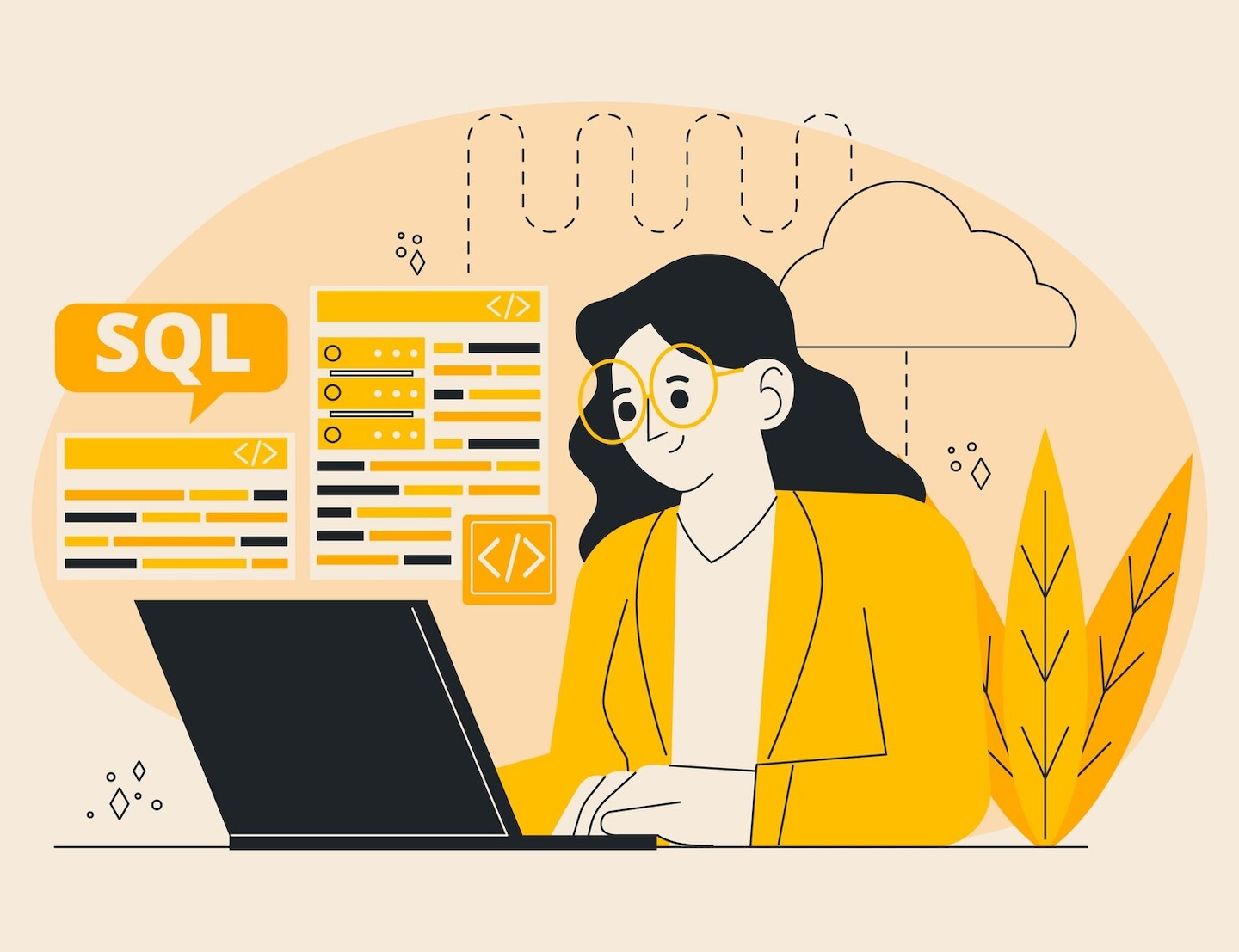
- 11th Dec 2023
- 23:20 pm
- Admin
SQL statements that are more complex and advanced than the usual SELECT, INSERT, UPDATE, and DELETE actions are referred to as advanced SQL queries. To retrieve, edit, and analyse data, these queries make full use of advanced SQL capabilities and procedures.
Here are some of the most critical elements of sophisticated SQL queries.
- Participate In Operations:
Complex queries frequently involve several tables, necessitating the use of JOIN methods to aggregate data from various sources. INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN are popular JOIN operations used to extract data based on table relationships.
```
SELECT employees.employee_id, employees.employee_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
```
- Subqueries:
Subqueries are queries that are contained within another query. They can be used in clauses like as SELECT, FROM, WHERE, and HAVING. Subqueries can be correlated or uncorrelated, and they are used for filtering, aggregating, and performing comparisons.
```
SELECT employee_name
FROM employees
WHERE department_id IN (SELECT department_id FROM departments WHERE department_name = 'IT');
```
- Aggregation Functions:
Advanced SQL queries frequently employ aggregate functions to conduct calculations on sets of numbers, such as COUNT, SUM, AVG, MIN, and MAX. These functions are frequently combined with GROUP BY clauses.
```
SELECT department_id, COUNT(*) as employee_count
FROM employees
GROUP BY department_id;
```
- Window Functions:
Window functions are used to do computations across rows without collapsing the result set and work on a collection of rows related to the current row. ROW_NUMBER, RANK, DENSE_RANK, and LAG are examples of common window functions.
```
SELECT employee_name, salary,
ROW_NUMBER() OVER (PARTITION BY department_id ORDER BY salary DESC) as rank
FROM employees;
```
- Common Table Expressions (CTEs):
CTEs allow you to define temporary result sets within your query, which improves readability and maintainability. They are defined with the WITH clause and can be referred to later in the query.
```
WITH high_salary_employees AS (
SELECT employee_name, salary
FROM employees
WHERE salary > 80000
)
SELECT * FROM high_salary_employees;
```
- CASE Statements:
The CASE statement is used to provide conditional logic within a query. It is frequently used to generate derived columns, custom aggregations, or transform data based on predefined parameters.
```
SELECT employee_name,
CASE
WHEN salary > 100000 THEN 'High Salary'
WHEN salary > 50000 THEN 'Medium Salary'
ELSE 'Low Salary'
END AS salary_category
FROM employees;
```
Advanced SQL queries are a powerful and versatile approach for developers and analysts to interact with relational databases, allowing them to access and alter data in sophisticated ways. These queries are critical for dealing with complex data sets, analysing trends, and extracting useful information from databases.
Uses cases
Advanced SQL queries are useful in situations where the intricacy of data collection, modification, and analysis necessitates more than basic SELECT, INSERT, UPDATE, and DELETE procedures. Here are some examples of frequent sophisticated SQL query use cases:
- Reporting on Business Intelligence (BI): Organisations frequently require complicated reports in BI applications that entail aggregations, filters, and calculations. Advanced SQL queries including aggregate functions, JOINs, and subqueries enable valuable insights to be extracted from enormous datasets.
- Data Warehousing: Data warehouses aggregate and organise data from various sources for analysis. Advanced SQL queries are required for data transformation and combining across numerous tables, handling complex relationships, and constructing summary tables for efficient reporting.
- Complex Filtering and Searching: Advanced SQL queries with conditions, JOINs, and subqueries can help extract specific subsets of data based on complicated criteria in applications where users need to execute advanced searches or apply complex filters.
- Data Migration and Transformation: Advanced SQL queries are used to map, convert, and purify data during data transfer or transformation procedures. This involves things like changing data types, converting values, and ensuring data integrity across several systems.
- Cleaning and Validation of Data: Data cleaning and validation are critical processes in ensuring data quality. Advanced SQL queries aid in the detection and correction of data anomalies such as duplicates, missing values, and inconsistencies, hence maintaining data accuracy and reliability.
- Time Series Analysis: Advanced SQL queries with window functions can be used to generate moving averages, discover patterns, and perform time-based comparisons when working with time-series data. Financial analysis, stock market patterns, and other time-dependent datasets are examples of this.
- Hierarchical Data: Advanced SQL queries employing recursive common table expressions (CTEs) or hierarchical query methods help browse and retrieve hierarchical relationships effectively in systems that contain hierarchical data, such as organisational structures or threaded conversations.
- User Authentication and Authorization: Advanced SQL queries are used in applications with user authentication and authorization systems to validate user credentials, check access permissions, and retrieve user-related information based on complicated access control rules.
- Data Enrichment: Using advanced SQL queries to combine data from multiple sources, advanced SQL queries are frequently used to enrich datasets. JOIN actions on tables with foreign keys, adding external data via subqueries, and merging datasets to provide comprehensive representations of information are all examples of this.
Application Dynamic Data Generation:
Applications that demand dynamic and personalised content frequently employ complex SQL queries to retrieve and deliver data that is suited to the user's preferences. Personalised suggestions, user-specific dashboards, and dynamic content development based on user interactions are all examples of this.
These use cases demonstrate the power of sophisticated SQL queries in dealing with difficult problems in a variety of fields, including business, analytics, data management, and application development. Organisations may draw useful insights, assure data quality, and construct sophisticated applications that fit unique business requirements by leveraging powerful SQL features.
Some Advanced SQL queries
Query Type | Description |
JOIN Operations | Combines rows from two or more tables based on a related column. Types include INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN. |
Subqueries | Embeds one query within another. Subqueries can be used in SELECT, FROM, WHERE, and HAVING clauses. Commonly used for filtering, aggregation, or comparison operations. |
Aggregation Functions | Performs calculations on sets of values. Common aggregate functions include COUNT, SUM, AVG, MIN, and MAX. Used with the GROUP BY clause for grouped aggregations. |
Window Functions | Operates on a set of rows related to the current row. Examples include ROW_NUMBER(), RANK(), DENSE_RANK(), and LAG(). Useful for performing calculations across rows without collapsing the result set. |
Common Table Expressions (CTEs) | Creates a temporary result set within a query for better readability and maintainability. Defined using the WITH clause and can be referenced in subsequent parts of the query. |
CASE Statements | Provides conditional logic within a query. Used for creating derived columns, custom aggregations, or transforming data based on specified conditions. |
Self Joins | Involves joining a table with itself. Commonly used when working with hierarchical data or to compare rows within the same table. |
Unions and Intersections | Combines or intersects the results of two or more SELECT statements. UNION returns distinct rows, while INTERSECT returns common rows. |
Pivot and Unpivot | Transforms data from rows to columns (Pivot) or from columns to rows (Unpivot). Useful for reshaping data for reporting or analysis purposes. |
Recursive Queries |
Involves querying hierarchical or recursive data. Typically used with recursive common table expressions (CTEs) to navigate and retrieve data from a table with a parent-child relationship. |
These advanced SQL queries cover a wide range of features, from data aggregation and transformation to dealing with complex relationships and hierarchical systems. Understanding and mastering these queries enables database engineers and analysts to perform complex data operations on relational databases and get deeper insights.