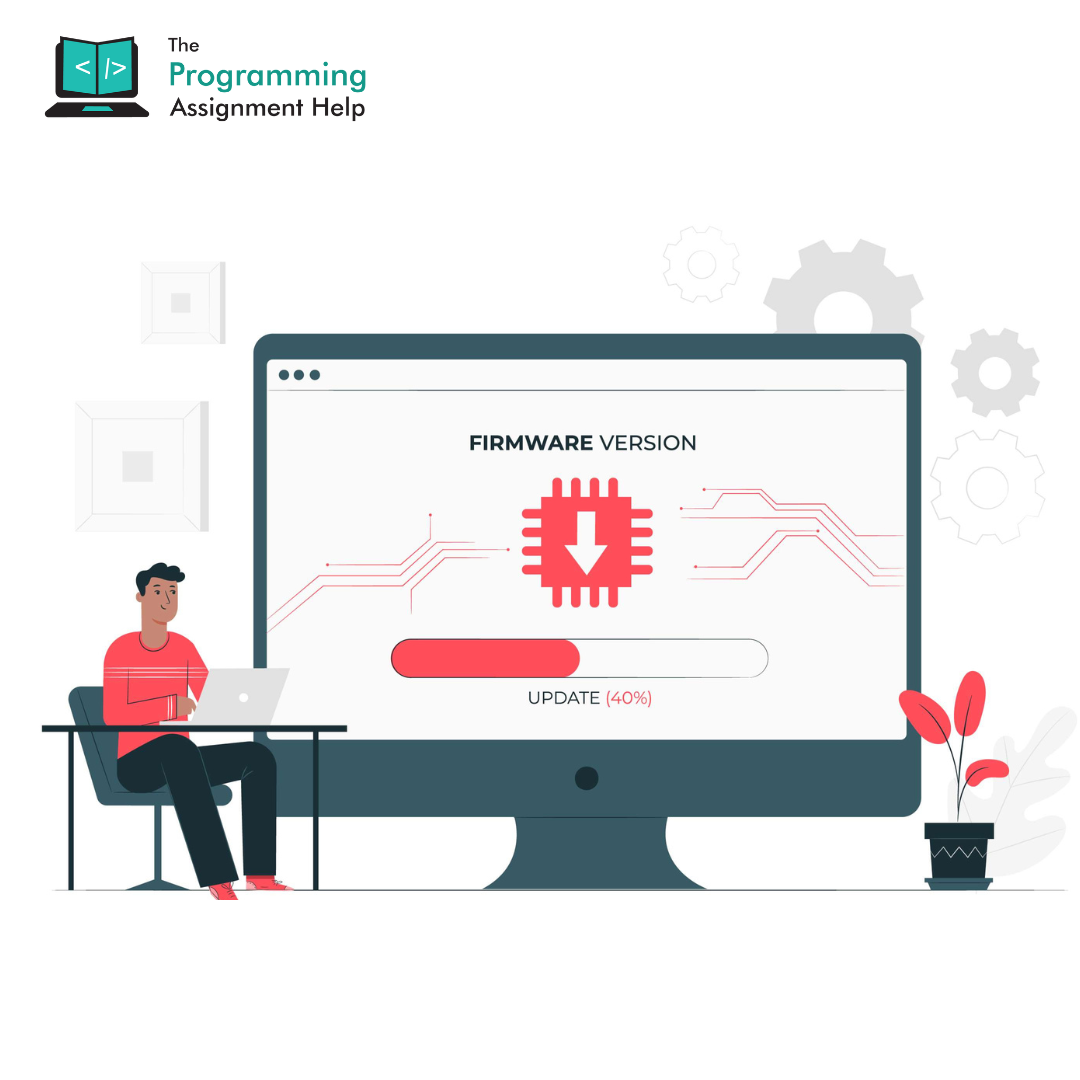
- 29th Dec 2022
- 03:05 am
- Admin
Arduino Assignment Help
Design a simple Arduino program using Atmel Studio to control an LED connected to Pin 13. The LED should blink ON and OFF at a specific interval. The assignment must be implemented without plagiarism, and all code and explanations will be original.
Programming Assignment Solution:
Step 1: Set up the Hardware Connect an LED to Pin 13 on your Arduino board. The longer leg (anode) should be connected to Pin 13, and the shorter leg (cathode) should be connected to GND.
Step 2: Download and Install Atmel Studio If you haven't already, download and install Atmel Studio from the official website. Ensure you have the necessary drivers and tools installed for your specific Arduino board.
Step 3: Create a New Project Open Atmel Studio and create a new project. Select "GCC C Executable Project" as the project type and choose your specific Arduino board as the target device. Give your project a name and location.
Step 4: Write the Arduino Code In Atmel Studio, open the main.c file (or the file with the main code). Write the code for blinking the LED as follows:
#include
#include
#define LED_PIN 13
int main(void)
{
// Set Pin 13 as an output pin
DDRB |= (1 << LED_PIN);
while (1)
{
// Turn ON the LED by setting Pin 13 high
PORTB |= (1 << LED_PIN);
// Delay for a specific time (e.g., 500ms)
_delay_ms(500);
// Turn OFF the LED by setting Pin 13 low
PORTB &= ~(1 << LED_PIN);
// Delay for a specific time (e.g., 500ms)
_delay_ms(500);
}
return 0;
}
Step 5: Build and Program the Arduino Now, click on the "Build" button in Atmel Studio to compile the code. Ensure there are no errors during compilation. Connect your Arduino board to your computer using a USB cable. Then, click on the "Device Programming" option under the "Tools" menu. In the Device Programming dialog, select your Arduino board and the communication port. Click on "Apply" to connect to the board. Finally, click on "Program" to upload the compiled code to the Arduino board.
Step 6: Test the LED Blinking Once the code is uploaded successfully, the LED should start blinking ON and OFF at a 500ms interval. You can adjust the blinking interval by changing the delay time in the code.