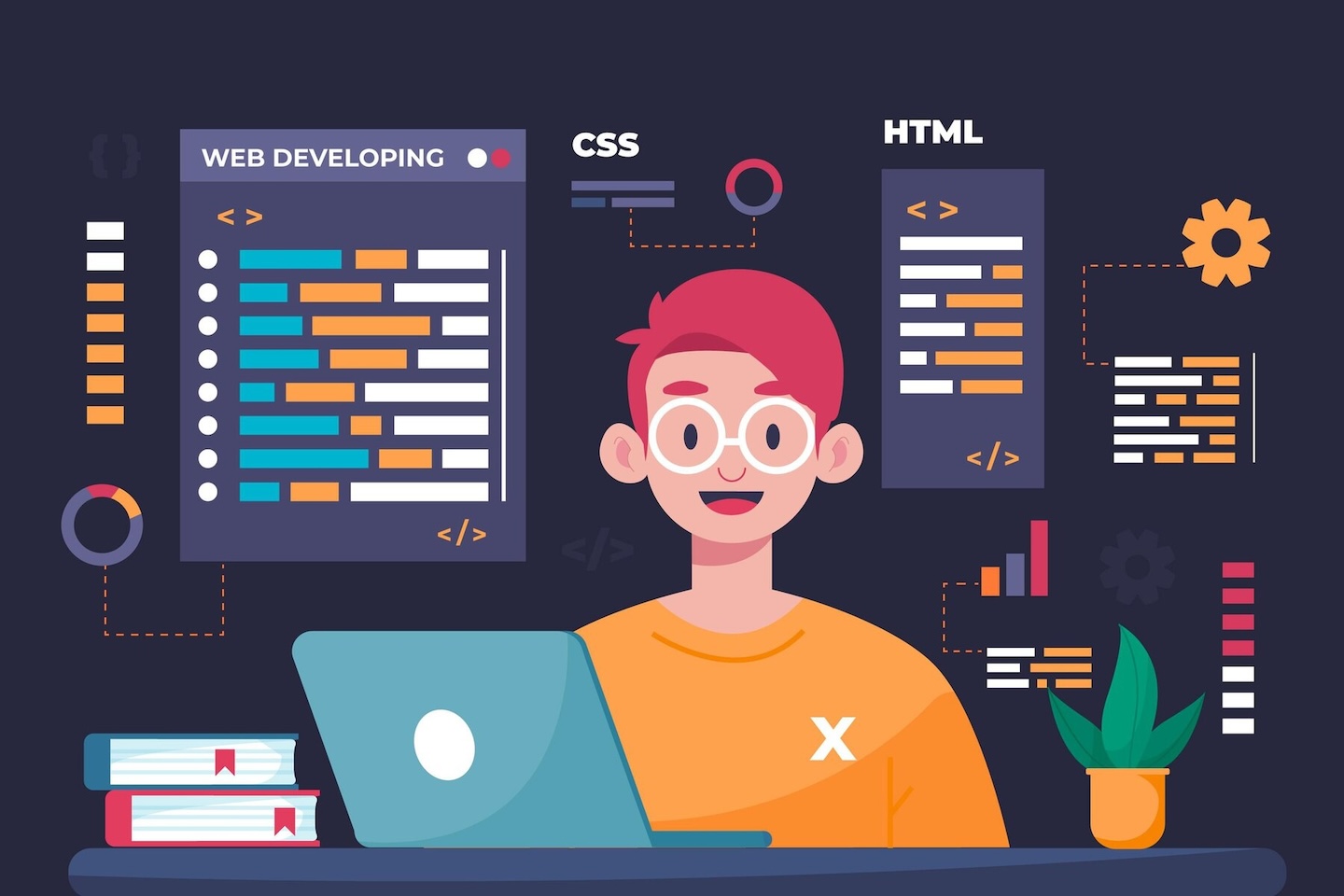
- 12th Dec 2023
- 16:22 pm
- Admin
Bootstrapping is a statistical resampling approach that uses sampling and replacement to generate several datasets, known as bootstrap samples, from a single dataset. This method enables statisticians and data scientists to evaluate a statistic's variability and distribution, determine confidence intervals, and draw sound predictions regarding population parameters. The bootstrapping process is easily accomplished in R using numerous functions and packages.
Important Bootstrapping Elements in R Programming:
- Bootstrap Sampling:
Bootstrapping entails randomly choosing samples from the original dataset with replacements. This method generates a number of bootstrap samples, each of the same size as the original dataset.
```
# Example of generating a bootstrap sample in R
original_data <- c(1, 2, 3, 4, 5)
bootstrap_sample <- sample(original_data, replace = TRUE, size = length(original_data))
```
- Statistic Calculation:
A statistic of interest (e.g., mean, median, standard deviation) is calculated for each bootstrap sample. This provides a distribution of the statistics.
```
# Example of calculating the mean from a bootstrap sample in R
mean_bootstrap <- mean(bootstrap_sample)
```
- Multiple Replications:
- The process of bootstrap sampling and statistic calculation is repeated a large number of times (e.g., thousands) to create a distribution of the statistic.
```
# Example of bootstrapping in R using the boot package
library(boot)
bootstrap_result <- boot(original_data, statistic = mean, R = 1000)
```
- Confidence Intervals:
- The resulting distribution is used to estimate confidence intervals for the statistic. Common percentiles (e.g., 2.5th and 97.5th) are often used to define confidence intervals.
```
# Example of calculating confidence intervals in R
ci <- quantile(bootstrap_result$t, c(0.025, 0.975))
```
When dealing with tiny sample sizes, non-normal distributions, or complex data structures, bootstrapping is a strong and adaptable method in statistical research. The procedure is made available in R via packages such as 'boot' and functions geared for resampling and statistical analysis.
Bootstrapping in R Programming
Bootstrapping in R has applications in many disciplines and is especially beneficial when dealing with complex data structures or when traditional statistical methods are constrained. Here are some examples of bootstrapping in R:
- Calculating Confidence Intervals:
Bootstrapping is a method for estimating confidence intervals for population metrics such as mean, median, and variance. When the underlying distribution is unknown or non-normal, this is extremely useful.
- Hypothesis Testing:
When the assumptions for parametric tests are not met, bootstrapping can be used to test hypotheses. It provides an empirical distribution for test statistics, allowing for more robust significance evaluations.
- Parameter Estimation in Regression Models:
Bootstrapping can help you estimate confidence ranges for regression coefficients and identify the uncertainty associated with model parameters. This is important when working with tiny sample numbers or when normalcy assumptions are called into doubt.
- Model Validation and Performance Evaluation:
In machine learning and predictive modeling, bootstrapping is used for model validation. It aids in determining the stability and repeatability of performance indicators like accuracy, precision, and recall across repeated bootstrap samples.
- Stability of Variable Selection:
Bootstrapping is used in regression or feature importance analysis to evaluate the stability of selected variables across multiple samples. This provides information about the robustness of the variables chosen.
- Creating Prediction Intervals:
In regression models, bootstrapping is used to create prediction intervals. Prediction intervals provide a range of feasible values for future observations while allowing for model uncertainty and data variability.
- Survey Data Analysis:
Bootstrapping is used in survey sampling to assess sample errors and construct confidence ranges for population parameters. This is especially true when working with intricate survey designs.
- Outlier Detection:
Bootstrapping aids in the identification of outliers in a dataset by evaluating the stability of observations across multiple bootstrap samples. This method is resistant to outliers, which can have a major impact on traditional statistical measures.
- Evaluating Skewed Distributions:
When dealing with skewed or non-normal distributions, bootstrapping offers a non-parametric alternative for estimating summary statistics and making population conclusions.
- Monte Carlo Integration:
Bootstrapping is a Monte Carlo approach used in numerical integration and simulation studies to estimate integrals or complex statistical quantities by resampling from observed data.
Bootstrapping in R is a versatile and powerful tool for dealing with statistical issues such as small sample sizes, non-parametric data, and breaches of distributional assumptions. Its adaptability and ease of use make it a significant tool for academics and data analysts from a variety of disciplines.
Performing Bootstrapping in R Programming
Bootstrapping in R includes several phases, which I'll walk you through using a basic example. In this example, we'll bootstrap the mean of the "mpg" variable using the built-in'mtcars' dataset.
- Step 1: Load the Dataset
Load the dataset you intend to analyze. In this case, we'll use the `mtcars` dataset.
```
# Load the mtcars dataset
data(mtcars)
```
- Step 2: Choose the Variable of Interest
Identify the variable for which you want to perform bootstrapping. In this example, we'll use the "mpg" variable.
```
# Select the variable of interest
variable_of_interest <- mtcars$mpg
```
- Step 3: Define the Bootstrapping Function
Create a custom function that generates a bootstrap sample and calculates the statistic of interest. In this case, we'll use the mean as our statistic.
```
# Define the bootstrapping function
bootstrap_function <- function(data) {
sample_data <- sample(data, replace = TRUE)
return(mean(sample_data))
}
```
- Step 4: Perform Bootstrapping
Use the `boot()` function from the `boot` package to perform bootstrapping. Specify the data, the bootstrapping function, and the number of bootstrap samples (`R`).
```
# Load the boot package
library(boot)
# Perform bootstrapping
bootstrap_result <- boot(data = variable_of_interest, statistic = bootstrap_function, R = 1000)
```
- Step 5: View the Results
Explore the results of the bootstrapping analysis. The `boot` object contains various components, including the bootstrap replicates and confidence intervals.
```
# View the results
print(bootstrap_result)
```
- Step 6: Visualize the Results (Optional)
You can create visualizations to better understand the distribution of the bootstrap replicates and the confidence intervals.
```
# Plot the distribution of bootstrap replicates
hist(bootstrap_result$t, main = "Bootstrap Distribution of Mean", xlab = "Mean")
```
- Step 7: Interpret the Results
Examine the results, including the point estimate (e.g., mean), confidence intervals, and other relevant statistics. The `boot.ci()` function can be used to calculate various types of confidence intervals.
```
# Calculate and view confidence intervals
boot_ci <- boot.ci(bootstrap_result, type = "basic")
print(boot_ci)
```
These instructions cover the fundamentals of bootstrapping in R. The specifics will rely on the statistical analysis you are performing and the nature of your data. Bootstrapping is a versatile strategy that may be applied to a variety of circumstances and research concerns.