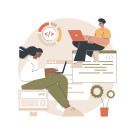
- 14th Nov 2023
- 20:42 pm
- Admin
Abstraction in Java is a cornerstone that simplifies complex programming structures, focusing on presenting a clear model while concealing implementation intricacies. This fundamental principle streamlines code and emphasizes critical features without revealing all the underlying complexities of the system's inner workings.
What is Abstraction in Java?
Abstraction in Java is the principle of hiding complex implementation details while presenting only the necessary features to users. It is the process of creating more manageable code by removing unnecessary complexity and emphasizing the relevant aspects for the end-users. By employing abstraction, developers can focus on building high-level logic, enhancing code reusability, and ensuring scalability.
In Java, abstraction simplifies the way programming is approached. It promotes the creation of clean and understandable code by showcasing only the required features while hiding the underlying complexities. This process is essential in large-scale projects where several developers collaborate, ensuring the code is organized, clear, and comprehensible. It also enhances the adaptability of the software, providing the flexibility to modify and extend functionality without altering the primary architecture. Abstraction in Java serves as a fundamental building block for constructing efficient and maintainable software solutions.
Types Of Abstraction In Java
Abstraction in Java can be categorized into two main types: data abstraction and control abstraction.
- Data Abstraction: This type emphasizes displaying only the relevant data and hiding the unnecessary implementation details. It's achieved through abstract data types or classes. By defining methods in a class, developers can access and modify the attributes while keeping the internal implementation hidden.
- Control Abstraction: Control abstraction focuses on simplifying code by utilizing functions, methods, or procedures to execute specific tasks or control flows. It involves breaking down complex procedures into manageable tasks, thus enhancing code readability and maintainability by using loops, conditional statements, and modular programming concepts.
Java Abstract Class
An abstract class in Java is a blueprint or a partially defined class that cannot be instantiated on its own. It's intended to be extended by subclasses that implement the abstract methods it contains. Abstract classes possess a mix of complete and incomplete methods, including abstract methods without any implementation details, as well as defined methods. These classes serve as a foundation for other classes to inherit common behavior or characteristics. They provide structure to the application's design while allowing specific methods to be defined in the child classes that extend the abstract class.
- Definition: An abstract class in Java is declared using the abstract keyword and cannot be instantiated.
- Characteristics: Abstract classes may contain abstract methods and concrete methods.
- Purpose: They offer a blueprint for other classes and provide common functionalities for derived classes.
- Usage: When developers need a base class to inherit from or extend to create more specific classes.
abstract class Shape {
abstract void draw();
}
class Circle extends Shape {
void draw() {
System.out.println("Drawing a circle");
}
}
class Rectangle extends Shape {
void draw() {
System.out.println("Drawing a rectangle");
}
}
public class Main {
public static void main(String[] args) {
Shape circle = new Circle();
circle.draw();
Shape rectangle = new Rectangle();
rectangle.draw();
}
}
Java Abstract methods
Abstract methods in Java are methods that are declared without an implementation in the abstract class. These methods lack a body and are designed to be overridden by the subclasses that extend the abstract class. They serve as a contract that specifies what the method should do, allowing child classes to provide their own unique implementation. Abstract methods define a method signature but lack the actual code to perform the specific function. These abstract methods play a key role in enforcing consistency across the class hierarchy while allowing different subclasses to implement these methods according to their unique requirements.
- Definition: Abstract methods in Java are declared without a body and marked with the abstract keyword.
- Characteristics: Abstract classes in Java do not contain any implementation details and are required to be overridden in the derived classes to provide specific implementations.
- Purpose: They provide a structure for derived classes to define their unique implementations.
- Usage: Ideal for methods that need to be defined by specific subclasses while retaining their generalized behavior.
abstract class Animal {
abstract void makeSound();
}
class Dog extends Animal {
void makeSound() {
System.out.println("Woof Woof!");
}
}
class Cat extends Animal {
void makeSound() {
System.out.println("Meow Meow!");
}
}
public class Main {
public static void main(String[] args) {
Animal dog = new Dog();
Animal cat = new Cat();
dog.makeSound();
cat.makeSound();
}
}
Abstract Class And Interface
Abstract classes and interfaces are fundamental components in Java programming. They serve as templates or models that guide subclasses to implement specific functions and behaviors. Abstract classes allow the declaration of abstract methods alongside concrete methods, and they can't be instantiated on their own. They act as a foundation for child classes to inherit common functionalities while also enforcing the implementation of abstract methods. Interfaces, on the other hand, define a contract where all methods are abstract by default. Multiple interfaces can be implemented by a single class, enabling a class to fulfill the requirements of various interfaces. They serve as a way to achieve multiple inheritances in Java.
- Abstract Class: An abstract class can have both abstract and concrete methods and cannot be instantiated. Subclasses extend it to define specific behaviors.
- Interface: An interface contains only abstract methods and defines a contract that concrete classes must adhere to.
- Differences: Abstract classes can offer some implementation, whereas an interface solely outlines a contract without implementing any behavior.
- Usage: Abstract classes are employed when a default behavior is needed, while interfaces are advantageous for cases requiring multiple inheritances or when a class must adhere to a contract.
Algorithm to implement abstraction in Java
Abstraction in Java simplifies complex real-world entities into their essential and relevant features. It enables programmers to build sophisticated systems by hiding irrelevant details and showcasing the essential components.
In Java, abstract classes and methods enable developers to create guidelines that shape the structure and behavior of derived classes. This approach facilitates modularity, enhances code reusability, and maintains a clear separation between interface and implementation.
The process of abstraction leads to the creation of well-defined, understandable, and scalable systems, ultimately streamlining the software development process in Java.
Advantages of Abstraction in Java
The advantages of abstraction in Java are numerous. It provides a clear distinction between the interface and implementation, enhancing code readability and maintenance.
- Distinct Interface and Implementation: Abstraction provides a clear separation between interface and implementation for better readability and maintenance.
- Reusability of Code: It enables developers to inherit and implement common features across multiple classes, enhancing code reusability.
- Simplification of Complexity: Abstracting essentials while hiding unnecessary details simplifies complex systems, making them easier to understand and maintain.
- Improved Modularity: Abstraction supports modularity, aiding in flexible design and easier adaptation to changing business needs.
- Security Enhancement: It assists in concealing sensitive information, contributing to the creation of more secure software architectures.
Disadvantages of Abstraction in Java
Here are some disadvantages of abstraction in Java:
- Complexity in Learning: The concept of abstraction sometimes introduces complexities for new developers learning Java due to its abstract nature.
- Performance Overhead: Excessive abstraction could sometimes lead to performance overhead, as it might introduce additional layers that impact execution time.
- Increased Code Volume: Extensive use of abstraction can lead to an increase in the overall lines of code, which may potentially increase code maintenance efforts.
- Difficulty in Tracking Changes: Overuse of abstraction might make it challenging to track changes, especially when dealing with a large number of abstractions across the codebase.
- Design Overhead: In certain cases, creating abstract classes and interfaces might add design complexity, making it harder to manage the codebase.
About The Author - Janhavi Gupta
Seasoned programmer with 6 years of experience in designing, developing, and deploying software solutions. Strong problem-solving skills coupled with the ability to collaborate effectively in multidisciplinary teams. Continuously learning and adapting to new technologies and methodologies to drive innovation and deliver high-quality, user-centric solutions.