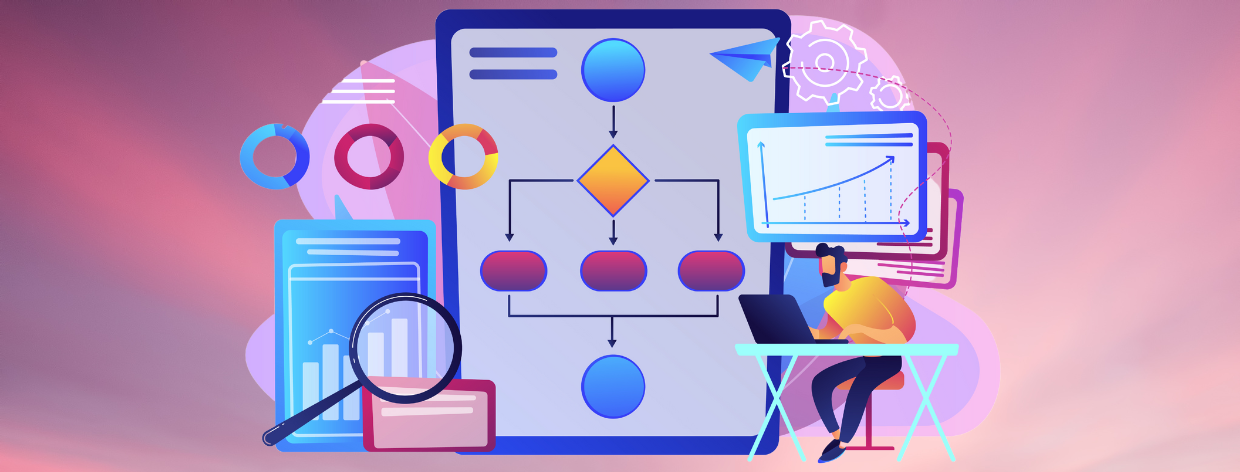
- 23rd Feb 2024
- 23:35 pm
The concept of the average, a single value representing a set of data points, plays a crucial role in various fields. From evaluating academic performance to understanding economic changes, it offers a concise summary of the central tendency within a dataset. Beyond statistics, averages inform scientific discoveries, guide financial decisions, and facilitate everyday comparisons.
Fortunately, the versatile programming language Python simplifies calculating averages. Whether you're a seasoned data analyst or a curious beginner, the built-in functions by Python and its powerful libraries are ranging over methods that are efficient. No more of those tricky manual calculations and intricate formulas.
With our Python Assignment Help and Python Homework Help service, we delves into the realm of finding averages in Python. We'll explore various approaches, from the basic sum-and-divide method to the advanced capabilities of NumPy functions. Our exploration will extend to specialized averages like medians and modes, equipping you to tackle diverse data analysis challenges with confidence.
Embark on this computational journey with us as we demystify the average, empower you with Python's tools, and unlock a world of possibilities in data exploration.
Understanding Averages: Mean, Median, and Mode in Python
In data analysis, averages reign supreme for summarizing and interpreting numerical data. But beyond the blanket term "average," distinct approaches exist, each with its strengths and use cases. This exploration delves into the mean, median, and mode, guiding their appropriate usage in Python.
- Mean: The familiar "average," calculated by summing all values and dividing by the total count. Python's statistics.mean() simplifies this. Suitable for normally distributed data, but susceptible to outliers.
- Median: The "middle number" when values are arranged in ascending or descending order. Python's statistics.median() provides the value. Robust against outliers, making it useful for skewed datasets.
- Mode: Identifies the most frequent value within a dataset. Python's statistics.mode() helps you discover it. Valuable for understanding common values but can have multiple modes or none at all.
Choosing the right average depends on your data and desired information. Would be working perfectly in the case of normal distributions, not working for skewed data, when the median would be preferable. When identifying common values, use the mode. Here, we have demonstrated how—with such knowledge and through Python tools—one can escape common pitfalls while gaining the full power of averages for deeper insights from your data.
Methods for Finding Average in Python
Python, with its versatile built-in functions and powerful libraries, offers a treasure trove of options for calculating different types of averages. This exploration delves into the world of built-in functions and NumPy, empowering you to choose the right tool for your specific needs.
Using sum() and len() in Python
This fundamental duo, sum() and len(), offers a straightforward approach, particularly for smaller datasets. While basic, it requires manual division by the list length:
data = [10, 20, 30]
average_sum = sum(data) / len(data)
print("Average using sum() and len():", average_sum)
# Output: 20.0
Remember, for empty lists, this method raises a ZeroDivisionError.
Advantages:
- Simple and easy to understand, especially for beginners.
- No external libraries required.
Disadvantages:
- Requires manual division, which can be error-prone.
- Not suitable for empty lists (raises ZeroDivisionError).
- Less efficient for large datasets.
Using reduce() and lambda in Python
For a more compact approach, reduce() and lambda offer a one-liner solution:
from functools import reduce
average_reduce = reduce(lambda x, y: x + y, data) / len(data)
print("Average using reduce() and lambda:", average_reduce)
# Output: 20.0
While concise, this method might be less intuitive for beginners due to its functional programming style.
Advantages:
- Highly concise one-line solution.
- Can be more efficient for memory usage than iteration.
Disadvantages:
- Less readable and intuitive for beginners due to functional programming style.
- Requires importing functools.
- Not as well-suited for custom calculations or edge cases.
Using Python mean()
Enter statistics.mean(), the champion for most cases. It provides a clear, concise, and robust way to calculate the mean, handling edge cases like empty lists gracefully:
import statistics
data = [10, 20, 30, 40]
average_mean = statistics.mean(data)
print("Average using mean():", average_mean)
# Output: 25.0
Beyond the mean, statistics offers functions for median and mode, making it a versatile choice.
Advantages:
- Clear, concise, and robust implementation.
- Handles edge cases gracefully (e.g., empty lists).
- Offers functions for median and mode beyond the mean.
Disadvantages:
- Might be slightly less efficient than numpy.mean() for very large datasets.
Using iterating List in Python
If you need more control or custom calculations, iterating over the list elements provides flexibility:
total = 0
for num in data:
total += num
average_iterate = total / len(data)
print("Average using iterating list:", average_iterate)
# Output: 25.0
While adaptable, this method can be less efficient for large datasets.
Advantages:
- Offers maximum flexibility for custom calculations and logic.
- Easy to understand and implement.
Disadvantages:
- Can be less efficient than vectorized operations for large datasets.
- More verbose code compared to other methods.
Using Python numpy.average() function
For massive datasets, NumPy reigns supreme. Its vectorized operations offer significant performance gains:
import numpy as np
data = np.array([10, 20, 30, 40])
average_numpy = np.mean(data)
print("Average using numpy.average():", average_numpy)
# Output: 25.0
NumPy also provides functions for various other averages and statistics, making it a powerful tool for large-scale data analysis.
Advantages:
- Highly efficient for large datasets due to vectorized operations.
- Offers various statistical functions beyond basic averages.
- Powerful for numerical computations in general.
Disadvantages:
- Requires importing the NumPy library.
- Might have a steeper learning curve for beginners unfamiliar with NumPy.
Using List comprehension
If you prefer a concise and readable approach similar to sum() and len(), consider list comprehension:
average_list_comprehension = sum(data) / len(data)
print("Average using list comprehension:", average_list_comprehension)
# Output: 20.0
Remember, similar to the basic method, this approach requires handling empty lists separately.
Advantages:
- Concise and readable syntax similar to sum() and len().
- Efficient memory usage compared to iteration.
Disadvantages:
- Requires handling empty lists separately (similar to sum() and len()).
- Less flexible for custom calculations compared to iteration.
Applications of Average List in Python
In the data analysis lies the humble average, a powerful tool for summarizing and interpreting numerical information. Python, with its diverse toolkit, offers a plethora of methods to calculate various averages, each finding its application in different scenarios. Let's delve into some key use cases:
Analyzing Central Tendency:
- Mean: The most common average, useful for understanding the "typical" value in a dataset. Helps identify datasets that deviate significantly from the norm.
- Median: Robust against outliers, ideal for skewed data where the mean might be misleading. Useful for measuring income levels or performance metrics.
Tracking Performance and Trends:
- Moving Average: Smoothes out fluctuations, revealing underlying trends in time-series data. Used in finance to analyze stock prices or in marketing to track website traffic.
- Weighted Average: Assigns different weights to data points based on their importance. Used in grading systems to consider varying difficulty levels or in sports to calculate weighted team averages.
Identifying Patterns and Distributions:
- Mode: Represents the most frequent value, helpful for understanding common preferences or product choices. Used in market research to identify popular features or in linguistics to analyze word frequency.
- Percentiles: Divide data into equal-sized groups, revealing the distribution of values. Used in risk analysis to assess financial stability or in education to compare student performance.
Machine Learning and Data Science:
- Averages in Cost Functions: Used in algorithms like linear regression to minimize the difference between predicted and actual values.
- Feature Engineering: Creating new features based on combinations of averages can improve model performance.
Everyday Applications:
- Calculating average household income, exam scores, or product ratings.
- Estimating travel time based on average speed limits.
- Comparing average temperatures across different regions.