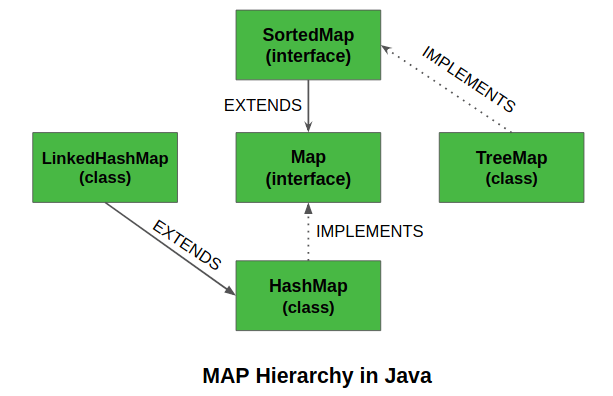
- 5th Jun 2019
- 05:01 am
- Ali Akavis
Java Program - Question
Remove the linked lists from project 3 and use a HashMap and TreeMap to store the Box objects. Read a line from the input file, instantiate a new Box (check for exceptions), and insert the Box into a HashMap and into a TreeMap.
Print the results of the stored HashMap in the left column of the grid layout, and the results of the stored TreeMap in the right column of the grid layout. The TreeMap results should come out in sorted order.
Java Program - Solution
Public Class Box
public class Box { private int length; private int width; private int height; public Box () { } //constructor public Box (int l, int w, int h) { if(l < 1 || w < 1 || h < 1) { throw new IllegalBoxException("The measurement for the box are invalid "); } else { this.length = l; this.width = w; this.height = h; } } //set length method public void setLength(int l) { if(l > 0) { this.length = l; } else { this.length = 1; } } //set width method public void setWidth(int w) { if(w > 0) { width = w; } else { width = 1; } } //set height method public void setHeight(int h) { if(h > 0) { height = h; } else { height = 1; } } //calculate volume method public int getvolume() { return length *width *height; } //get length method public int getLength() { return length; } //get width method public int getWidth() { return width; } //get height public int getHeight() { return height; } public String toString() { return String.format("L: %d, W: %d, H:%d (V: %d)", getLength(), getWidth(), getHeight(),getvolume()); } }
Box GUI
import java.awt.GridLayout; import java.util.Map; import javax.swing.JFrame; import javax.swing.JTextArea; @SuppressWarnings("serial") public class BoxGUI extends JFrame { private JTextArea leftTextArea=new JTextArea(10,10); private JTextArea rightTextArea=new JTextArea(10,10); public BoxGUI(Map hashmap, Map treemap) { setTitle("Box"); setSize(350, 350); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLayout(new GridLayout(1, 2,20,20)); String data = "HASH MAP\n\n"; //Title for Unsorted Boxes for(Map.Entry entry : hashmap.entrySet()) { data+= entry.getValue().toString() + "\n"; } leftTextArea.setText(data); add(leftTextArea); rightTextArea.setEditable(false); data = "TREE MAP\n\n"; for(Map.Entry entry : treemap.entrySet()) { data+= entry.getValue().toString() + "\n"; } rightTextArea.setText(data); add(rightTextArea); setVisible(true); pack(); } }
Illegal Box Exception
/** * Title: IllegalBoxException Class * * Description: Exception Class for use by all Box classes */ @SuppressWarnings("serial") public class IllegalBoxException extends RuntimeException{ /** * Initializes an ElementNotFoundException storing an appropriate * message along with the type of the collection (as specified by the user). */ public IllegalBoxException (String collection) { super("The measurement for the box are invalid " + collection); } }
Project Solution
import java.io.IOException; import java.nio.charset.Charset; import java.nio.file.Files; import java.nio.file.Paths; import java.util.HashMap; import java.util.Map; import java.util.Scanner; import java.util.TreeMap; import javax.swing.JOptionPane; import javax.swing.JScrollPane; import javax.swing.JTextArea; public class Project4 { public static void main(String[] args) { //the filepath needs to be manually updated depending on the placement of the file and OS String filename="/Users/nirav/eclipse-workspace/Project3/boxes.txt"; //String filename="boxes.txt"; Scanner filescanner=null; String filecontent=""; int size; int i=0; try { filecontent=readFile(filename,Charset.defaultCharset()); String rows[]=filecontent.split("\n"); size = rows.length; Map hashmap = new HashMap<>(); Map treemap = new TreeMap<>(); while(i { try { String data[]=rows[i].trim().split(","); int l=Integer.parseInt(data[0]); int w=Integer.parseInt(data[1]); int h=Integer.parseInt(data[2]); Box box = new Box(l,w,h); hashmap.put(box.getvolume(), box); treemap.put(box.getvolume(), box); } catch (IllegalBoxException e) { System.out.println(e.getMessage()); } i++; } BoxGUI boxGUI = new BoxGUI(hashmap, treemap); boxGUI.show(); } catch (IOException e) { System.out.println(e.getMessage()); } } static String readFile(String path, Charset encoding) throws IOException { byte[] encoded = Files.readAllBytes(Paths.get(path)); return new String(encoded, encoding); } }