on real-world applications, this resource can offer in-depth explanations and support. The programming assignment help website serves as a reliable platform for enhancing your knowledge and problem-solving skills related to BFS and various other programming and algorithmic concepts.